How to Get the Largest Number in an Array | Find the Largest Number from Array
How to get the largest number in an array or how to find the largest number from array. How to find largest number in array in JavaScript? It is a common javascript interview question or coding challenge.
Let's define a JavaScript function called 'findLargestNumber' that takes in an array items as an argument. Initialize a variable named as 'largest' and assign it the value of the first element of the array items. This is done to ensure that there is an initial value for largest before the for loop starts.
It's final value will be returned as the result. Start a for loop that starts at index 1 of the array (the second element) first element in array has index 0, second element has index 1. Then iterate until the end of the array. The loop variable i is used to access the current element of the array in each iteration. Inside the for loop, there is an if statement that compares the value of the current element items[i] with the current value of largest. If the current element is greater than largest, the value of largest is updated to the current element items[i]. When the for loop completes, the final value of largest will be the largest number in the array. The function should then return the final value. Call the function findLargestNumber(myArray) and pass the array as an argument. It will return the largest number in the array.
There is another short way of doing it.
Define a JavaScript function called 'findLargest' that takes in an array as an argument. Inside function, use the Math.max method. Math.max method is used to find the highest value in a set of numbers. The Math.max method takes in any number of arguments, and returns the highest value among them. The spread operator (...), allows an iterable such as an array to be expanded in places
where zero or more arguments are expected. So in this case, it spreads out all the values of the array, making them individual arguments for the Math.max method. So call the function and pass the array as an argument. It will return the largest number in the array. The function then returns the highest number in the array. In this case the function will return 100 as it is the largest number in the array. This implementation is more concise and easy to understand than the previous one and it is also more efficient.
Both the implementations are correct but the first one will work with older browsers as well because it doesn't require spread operator.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
https://youtube.com/playlist?list=PL1w28LzkbaQHF_HmdZUD0h3lz2p4PzO0A
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Our tutorials help you to improve your career growth, perform better in your job and make money online as a freelancer. Learn the skills to build and design professional websites, and create dynamic and interactive web applications using JavaScript, or WordPress. Our tutorials are tailored to help beginners and professionals alike. Whether you're just starting in the field or you're looking to expand your knowledge, we've got something for you. Join us on this journey to becoming a skilled web developer. Subscribe to our channel and let's get started!
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
⚡Channel: https://www.youtube.com/@webstylepress
⚡Website: https://www.webstylepress.com
⚡FaceBook: https://www.facebook.com/webstylepress
⚡Twitter: https://twitter.com/webstylepress
⚡GitHub: https://github.com/webstylepress
#js #javascript #challenge #WebStylePress #WebDevelopment #javascriptinterviewquestions #javascripttutorial #leetcode #coding #programming #computerscience
Видео How to Get the Largest Number in an Array | Find the Largest Number from Array канала WebStylePress
how to find largest no in array, how to get the largest number in an array, find the largest number from array, how to get the largest number in an array in javascript, Find Largest Number in JavaScript Array, how to find largest number in array js, How to Find Largest Number in Array, largest number from array, How to Find Largest Number in Array in JavaScript, find largest number in array javascript, largest number in array javascript, Find Largest Number in Array, js, javascript
Let's define a JavaScript function called 'findLargestNumber' that takes in an array items as an argument. Initialize a variable named as 'largest' and assign it the value of the first element of the array items. This is done to ensure that there is an initial value for largest before the for loop starts.
It's final value will be returned as the result. Start a for loop that starts at index 1 of the array (the second element) first element in array has index 0, second element has index 1. Then iterate until the end of the array. The loop variable i is used to access the current element of the array in each iteration. Inside the for loop, there is an if statement that compares the value of the current element items[i] with the current value of largest. If the current element is greater than largest, the value of largest is updated to the current element items[i]. When the for loop completes, the final value of largest will be the largest number in the array. The function should then return the final value. Call the function findLargestNumber(myArray) and pass the array as an argument. It will return the largest number in the array.
There is another short way of doing it.
Define a JavaScript function called 'findLargest' that takes in an array as an argument. Inside function, use the Math.max method. Math.max method is used to find the highest value in a set of numbers. The Math.max method takes in any number of arguments, and returns the highest value among them. The spread operator (...), allows an iterable such as an array to be expanded in places
where zero or more arguments are expected. So in this case, it spreads out all the values of the array, making them individual arguments for the Math.max method. So call the function and pass the array as an argument. It will return the largest number in the array. The function then returns the highest number in the array. In this case the function will return 100 as it is the largest number in the array. This implementation is more concise and easy to understand than the previous one and it is also more efficient.
Both the implementations are correct but the first one will work with older browsers as well because it doesn't require spread operator.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
https://youtube.com/playlist?list=PL1w28LzkbaQHF_HmdZUD0h3lz2p4PzO0A
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Our tutorials help you to improve your career growth, perform better in your job and make money online as a freelancer. Learn the skills to build and design professional websites, and create dynamic and interactive web applications using JavaScript, or WordPress. Our tutorials are tailored to help beginners and professionals alike. Whether you're just starting in the field or you're looking to expand your knowledge, we've got something for you. Join us on this journey to becoming a skilled web developer. Subscribe to our channel and let's get started!
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
⚡Channel: https://www.youtube.com/@webstylepress
⚡Website: https://www.webstylepress.com
⚡FaceBook: https://www.facebook.com/webstylepress
⚡Twitter: https://twitter.com/webstylepress
⚡GitHub: https://github.com/webstylepress
#js #javascript #challenge #WebStylePress #WebDevelopment #javascriptinterviewquestions #javascripttutorial #leetcode #coding #programming #computerscience
Видео How to Get the Largest Number in an Array | Find the Largest Number from Array канала WebStylePress
how to find largest no in array, how to get the largest number in an array, find the largest number from array, how to get the largest number in an array in javascript, Find Largest Number in JavaScript Array, how to find largest number in array js, How to Find Largest Number in Array, largest number from array, How to Find Largest Number in Array in JavaScript, find largest number in array javascript, largest number in array javascript, Find Largest Number in Array, js, javascript
Показать
Комментарии отсутствуют
Информация о видео
26 января 2023 г. 18:00:09
00:03:53
Другие видео канала
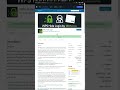
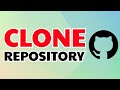
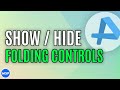
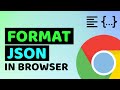

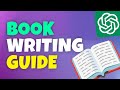

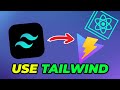

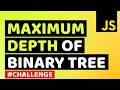
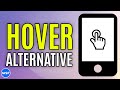
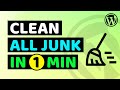
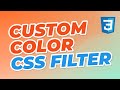

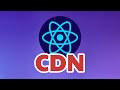
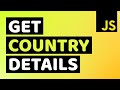
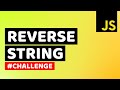


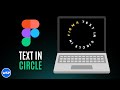