Mastering Error Handling: Overcoming Challenges in Excel VBA Code
Discover effective strategies for handling errors in Excel VBA, ensuring your code runs smoothly and efficiently, even when unexpected issues arise.
---
This video is based on the question https://stackoverflow.com/q/72959286/ asked by the user 'Cameron Critchlow' ( https://stackoverflow.com/u/16826729/ ) and on the answer https://stackoverflow.com/a/72973235/ provided by the user 'Cameron Critchlow' ( https://stackoverflow.com/u/16826729/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error Handling: Error and string manipulation even after error handling
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Error Handling: Overcoming Challenges in Excel VBA Code
In the realm of programming, particularly in Excel VBA, error handling can be one of the trickiest aspects to manage. Whether you're dealing with unexpected disruptions or simply trying to manipulate strings effectively, implementing a solid error handling strategy is crucial. In this guide, we explore a common error handling issue that many users face and provide a clear, structured solution to resolve it effectively.
Understanding the Problem
Imagine you are working on a VBA script designed to pull data from a source, but you run into intermittent errors. Your code is set to retry operations, but occasionally, it fails to handle errors gracefully. Here’s a breakdown of the situation faced by one user:
Script Functionality: The script is designed to retrieve string data effectively.
Error Handling: The approach taken seems to work most of the time but occasionally results in failure.
Challenge: Specific errors occur, particularly within string manipulation, which violate the expected behavioral pattern.
The issue lies in the way error handling is structured in the VBA code, leading to missed opportunities for retrying operations effectively.
Analyzing the Original Code Structure
The original error handling structure utilized inline error handling that did not effectively provide the necessary control over the execution flow when an error occurred. Below is a simplified representation of how error handling was initially arranged:
[[See Video to Reveal this Text or Code Snippet]]
Here, if an error occurred during one of the string manipulations, the code didn't consistently return to a point that allowed for a retry or suitable resolution.
The Solution: Centralized Error Handling
To provide a clearer and more effective error handling mechanism, the solution involves reorganizing the error handling code. Here’s how you can do it:
Step-by-Step Rewrite
Establish a Structured Error Handling Section: Move error handling to a dedicated portion of your code. This not only increases clarity but also allows for better maintenance.
Implement the Resume Statement: Using the Resume (label) statement allows execution to branch back to your retry logic after handling an error, giving your program a second chance to execute.
Set Limit for Retries: Ensure there's a maximum retry limit to prevent indefinite loops, enhancing the robustness of your code.
Here's a refined version of the code with the updated error handling:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Separate Error Handling: By relocating error handling out of the main logic, your code becomes cleaner and easier to manage.
Use of Resume: This crucial addition allows your program to return to a specific point, enabling the retry of failed code segments.
Clear Error Messages: Providing informative error messages helps users understand what went wrong and need to be addressed.
Conclusion
Effective error handling in Excel VBA is vital for smooth operations. By centralizing your error handling sections, implementing retry logic effectively, and maintaining clarity in your coding practices, you can tackle unexpected issues with confidence. Remember to add thorough testing periods for your revised scripts to ensure they handle errors gracefully in a real-world scenario. Happy coding!
Видео Mastering Error Handling: Overcoming Challenges in Excel VBA Code канала vlogize
Error Handling: Error and string manipulation even after error handling, excel, vba, error handling
---
This video is based on the question https://stackoverflow.com/q/72959286/ asked by the user 'Cameron Critchlow' ( https://stackoverflow.com/u/16826729/ ) and on the answer https://stackoverflow.com/a/72973235/ provided by the user 'Cameron Critchlow' ( https://stackoverflow.com/u/16826729/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error Handling: Error and string manipulation even after error handling
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Error Handling: Overcoming Challenges in Excel VBA Code
In the realm of programming, particularly in Excel VBA, error handling can be one of the trickiest aspects to manage. Whether you're dealing with unexpected disruptions or simply trying to manipulate strings effectively, implementing a solid error handling strategy is crucial. In this guide, we explore a common error handling issue that many users face and provide a clear, structured solution to resolve it effectively.
Understanding the Problem
Imagine you are working on a VBA script designed to pull data from a source, but you run into intermittent errors. Your code is set to retry operations, but occasionally, it fails to handle errors gracefully. Here’s a breakdown of the situation faced by one user:
Script Functionality: The script is designed to retrieve string data effectively.
Error Handling: The approach taken seems to work most of the time but occasionally results in failure.
Challenge: Specific errors occur, particularly within string manipulation, which violate the expected behavioral pattern.
The issue lies in the way error handling is structured in the VBA code, leading to missed opportunities for retrying operations effectively.
Analyzing the Original Code Structure
The original error handling structure utilized inline error handling that did not effectively provide the necessary control over the execution flow when an error occurred. Below is a simplified representation of how error handling was initially arranged:
[[See Video to Reveal this Text or Code Snippet]]
Here, if an error occurred during one of the string manipulations, the code didn't consistently return to a point that allowed for a retry or suitable resolution.
The Solution: Centralized Error Handling
To provide a clearer and more effective error handling mechanism, the solution involves reorganizing the error handling code. Here’s how you can do it:
Step-by-Step Rewrite
Establish a Structured Error Handling Section: Move error handling to a dedicated portion of your code. This not only increases clarity but also allows for better maintenance.
Implement the Resume Statement: Using the Resume (label) statement allows execution to branch back to your retry logic after handling an error, giving your program a second chance to execute.
Set Limit for Retries: Ensure there's a maximum retry limit to prevent indefinite loops, enhancing the robustness of your code.
Here's a refined version of the code with the updated error handling:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Separate Error Handling: By relocating error handling out of the main logic, your code becomes cleaner and easier to manage.
Use of Resume: This crucial addition allows your program to return to a specific point, enabling the retry of failed code segments.
Clear Error Messages: Providing informative error messages helps users understand what went wrong and need to be addressed.
Conclusion
Effective error handling in Excel VBA is vital for smooth operations. By centralizing your error handling sections, implementing retry logic effectively, and maintaining clarity in your coding practices, you can tackle unexpected issues with confidence. Remember to add thorough testing periods for your revised scripts to ensure they handle errors gracefully in a real-world scenario. Happy coding!
Видео Mastering Error Handling: Overcoming Challenges in Excel VBA Code канала vlogize
Error Handling: Error and string manipulation even after error handling, excel, vba, error handling
Показать
Комментарии отсутствуют
Информация о видео
6 апреля 2025 г. 18:56:10
00:02:00
Другие видео канала
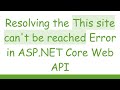

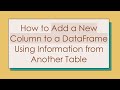

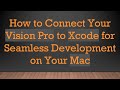
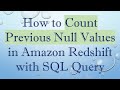
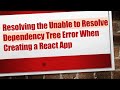
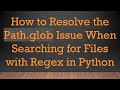
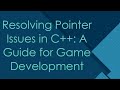
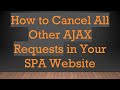
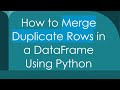
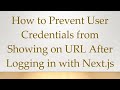

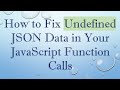

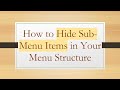
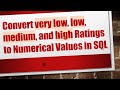
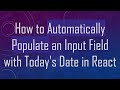
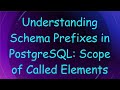

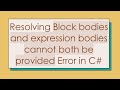