How to Prevent an Infinite Loop in React's useEffect with useSelector
Discover the common pitfall of `infinite loops` in React when using `useEffect` with `useSelector`, and learn the simple solution to fix it!
---
This video is based on the question https://stackoverflow.com/q/73273591/ asked by the user 'irahama' ( https://stackoverflow.com/u/16204040/ ) and on the answer https://stackoverflow.com/a/73273647/ provided by the user 'Mirza Umair' ( https://stackoverflow.com/u/12101795/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Infinite loop react Js ,use useEffect after i put on parameter with useSelector contains data of array
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Infinite Loops in React's useEffect
React is a powerful library that allows developers to build dynamic web applications. However, when using hooks such as useEffect in conjunction with state management tools like Redux, it's common to stumble upon issues such as infinite loops. This guide will provide clarity on this specific problem and guide you on how to resolve it effectively.
The Problem: Infinite Loop with useEffect and useSelector
Imagine you have a situation where you are using the useEffect hook to call an API and dispatch an action that updates your Redux store. The intention is straightforward: fetch data and update the display with new information. However, you notice that the console keeps logging output repeatedly, leading to what seems like an infinite loop.
Here’s a simplified version of your code for context:
[[See Video to Reveal this Text or Code Snippet]]
In this setup, every time itemGroup updates, the useEffect gets triggered, which in turn calls callFunction(). This function dispatches an action to fetch data again, modifying itemGroup, and the cycle continues endlessly. This creates an infinite loop that can hinder your application’s performance and lead to unwanted side effects.
The Solution: Stop the Loop with an Empty Dependency Array
The root cause of the infinite loop is the dependency array you've supplied to the useEffect hook. By including itemGroup as a dependency, you are instructing React to re-run the effect whenever itemGroup changes, thus causing the continual fetching of the data.
Step-by-Step Solution
Remove the Dependency: To break this cycle, you can eliminate itemGroup from the dependency array.
Use an Empty Array: Instead, you can provide an empty array [], meaning that the effect will only run once when the component mounts.
Here’s how you can update your code:
[[See Video to Reveal this Text or Code Snippet]]
This change ensures that callFunction() is called just a single time without triggering further updates based on itemGroup. As you fetch data when the component mounts, subsequent changes to itemGroup won’t keep calling the function repeatedly.
Additional Considerations
Data Updates: If you need the data to update based on user actions or other events, consider placing that logic outside of useEffect and triggering it as needed.
Debugging: Use console.log(itemGroup); effectively to monitor changes, but avoid causing side effects that trigger further updates.
Conclusion
Infinite loops in React can be a perplexing issue, especially when combining hooks and state management. By understanding how dependencies work in useEffect, you can ensure that your application runs smoothly without unnecessary re-renders. Remember, sometimes the best solution is simply passing an empty dependency array to prevent any unintended consequences.
By implementing the tips outlined in this post, you can navigate around the complications of Redux and React gracefully, delivering a seamless experience for both developers and users.
If you have more questions or need further clarification, feel free to reach out!
Видео How to Prevent an Infinite Loop in React's useEffect with useSelector канала vlogize
---
This video is based on the question https://stackoverflow.com/q/73273591/ asked by the user 'irahama' ( https://stackoverflow.com/u/16204040/ ) and on the answer https://stackoverflow.com/a/73273647/ provided by the user 'Mirza Umair' ( https://stackoverflow.com/u/12101795/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Infinite loop react Js ,use useEffect after i put on parameter with useSelector contains data of array
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Infinite Loops in React's useEffect
React is a powerful library that allows developers to build dynamic web applications. However, when using hooks such as useEffect in conjunction with state management tools like Redux, it's common to stumble upon issues such as infinite loops. This guide will provide clarity on this specific problem and guide you on how to resolve it effectively.
The Problem: Infinite Loop with useEffect and useSelector
Imagine you have a situation where you are using the useEffect hook to call an API and dispatch an action that updates your Redux store. The intention is straightforward: fetch data and update the display with new information. However, you notice that the console keeps logging output repeatedly, leading to what seems like an infinite loop.
Here’s a simplified version of your code for context:
[[See Video to Reveal this Text or Code Snippet]]
In this setup, every time itemGroup updates, the useEffect gets triggered, which in turn calls callFunction(). This function dispatches an action to fetch data again, modifying itemGroup, and the cycle continues endlessly. This creates an infinite loop that can hinder your application’s performance and lead to unwanted side effects.
The Solution: Stop the Loop with an Empty Dependency Array
The root cause of the infinite loop is the dependency array you've supplied to the useEffect hook. By including itemGroup as a dependency, you are instructing React to re-run the effect whenever itemGroup changes, thus causing the continual fetching of the data.
Step-by-Step Solution
Remove the Dependency: To break this cycle, you can eliminate itemGroup from the dependency array.
Use an Empty Array: Instead, you can provide an empty array [], meaning that the effect will only run once when the component mounts.
Here’s how you can update your code:
[[See Video to Reveal this Text or Code Snippet]]
This change ensures that callFunction() is called just a single time without triggering further updates based on itemGroup. As you fetch data when the component mounts, subsequent changes to itemGroup won’t keep calling the function repeatedly.
Additional Considerations
Data Updates: If you need the data to update based on user actions or other events, consider placing that logic outside of useEffect and triggering it as needed.
Debugging: Use console.log(itemGroup); effectively to monitor changes, but avoid causing side effects that trigger further updates.
Conclusion
Infinite loops in React can be a perplexing issue, especially when combining hooks and state management. By understanding how dependencies work in useEffect, you can ensure that your application runs smoothly without unnecessary re-renders. Remember, sometimes the best solution is simply passing an empty dependency array to prevent any unintended consequences.
By implementing the tips outlined in this post, you can navigate around the complications of Redux and React gracefully, delivering a seamless experience for both developers and users.
If you have more questions or need further clarification, feel free to reach out!
Видео How to Prevent an Infinite Loop in React's useEffect with useSelector канала vlogize
Комментарии отсутствуют
Информация о видео
31 марта 2025 г. 3:13:01
00:01:31
Другие видео канала


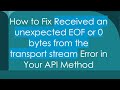
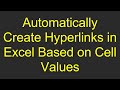


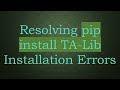
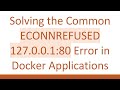

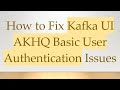
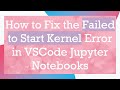
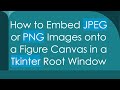

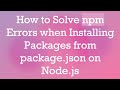
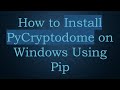
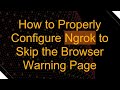
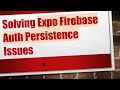
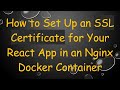
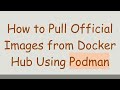

