Troubleshooting Unexpected Values in C Code for Credit Card Validation
Discover why your C code might return nonsense values and how to fix it when processing credit card numbers.
---
This video is based on the question https://stackoverflow.com/q/75431128/ asked by the user 'Alan' ( https://stackoverflow.com/u/19463916/ ) and on the answer https://stackoverflow.com/a/75431241/ provided by the user 'Allan Wind' ( https://stackoverflow.com/u/9706/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why is this C code returning unexpected values?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Unexpected Values in C Code for Credit Card Validation
When working with numeric input in programming, especially with identifiers like credit card numbers, it's crucial to understand how data types and operations can affect results. A common scenario arises when developers write a program that processes digits but unexpectedly encounters issues, such as returning negative or nonsensical values. If you've found yourself in a situation with C code returning unexpected values while validating credit card numbers, this post is for you!
The Problem: Unexpected Output from Credit Card Processing
In C, it's easy to hit a snag when manipulating numeric values, particularly when using operations that are sensitive to overflow. Below is a snippet of code that's causing confusion among programmers working on credit card validation.
[[See Video to Reveal this Text or Code Snippet]]
When attempting to multiply and sum every other digit, starting from the second-to-last digit of a credit card number, the outputs may seem completely nonsensical. Often, this results from integer overflow—when the computed value exceeds the maximum limit that can be stored in an integer variable.
The Cause of Nonsensical Values
The core issue in the above code arises from the treatment of the credit_card value during multiplication and division operations. Specifically, when attempting to double a digit from the credit_card number after a series of arithmetic operations, it turns out that:
[[See Video to Reveal this Text or Code Snippet]]
In the first iteration (i=1), we may be calculating an integer value too large for an int type, which can lead to truncation or overflow. This results in negative values, due to how C handles overflow.
Suggested Solution
To tackle and manage this issue, we have several potential fixes. Below is a structured breakdown of the recommended changes:
Use Appropriate Data Types
Include the stdint.h Header:
Utilize fixed-width integer types such as uint64_t (unsigned 64-bit integer) which can safely hold larger values encountered during the credit card processing.
Avoid Float Calculations:
Replace pow() calls (which return a double) in favor of integer-based calculations to prevent floating-point operations that could introduce rounding errors.
Revised Code Implementation
Here's a cleaner and revised implementation of the original code that avoids the pitfalls of integer overflow and maintains clarity and accuracy:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes:
Using uint64_t: Ensures that large numbers are handled correctly without overflow.
Defining Utility Functions: Custom functions for power calculations (my_pow10) ensure integers without reliance on floating-point math, maintaining accuracy.
Conclusion
In conclusion, ensuring the correct data types and avoiding operations that can lead to overflow or rounding errors is critical in C programming, especially when it comes to financial data like credit card numbers. Implementing small yet effective changes can prevent unwanted outputs and lead to a better, more reliable program.
By understanding how to manipulate data types and arithmetic carefully, you can avoid the pitfalls that come with unexpected values in your code. Happy coding!
Видео Troubleshooting Unexpected Values in C Code for Credit Card Validation канала vlogize
---
This video is based on the question https://stackoverflow.com/q/75431128/ asked by the user 'Alan' ( https://stackoverflow.com/u/19463916/ ) and on the answer https://stackoverflow.com/a/75431241/ provided by the user 'Allan Wind' ( https://stackoverflow.com/u/9706/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why is this C code returning unexpected values?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Unexpected Values in C Code for Credit Card Validation
When working with numeric input in programming, especially with identifiers like credit card numbers, it's crucial to understand how data types and operations can affect results. A common scenario arises when developers write a program that processes digits but unexpectedly encounters issues, such as returning negative or nonsensical values. If you've found yourself in a situation with C code returning unexpected values while validating credit card numbers, this post is for you!
The Problem: Unexpected Output from Credit Card Processing
In C, it's easy to hit a snag when manipulating numeric values, particularly when using operations that are sensitive to overflow. Below is a snippet of code that's causing confusion among programmers working on credit card validation.
[[See Video to Reveal this Text or Code Snippet]]
When attempting to multiply and sum every other digit, starting from the second-to-last digit of a credit card number, the outputs may seem completely nonsensical. Often, this results from integer overflow—when the computed value exceeds the maximum limit that can be stored in an integer variable.
The Cause of Nonsensical Values
The core issue in the above code arises from the treatment of the credit_card value during multiplication and division operations. Specifically, when attempting to double a digit from the credit_card number after a series of arithmetic operations, it turns out that:
[[See Video to Reveal this Text or Code Snippet]]
In the first iteration (i=1), we may be calculating an integer value too large for an int type, which can lead to truncation or overflow. This results in negative values, due to how C handles overflow.
Suggested Solution
To tackle and manage this issue, we have several potential fixes. Below is a structured breakdown of the recommended changes:
Use Appropriate Data Types
Include the stdint.h Header:
Utilize fixed-width integer types such as uint64_t (unsigned 64-bit integer) which can safely hold larger values encountered during the credit card processing.
Avoid Float Calculations:
Replace pow() calls (which return a double) in favor of integer-based calculations to prevent floating-point operations that could introduce rounding errors.
Revised Code Implementation
Here's a cleaner and revised implementation of the original code that avoids the pitfalls of integer overflow and maintains clarity and accuracy:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes:
Using uint64_t: Ensures that large numbers are handled correctly without overflow.
Defining Utility Functions: Custom functions for power calculations (my_pow10) ensure integers without reliance on floating-point math, maintaining accuracy.
Conclusion
In conclusion, ensuring the correct data types and avoiding operations that can lead to overflow or rounding errors is critical in C programming, especially when it comes to financial data like credit card numbers. Implementing small yet effective changes can prevent unwanted outputs and lead to a better, more reliable program.
By understanding how to manipulate data types and arithmetic carefully, you can avoid the pitfalls that come with unexpected values in your code. Happy coding!
Видео Troubleshooting Unexpected Values in C Code for Credit Card Validation канала vlogize
Комментарии отсутствуют
Информация о видео
9 апреля 2025 г. 16:17:07
00:02:20
Другие видео канала
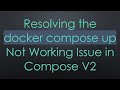


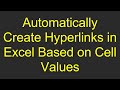
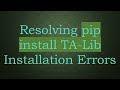
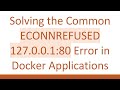


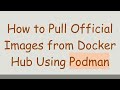

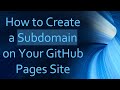

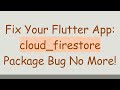
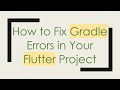
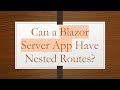
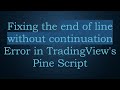
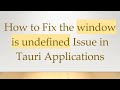
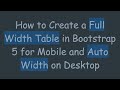
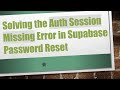

