Troubleshooting Python: Why Your if Statement is Only Running the Else Condition
Discover why your Python `if` statements might not be working as expected and learn how to fix it with practical examples.
---
This video is based on the question https://stackoverflow.com/q/73073830/ asked by the user 'XboxOneSogie720' ( https://stackoverflow.com/u/19570262/ ) and on the answer https://stackoverflow.com/a/73073966/ provided by the user 'Yuri Ginsburg' ( https://stackoverflow.com/u/2397684/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: If statements only runs the else condition even though the if condition is true
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Python: Why Your if Statement is Only Running the Else Condition
If you've been programming in Python, you may have encountered an issue where your if statement always seems to execute the else condition, even when it appears that the condition should evaluate to true. This can be particularly frustrating if you're working on an important project, like a push-up log that tracks your workouts. Let’s explore the common pitfalls that lead to this problem and provide a straightforward solution.
The Scenario: Push-Up Log Application
Imagine you're creating a push-up log application that checks for the date in a text file. If the date already exists in the file, it should append your push-up counts to that date. However, your initial attempt returns False even when the date clearly exists in the file. Here’s the code snippet you’re working with:
[[See Video to Reveal this Text or Code Snippet]]
The Unexpected Output
The output of your code is False, even if you have something like this in your text file:
[[See Video to Reveal this Text or Code Snippet]]
At first glance, this is perplexing. You may wonder if your comparisons are off. Let's delve deeper into what’s actually happening behind the scenes.
What’s Going Wrong?
Explanation of File Modes
When you open a file in append mode (a+), Python positions the file pointer at the end of the file. This means that when you call Counter.read(), it starts reading from the current position, which is at the end, resulting in an empty string. Therefore, AllLogs ends up being empty, and your if condition cannot possibly find the current date in it.
Visual Illustration
File Pointer Positioning:
Opened in a+ mode
File pointer at the end of the file (adds content at end, but reads nothing)
The Solution: Using the seek Method
To fix this issue, you need to adjust the position of the file pointer back to the beginning of the file before you read its contents. This is done using the seek(0) method, which resets the position, allowing you to read all the content from the start of the file.
Updated Code
Here’s the corrected version of your code:
[[See Video to Reveal this Text or Code Snippet]]
How It Works
Open the File: The file is opened in append mode; we still need to allow for reading.
Reset the Pointer: Counter.seek(0) moves the pointer back to the start of the file.
Read Content: Now, AllLogs correctly contains all the logged dates.
Condition Check: Your if statement can now accurately evaluate whether today's date is in AllLogs.
Conclusion
Understanding how file modes and pointers work is crucial for effectively using files in Python. By implementing the seek(0) method, you ensure that your if statement checks the correct content in your text file, thus eliminating issues where the else condition runs unexpectedly.
With these adjustments, your push-up log application should work smoothly, allowing you to track your workouts efficiently. Happy coding!
Видео Troubleshooting Python: Why Your if Statement is Only Running the Else Condition канала vlogize
If statements only runs the else condition even though the if condition is true, python, python 3.x
---
This video is based on the question https://stackoverflow.com/q/73073830/ asked by the user 'XboxOneSogie720' ( https://stackoverflow.com/u/19570262/ ) and on the answer https://stackoverflow.com/a/73073966/ provided by the user 'Yuri Ginsburg' ( https://stackoverflow.com/u/2397684/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: If statements only runs the else condition even though the if condition is true
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Python: Why Your if Statement is Only Running the Else Condition
If you've been programming in Python, you may have encountered an issue where your if statement always seems to execute the else condition, even when it appears that the condition should evaluate to true. This can be particularly frustrating if you're working on an important project, like a push-up log that tracks your workouts. Let’s explore the common pitfalls that lead to this problem and provide a straightforward solution.
The Scenario: Push-Up Log Application
Imagine you're creating a push-up log application that checks for the date in a text file. If the date already exists in the file, it should append your push-up counts to that date. However, your initial attempt returns False even when the date clearly exists in the file. Here’s the code snippet you’re working with:
[[See Video to Reveal this Text or Code Snippet]]
The Unexpected Output
The output of your code is False, even if you have something like this in your text file:
[[See Video to Reveal this Text or Code Snippet]]
At first glance, this is perplexing. You may wonder if your comparisons are off. Let's delve deeper into what’s actually happening behind the scenes.
What’s Going Wrong?
Explanation of File Modes
When you open a file in append mode (a+), Python positions the file pointer at the end of the file. This means that when you call Counter.read(), it starts reading from the current position, which is at the end, resulting in an empty string. Therefore, AllLogs ends up being empty, and your if condition cannot possibly find the current date in it.
Visual Illustration
File Pointer Positioning:
Opened in a+ mode
File pointer at the end of the file (adds content at end, but reads nothing)
The Solution: Using the seek Method
To fix this issue, you need to adjust the position of the file pointer back to the beginning of the file before you read its contents. This is done using the seek(0) method, which resets the position, allowing you to read all the content from the start of the file.
Updated Code
Here’s the corrected version of your code:
[[See Video to Reveal this Text or Code Snippet]]
How It Works
Open the File: The file is opened in append mode; we still need to allow for reading.
Reset the Pointer: Counter.seek(0) moves the pointer back to the start of the file.
Read Content: Now, AllLogs correctly contains all the logged dates.
Condition Check: Your if statement can now accurately evaluate whether today's date is in AllLogs.
Conclusion
Understanding how file modes and pointers work is crucial for effectively using files in Python. By implementing the seek(0) method, you ensure that your if statement checks the correct content in your text file, thus eliminating issues where the else condition runs unexpectedly.
With these adjustments, your push-up log application should work smoothly, allowing you to track your workouts efficiently. Happy coding!
Видео Troubleshooting Python: Why Your if Statement is Only Running the Else Condition канала vlogize
If statements only runs the else condition even though the if condition is true, python, python 3.x
Показать
Комментарии отсутствуют
Информация о видео
4 апреля 2025 г. 18:33:04
00:01:51
Другие видео канала

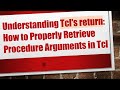
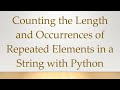
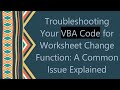
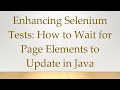

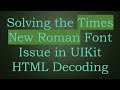
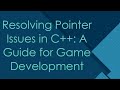
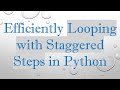

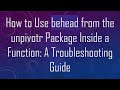
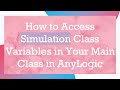
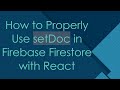
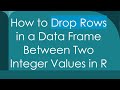
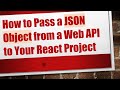

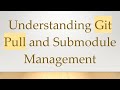

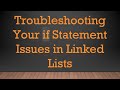
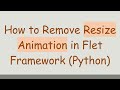
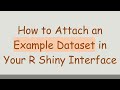