Understanding the Difference Between @ Controller and @ RestController in Spring Boot
Learn why your Spring Controller returns a 404 error while using `@ RestController` works perfectly. Explore common issues and solutions for Spring MVC.
---
This video is based on the question https://stackoverflow.com/q/75199331/ asked by the user 'Seaky Lone' ( https://stackoverflow.com/u/7412111/ ) and on the answer https://stackoverflow.com/a/75199533/ provided by the user 'Matin Kanani' ( https://stackoverflow.com/u/11226918/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: spring controller returns 404 but restcontroller ok
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Difference Between @ Controller and @ RestController in Spring Boot
Spring Boot is a popular framework for building Java web applications, but sometimes navigating its various annotations can lead to unexpected behavior. One common issue developers encounter is when the @ Controller annotation leads to a 404 error, while @ RestController works without a hitch. If you've faced this scenario, you’re not alone! Let’s delve into the reasons behind this behavior and provide clear solutions.
The Problem: Why Are You Getting a 404 Error?
Imagine you have a simple controller set up in Spring Boot that looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Upon accessing the "/api/test/html" endpoint, you’re greeted with a 404 Not Found error. This happens because the type of response expected differs based on whether you use @ Controller or @ RestController.
Explanation of the Controller Annotations
@ Controller Annotation
Purpose: The @ Controller annotation is used to define a traditional Spring MVC controller. It indicates that the class serves the role of a controller in the MVC (Model-View-Controller) pattern.
Response Handling: When a method in a controller annotated with @ Controller is called, it is expected to return a view name, not raw data. Spring looks for a view template associated with the returned string.
@ RestController Annotation
Purpose: The @ RestController annotation is a convenience annotation that combines both @ Controller and @ ResponseBody. It is intended for RESTful web service controllers.
Response Handling: When a method is called, it directly returns the response body, usually in JSON or XML format, with no need to resolve it to a view.
Diagnosis of Your Code
In your case, the method retHtml() returns a String containing HTML tags, which does not correspond to a valid view name. Since you're using @ Controller, Spring attempts to resolve this string to a view, leading to the 404 error:
Incorrect Return Type: Your return value <html></html> does not represent a valid path to a view template.
Response Expectations: Spring is still looking for a view file (like an HTML file) in your project’s resources when using @ Controller.
Solutions: How to Fix the 404 Error
Option 1: Use @ RestController instead of @ Controller
If your goal is to return raw data (like the HTML content), simply change the annotation to @ RestController:
[[See Video to Reveal this Text or Code Snippet]]
Option 2: Change the Implementation for a Traditional MVC Controller
If you want to maintain using @ Controller and return a view, you should adjust your method to return the name of a view template (for example, index), and ensure that the corresponding HTML template exists in your view directory:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the distinction between @ Controller and @ RestController is crucial in Spring Boot development. When you receive a 404 error with a @ Controller, it generally indicates a mismatch in how the return type is being handled. By following the solutions provided, you can effectively resolve the issue and ensure your endpoints function as intended.
Keep experimenting with your controllers, and remember to check the expected return types to avoid similar pitfalls in the future!
Видео Understanding the Difference Between @ Controller and @ RestController in Spring Boot канала vlogize
---
This video is based on the question https://stackoverflow.com/q/75199331/ asked by the user 'Seaky Lone' ( https://stackoverflow.com/u/7412111/ ) and on the answer https://stackoverflow.com/a/75199533/ provided by the user 'Matin Kanani' ( https://stackoverflow.com/u/11226918/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: spring controller returns 404 but restcontroller ok
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Difference Between @ Controller and @ RestController in Spring Boot
Spring Boot is a popular framework for building Java web applications, but sometimes navigating its various annotations can lead to unexpected behavior. One common issue developers encounter is when the @ Controller annotation leads to a 404 error, while @ RestController works without a hitch. If you've faced this scenario, you’re not alone! Let’s delve into the reasons behind this behavior and provide clear solutions.
The Problem: Why Are You Getting a 404 Error?
Imagine you have a simple controller set up in Spring Boot that looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Upon accessing the "/api/test/html" endpoint, you’re greeted with a 404 Not Found error. This happens because the type of response expected differs based on whether you use @ Controller or @ RestController.
Explanation of the Controller Annotations
@ Controller Annotation
Purpose: The @ Controller annotation is used to define a traditional Spring MVC controller. It indicates that the class serves the role of a controller in the MVC (Model-View-Controller) pattern.
Response Handling: When a method in a controller annotated with @ Controller is called, it is expected to return a view name, not raw data. Spring looks for a view template associated with the returned string.
@ RestController Annotation
Purpose: The @ RestController annotation is a convenience annotation that combines both @ Controller and @ ResponseBody. It is intended for RESTful web service controllers.
Response Handling: When a method is called, it directly returns the response body, usually in JSON or XML format, with no need to resolve it to a view.
Diagnosis of Your Code
In your case, the method retHtml() returns a String containing HTML tags, which does not correspond to a valid view name. Since you're using @ Controller, Spring attempts to resolve this string to a view, leading to the 404 error:
Incorrect Return Type: Your return value <html></html> does not represent a valid path to a view template.
Response Expectations: Spring is still looking for a view file (like an HTML file) in your project’s resources when using @ Controller.
Solutions: How to Fix the 404 Error
Option 1: Use @ RestController instead of @ Controller
If your goal is to return raw data (like the HTML content), simply change the annotation to @ RestController:
[[See Video to Reveal this Text or Code Snippet]]
Option 2: Change the Implementation for a Traditional MVC Controller
If you want to maintain using @ Controller and return a view, you should adjust your method to return the name of a view template (for example, index), and ensure that the corresponding HTML template exists in your view directory:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the distinction between @ Controller and @ RestController is crucial in Spring Boot development. When you receive a 404 error with a @ Controller, it generally indicates a mismatch in how the return type is being handled. By following the solutions provided, you can effectively resolve the issue and ensure your endpoints function as intended.
Keep experimenting with your controllers, and remember to check the expected return types to avoid similar pitfalls in the future!
Видео Understanding the Difference Between @ Controller and @ RestController in Spring Boot канала vlogize
Комментарии отсутствуют
Информация о видео
9 апреля 2025 г. 16:04:43
00:01:45
Другие видео канала
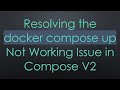


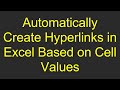
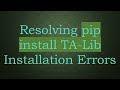
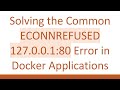

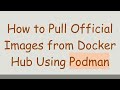

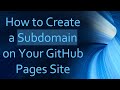

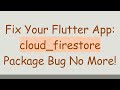
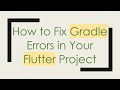
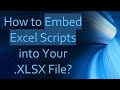
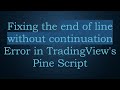
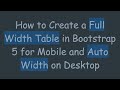
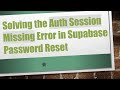



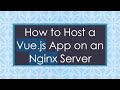