Ensuring Atomic Updates in Entity Framework: A Deep Dive
Discover how to achieve `atomic updates` in Entity Framework with clear examples and best practices that prevent data duplication in history records.
---
This video is based on the question https://stackoverflow.com/q/73098142/ asked by the user 'dafie' ( https://stackoverflow.com/u/9901683/ ) and on the answer https://stackoverflow.com/a/73104301/ provided by the user 'Steve Py' ( https://stackoverflow.com/u/423497/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Atomic updates in Entity Framework
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Ensuring Atomic Updates in Entity Framework
Entity Framework (EF) is a powerful object-relational mapping (ORM) framework that enables developers to interact with databases using .NET objects. However, when it comes to ensuring atomic updates, developers might encounter some challenges. This post will guide you through the process of creating atomic updates in EF and help you prevent issues like duplicate records in your application.
The Problem
You want to update a record in the history of your database in atomic fashion. This means that you want to ensure that when you increment a value, no two increments can overlap and create duplicate entries for the same value in the history. For example, if you have a value of 5, you wouldn’t want to see multiple records of 5 in the history after several increments. Unfortunately, the initial implementation may not guarantee this.
In your case, even though you thought the Increment method would prevent duplicates using this condition: if (entity.Histories.Last().Value != newValue), you found multiple records with the same value in SQL.
The Solution
To achieve atomic updates in Entity Framework, here are some key recommendations divided into easy-to-follow sections.
1. Understanding Entity Framework and Projections
Entity Framework is designed to handle data queries efficiently. Instead of loading entire entities into memory when you only need one piece of data (like the last history record's value), you can use a more efficient projection approach.
Original Method:
[[See Video to Reveal this Text or Code Snippet]]
Optimized Method:
[[See Video to Reveal this Text or Code Snippet]]
By switching to projections, we ensure that we only fetch what we need, which can lead to performance improvements.
2. Incrementing History Records
When it comes to the incrementing logic, it’s essential to make sure that you check and add the history record instead of relying solely on the Last() value. Here’s an improved and safer implementation for the Increment method:
[[See Video to Reveal this Text or Code Snippet]]
3. Addressing Potential Duplications
With the above method, it still doesn’t eliminate the risk of duplication if simultaneous calls to the Increment method occur. If two threads try to increment the same value at the same time, for example, there is nothing preventing them from passing the if check.
To further enhance consistency and avoid duplicates:
Consider implementing database-level constraints that ensure uniqueness for certain fields.
Use transactions when performing operations that require multiple steps.
Employ optimistic concurrency control by using timestamps or row versions.
Conclusion
By following the strategies outlined above, you can significantly reduce the chances of encountering duplicates in your history records and ensure that your increment operations are atomic.
Entity Framework offers a robust foundation for building data-driven applications, but it requires careful implementation of methods to avoid common pitfalls, particularly around concurrent updates.
Final Thoughts
Ensure that you regularly review and test your database queries for performance and correctness. Over time, as your application scales, the efficiency of these queries will play a significant role in the overall user experience.
Take your Entity Framework skills to the next level by consistently refining your approach to data handling and concurrency management. Happy coding!
Видео Ensuring Atomic Updates in Entity Framework: A Deep Dive канала vlogize
Atomic updates in Entity Framework, c#, entity framework, entity framework core
---
This video is based on the question https://stackoverflow.com/q/73098142/ asked by the user 'dafie' ( https://stackoverflow.com/u/9901683/ ) and on the answer https://stackoverflow.com/a/73104301/ provided by the user 'Steve Py' ( https://stackoverflow.com/u/423497/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Atomic updates in Entity Framework
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Ensuring Atomic Updates in Entity Framework
Entity Framework (EF) is a powerful object-relational mapping (ORM) framework that enables developers to interact with databases using .NET objects. However, when it comes to ensuring atomic updates, developers might encounter some challenges. This post will guide you through the process of creating atomic updates in EF and help you prevent issues like duplicate records in your application.
The Problem
You want to update a record in the history of your database in atomic fashion. This means that you want to ensure that when you increment a value, no two increments can overlap and create duplicate entries for the same value in the history. For example, if you have a value of 5, you wouldn’t want to see multiple records of 5 in the history after several increments. Unfortunately, the initial implementation may not guarantee this.
In your case, even though you thought the Increment method would prevent duplicates using this condition: if (entity.Histories.Last().Value != newValue), you found multiple records with the same value in SQL.
The Solution
To achieve atomic updates in Entity Framework, here are some key recommendations divided into easy-to-follow sections.
1. Understanding Entity Framework and Projections
Entity Framework is designed to handle data queries efficiently. Instead of loading entire entities into memory when you only need one piece of data (like the last history record's value), you can use a more efficient projection approach.
Original Method:
[[See Video to Reveal this Text or Code Snippet]]
Optimized Method:
[[See Video to Reveal this Text or Code Snippet]]
By switching to projections, we ensure that we only fetch what we need, which can lead to performance improvements.
2. Incrementing History Records
When it comes to the incrementing logic, it’s essential to make sure that you check and add the history record instead of relying solely on the Last() value. Here’s an improved and safer implementation for the Increment method:
[[See Video to Reveal this Text or Code Snippet]]
3. Addressing Potential Duplications
With the above method, it still doesn’t eliminate the risk of duplication if simultaneous calls to the Increment method occur. If two threads try to increment the same value at the same time, for example, there is nothing preventing them from passing the if check.
To further enhance consistency and avoid duplicates:
Consider implementing database-level constraints that ensure uniqueness for certain fields.
Use transactions when performing operations that require multiple steps.
Employ optimistic concurrency control by using timestamps or row versions.
Conclusion
By following the strategies outlined above, you can significantly reduce the chances of encountering duplicates in your history records and ensure that your increment operations are atomic.
Entity Framework offers a robust foundation for building data-driven applications, but it requires careful implementation of methods to avoid common pitfalls, particularly around concurrent updates.
Final Thoughts
Ensure that you regularly review and test your database queries for performance and correctness. Over time, as your application scales, the efficiency of these queries will play a significant role in the overall user experience.
Take your Entity Framework skills to the next level by consistently refining your approach to data handling and concurrency management. Happy coding!
Видео Ensuring Atomic Updates in Entity Framework: A Deep Dive канала vlogize
Atomic updates in Entity Framework, c#, entity framework, entity framework core
Показать
Комментарии отсутствуют
Информация о видео
5 апреля 2025 г. 6:56:11
00:02:13
Другие видео канала
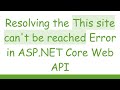
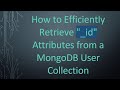
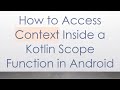

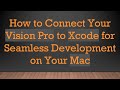
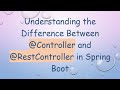

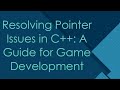

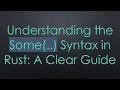
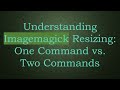

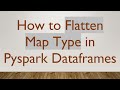
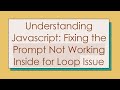
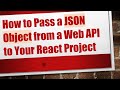
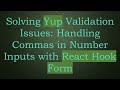


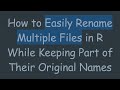
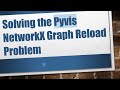
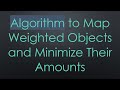