Mastering map and lambda Functions in Python 3
Discover how to effectively use `map` and `lambda` functions in Python 3 for data manipulation, with an emphasis on practical examples and common pitfalls.
---
This video is based on the question https://stackoverflow.com/q/70428600/ asked by the user 'ניר' ( https://stackoverflow.com/u/16776498/ ) and on the answer https://stackoverflow.com/a/70428692/ provided by the user 'chepner' ( https://stackoverflow.com/u/1126841/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: learning to use map and lambda in python 3
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering map and lambda Functions in Python 3: A Practical Guide
In the world of Python programming, two features that often come together to make code cleaner and more efficient are map functions and lambda expressions. However, many beginners stumble when trying to use these tools effectively, particularly in scenarios that involve database updates or list manipulations. This guide aims to clarify how to use map and lambda in Python 3, specifically in the context of manipulating SQLite databases.
Understanding the Problem
You might find yourself in a situation where you want to update several columns in a database table using the same data. You may consider making use of Python's lambda and map functions, which can help streamline your code. However, things may not work as expected. For instance, in a recent script involving SQLite, an attempt to insert a string to all columns resulted in no updates occurring—even without throwing an error. Let's explore why that might be, and how to fix it.
The Code Structure
Let's look at the relevant parts of a Python script that aimed to automate updates in an SQLite table:
[[See Video to Reveal this Text or Code Snippet]]
The script features a class DB with methods for fetching column names and updating their respective values. However, the use of map and lambda functions is not effective because the map output is not being iterated, leading to no updates taking place.
Why the Original Code Fails
The primary issue lies in the misunderstanding of how map operates. The map function does not return a list but an iterator that produces items on demand. Consequently, if you're not iterating over this map instance, the underlying functions will not be executed.
Key Points
map generates items on demand: Without iteration, the subsequent function will not execute.
For side effects, prefer a for loop: Instead of trying to use map for side effects (like updating the database), a simple loop can be much clearer.
A Better Approach Using a For Loop
To solve the issue, you can simply use a for loop instead. Here’s how you can rewrite the part that updates the database:
[[See Video to Reveal this Text or Code Snippet]]
This method iterates through each column and applies the update_to_last method directly, ensuring all desired updates are performed correctly.
When to Use map and lambda Functions
While using map and lambda is not advisable for operations with side effects, there are scenarios where it shines. For example, if you want to create a list based on outputs from a function, using map can be efficient.
Example of Appropriate Use of map
If your update_to_last method produced a returnable value that you wanted to store in a list, you could do something like:
[[See Video to Reveal this Text or Code Snippet]]
However, using a list comprehension is generally clearer and preferred:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, while map and lambda are powerful features of Python, their application should be carefully considered, especially in side-effect cases like database updates. For better readability and functionality, a traditional for loop is often the best choice. By understanding how these features work, you can enhance your coding skills and create more efficient Python scripts.
Happy coding!
Видео Mastering map and lambda Functions in Python 3 канала vlogize
learning to use map and lambda in python 3, python, python 3.x, dictionary, lambda
---
This video is based on the question https://stackoverflow.com/q/70428600/ asked by the user 'ניר' ( https://stackoverflow.com/u/16776498/ ) and on the answer https://stackoverflow.com/a/70428692/ provided by the user 'chepner' ( https://stackoverflow.com/u/1126841/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: learning to use map and lambda in python 3
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering map and lambda Functions in Python 3: A Practical Guide
In the world of Python programming, two features that often come together to make code cleaner and more efficient are map functions and lambda expressions. However, many beginners stumble when trying to use these tools effectively, particularly in scenarios that involve database updates or list manipulations. This guide aims to clarify how to use map and lambda in Python 3, specifically in the context of manipulating SQLite databases.
Understanding the Problem
You might find yourself in a situation where you want to update several columns in a database table using the same data. You may consider making use of Python's lambda and map functions, which can help streamline your code. However, things may not work as expected. For instance, in a recent script involving SQLite, an attempt to insert a string to all columns resulted in no updates occurring—even without throwing an error. Let's explore why that might be, and how to fix it.
The Code Structure
Let's look at the relevant parts of a Python script that aimed to automate updates in an SQLite table:
[[See Video to Reveal this Text or Code Snippet]]
The script features a class DB with methods for fetching column names and updating their respective values. However, the use of map and lambda functions is not effective because the map output is not being iterated, leading to no updates taking place.
Why the Original Code Fails
The primary issue lies in the misunderstanding of how map operates. The map function does not return a list but an iterator that produces items on demand. Consequently, if you're not iterating over this map instance, the underlying functions will not be executed.
Key Points
map generates items on demand: Without iteration, the subsequent function will not execute.
For side effects, prefer a for loop: Instead of trying to use map for side effects (like updating the database), a simple loop can be much clearer.
A Better Approach Using a For Loop
To solve the issue, you can simply use a for loop instead. Here’s how you can rewrite the part that updates the database:
[[See Video to Reveal this Text or Code Snippet]]
This method iterates through each column and applies the update_to_last method directly, ensuring all desired updates are performed correctly.
When to Use map and lambda Functions
While using map and lambda is not advisable for operations with side effects, there are scenarios where it shines. For example, if you want to create a list based on outputs from a function, using map can be efficient.
Example of Appropriate Use of map
If your update_to_last method produced a returnable value that you wanted to store in a list, you could do something like:
[[See Video to Reveal this Text or Code Snippet]]
However, using a list comprehension is generally clearer and preferred:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, while map and lambda are powerful features of Python, their application should be carefully considered, especially in side-effect cases like database updates. For better readability and functionality, a traditional for loop is often the best choice. By understanding how these features work, you can enhance your coding skills and create more efficient Python scripts.
Happy coding!
Видео Mastering map and lambda Functions in Python 3 канала vlogize
learning to use map and lambda in python 3, python, python 3.x, dictionary, lambda
Показать
Комментарии отсутствуют
Информация о видео
30 марта 2025 г. 7:41:10
00:01:48
Другие видео канала

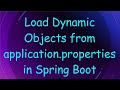

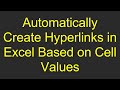
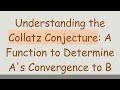


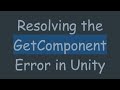

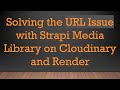
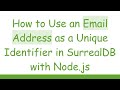
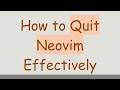
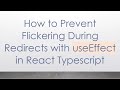
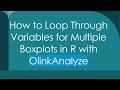

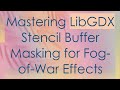

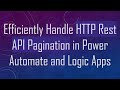
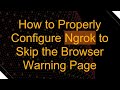
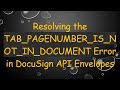
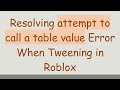