How to Calculate the Depth of Binary Tree | Find Maximum Depth of Binary Tree
How to calculate the depth of binary tree? Find maximum depth of binary tree. Or find maximum depth of a binary tree in javascript. Write a function that finds the maximum depth of a binary tree in JavaScript. A binary tree is a tree data structure in which each node can have at most two children, which are referred to as the left child and the right child.
A binary tree is a hierarchical data structure that is made up of nodes. Each node has a value and two children, which are referred to as the left child and the right child. The root node is the topmost node in the tree and it has no parent. If a node does not have any children, it is referred to as a leaf node.
Binary trees are often used for searching and sorting algorithms and are particularly useful for implementing data structures like heaps and search trees. The structure of a binary tree can be used to determine the order in which nodes are processed and searched, making it an efficient way to represent and process hierarchical data.
Define a class 'TreeNode' with a constructor function that takes a value as input and sets it to the val property of the node. Set the left and right properties of the node to null, indicating that the node has no children yet. Define a function 'maxDepth' that takes a node, called 'root', as its input. This function will return the maximum depth of the binary tree.
In the maxDepth function, use an if statement to check if the root is null. If it is, return 0. This indicates the end of a branch and the return value will be used to determine the maximum depth of the binary tree.
If the root is not null, the function calls itself twice, once with root.left as the argument and once with root.right as the argument. These calls will return the maximum depth of the left and right subtrees, respectively. The function then takes the maximum of the two values and adds 1 to it to count the current node. This result is returned as the maximum depth of the binary tree.
Construct a binary tree by creating a new TreeNode with some value, and assign it to the root variable. Create new TreeNode objects for the left and right children of root and assign to root.left and root.right, respectively. Create two more TreeNode objects for the children of root.right and assign to root.right.left and root.right.right. Call the 'maxDepth' function with root as the argument,
and assign the result to the depth variable. You will get the value of depth as the result.
So this is how you can find maximum depth of a binary tree in JavaScript.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
https://youtube.com/playlist?list=PL1w28LzkbaQHF_HmdZUD0h3lz2p4PzO0A
Queries answered in this video tutorial:
- Find max depth of tree
- How to calculate the depth of binary tree
- Find depth of binary tree
- Find Maximum Depth of a Binary Tree
- Find Maximum Depth of Binary Tree
- Maximum Depth of a Binary Tree
Our tutorials help you to improve your career growth, perform better in your job and make money online as a freelancer. Learn the skills to build and design professional websites, and create dynamic and interactive web applications using JavaScript, or WordPress. Our tutorials are tailored to help beginners and professionals alike. Whether you're just starting in the field or you're looking to expand your knowledge, we've got something for you. Join us on this journey to becoming a skilled web developer. Subscribe to our channel and let's get started!
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
⚡Channel: https://www.youtube.com/@webstylepress
⚡Website: https://www.webstylepress.com
⚡FaceBook: https://www.facebook.com/webstylepress
⚡Twitter: https://twitter.com/webstylepress
⚡GitHub: https://github.com/webstylepress
#js #javascript #challenge #codingchallenge #javascriptinterviewquestions #javascripttutorial #leetcode #coding #programming #computerscience #algorithm #WebStylePress #WebDevelopment #binaryTree
Видео How to Calculate the Depth of Binary Tree | Find Maximum Depth of Binary Tree канала WebStylePress
find max depth of tree, how to calculate the depth of binary tree, find depth of binary tree, Find Maximum Depth of a Binary Tree, Find Maximum Depth of Binary Tree, Maximum Depth of a Binary Tree, Maximum Depth of Binary Tree, find minimum depth of a binary tree, Maximum Depth of Binary Tree in JavaScript, Maximum Depth of a Binary Tree in JavaScript, find max depth of binary tree, Find Maximum Depth of a Binary Tree in JavaScript, js, javascript, algorithm, binary tree
A binary tree is a hierarchical data structure that is made up of nodes. Each node has a value and two children, which are referred to as the left child and the right child. The root node is the topmost node in the tree and it has no parent. If a node does not have any children, it is referred to as a leaf node.
Binary trees are often used for searching and sorting algorithms and are particularly useful for implementing data structures like heaps and search trees. The structure of a binary tree can be used to determine the order in which nodes are processed and searched, making it an efficient way to represent and process hierarchical data.
Define a class 'TreeNode' with a constructor function that takes a value as input and sets it to the val property of the node. Set the left and right properties of the node to null, indicating that the node has no children yet. Define a function 'maxDepth' that takes a node, called 'root', as its input. This function will return the maximum depth of the binary tree.
In the maxDepth function, use an if statement to check if the root is null. If it is, return 0. This indicates the end of a branch and the return value will be used to determine the maximum depth of the binary tree.
If the root is not null, the function calls itself twice, once with root.left as the argument and once with root.right as the argument. These calls will return the maximum depth of the left and right subtrees, respectively. The function then takes the maximum of the two values and adds 1 to it to count the current node. This result is returned as the maximum depth of the binary tree.
Construct a binary tree by creating a new TreeNode with some value, and assign it to the root variable. Create new TreeNode objects for the left and right children of root and assign to root.left and root.right, respectively. Create two more TreeNode objects for the children of root.right and assign to root.right.left and root.right.right. Call the 'maxDepth' function with root as the argument,
and assign the result to the depth variable. You will get the value of depth as the result.
So this is how you can find maximum depth of a binary tree in JavaScript.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
https://youtube.com/playlist?list=PL1w28LzkbaQHF_HmdZUD0h3lz2p4PzO0A
Queries answered in this video tutorial:
- Find max depth of tree
- How to calculate the depth of binary tree
- Find depth of binary tree
- Find Maximum Depth of a Binary Tree
- Find Maximum Depth of Binary Tree
- Maximum Depth of a Binary Tree
Our tutorials help you to improve your career growth, perform better in your job and make money online as a freelancer. Learn the skills to build and design professional websites, and create dynamic and interactive web applications using JavaScript, or WordPress. Our tutorials are tailored to help beginners and professionals alike. Whether you're just starting in the field or you're looking to expand your knowledge, we've got something for you. Join us on this journey to becoming a skilled web developer. Subscribe to our channel and let's get started!
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
⚡Channel: https://www.youtube.com/@webstylepress
⚡Website: https://www.webstylepress.com
⚡FaceBook: https://www.facebook.com/webstylepress
⚡Twitter: https://twitter.com/webstylepress
⚡GitHub: https://github.com/webstylepress
#js #javascript #challenge #codingchallenge #javascriptinterviewquestions #javascripttutorial #leetcode #coding #programming #computerscience #algorithm #WebStylePress #WebDevelopment #binaryTree
Видео How to Calculate the Depth of Binary Tree | Find Maximum Depth of Binary Tree канала WebStylePress
find max depth of tree, how to calculate the depth of binary tree, find depth of binary tree, Find Maximum Depth of a Binary Tree, Find Maximum Depth of Binary Tree, Maximum Depth of a Binary Tree, Maximum Depth of Binary Tree, find minimum depth of a binary tree, Maximum Depth of Binary Tree in JavaScript, Maximum Depth of a Binary Tree in JavaScript, find max depth of binary tree, Find Maximum Depth of a Binary Tree in JavaScript, js, javascript, algorithm, binary tree
Показать
Комментарии отсутствуют
Информация о видео
8 февраля 2023 г. 20:00:30
00:04:06
Другие видео канала
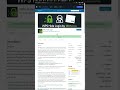
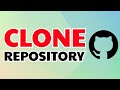
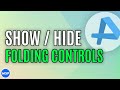
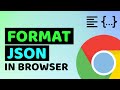

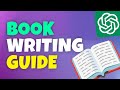
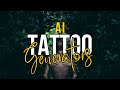
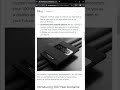


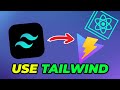

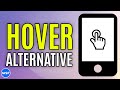
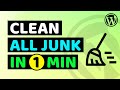
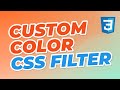

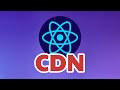
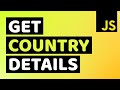
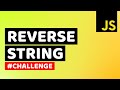
