Ensuring Robust Error Handling in Python Class Constructors
Learn how to implement effective error handling in class constructors to validate input values in Python.
---
This video is based on the question https://stackoverflow.com/q/73450350/ asked by the user 'Kosmylo' ( https://stackoverflow.com/u/12000021/ ) and on the answer https://stackoverflow.com/a/73451066/ provided by the user 'Barmar' ( https://stackoverflow.com/u/1491895/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error handling in the values in a class constructor
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Error Handling in Python Class Constructors: A Guide
When developing applications in Python, ensuring that your classes receive valid input is crucial to maintaining stability and preventing unexpected behavior. One common question arises when you need to validate parameters in a class constructor. Specifically, how can you ensure that values passed to the constructor are both positive and within a specific range? In this post, we will explore an effective approach to error handling in Python class constructors, using a simple example.
The Problem
Consider a class defined as follows:
[[See Video to Reveal this Text or Code Snippet]]
In this example, n and m are two parameters that you want to restrict based on certain conditions:
n should be a positive number.
m must be less than n squared (i.e., n**2) and positive itself.
The Need for Validation
Without proper validation, if someone attempts to create an instance of SimpleClass with invalid values, it may lead to errors later in your code. For example, if m is negative or greater than n**2, it could cause runtime exceptions or logical issues in your application.
The Solution: Implementing Error Handling
To effectively handle errors in your class constructor, the standard approach is to use simple if-statements to check the validity of the input parameters. If any condition is not met, you raise a ValueError with a descriptive message. Let’s explore how this can be implemented:
Step-by-Step Implementation
Here’s how you can modify the class to include error checks:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Implementation
Condition Checks:
The first if statement checks if n is less than or equal to zero. If so, it raises a ValueError with a clear message indicating that n must be positive.
The second if statement checks two conditions for m: it verifies that m is greater than zero and less than n squared. If m does not meet these criteria, another ValueError is raised with a specific message.
Raising Exceptions: Using ValueError is appropriate here as you are indicating that the argument value provided to the function is inappropriate.
Data Assignment: If all checks pass, self.n and self.m are assigned the validated values.
Benefits of This Approach
Clarity: Each error check is distinct and makes it clear what the possible input issues are.
Maintainability: By using if-statements, this code is easy to read and adjust if additional constraints need to be added later.
User-Friendly Feedback: The specific error messages help the user understand what went wrong, making it easier to correct input mistakes.
Conclusion
In summary, handling errors in class constructors is vital for developing robust Python applications. By implementing straightforward if-statements and raising exceptions as needed, you can ensure that your class is only instantiated with valid inputs. Not only does this enhance the reliability of your code, but it also improves the overall user experience by providing clear feedback about input errors.
Now you can confidently implement error handling in your Python classes, ensuring that the values passed to constructors are appropriate and valid!
Видео Ensuring Robust Error Handling in Python Class Constructors канала vlogize
Error handling in the values in a class constructor, python, class, error handling
---
This video is based on the question https://stackoverflow.com/q/73450350/ asked by the user 'Kosmylo' ( https://stackoverflow.com/u/12000021/ ) and on the answer https://stackoverflow.com/a/73451066/ provided by the user 'Barmar' ( https://stackoverflow.com/u/1491895/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error handling in the values in a class constructor
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Error Handling in Python Class Constructors: A Guide
When developing applications in Python, ensuring that your classes receive valid input is crucial to maintaining stability and preventing unexpected behavior. One common question arises when you need to validate parameters in a class constructor. Specifically, how can you ensure that values passed to the constructor are both positive and within a specific range? In this post, we will explore an effective approach to error handling in Python class constructors, using a simple example.
The Problem
Consider a class defined as follows:
[[See Video to Reveal this Text or Code Snippet]]
In this example, n and m are two parameters that you want to restrict based on certain conditions:
n should be a positive number.
m must be less than n squared (i.e., n**2) and positive itself.
The Need for Validation
Without proper validation, if someone attempts to create an instance of SimpleClass with invalid values, it may lead to errors later in your code. For example, if m is negative or greater than n**2, it could cause runtime exceptions or logical issues in your application.
The Solution: Implementing Error Handling
To effectively handle errors in your class constructor, the standard approach is to use simple if-statements to check the validity of the input parameters. If any condition is not met, you raise a ValueError with a descriptive message. Let’s explore how this can be implemented:
Step-by-Step Implementation
Here’s how you can modify the class to include error checks:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Implementation
Condition Checks:
The first if statement checks if n is less than or equal to zero. If so, it raises a ValueError with a clear message indicating that n must be positive.
The second if statement checks two conditions for m: it verifies that m is greater than zero and less than n squared. If m does not meet these criteria, another ValueError is raised with a specific message.
Raising Exceptions: Using ValueError is appropriate here as you are indicating that the argument value provided to the function is inappropriate.
Data Assignment: If all checks pass, self.n and self.m are assigned the validated values.
Benefits of This Approach
Clarity: Each error check is distinct and makes it clear what the possible input issues are.
Maintainability: By using if-statements, this code is easy to read and adjust if additional constraints need to be added later.
User-Friendly Feedback: The specific error messages help the user understand what went wrong, making it easier to correct input mistakes.
Conclusion
In summary, handling errors in class constructors is vital for developing robust Python applications. By implementing straightforward if-statements and raising exceptions as needed, you can ensure that your class is only instantiated with valid inputs. Not only does this enhance the reliability of your code, but it also improves the overall user experience by providing clear feedback about input errors.
Now you can confidently implement error handling in your Python classes, ensuring that the values passed to constructors are appropriate and valid!
Видео Ensuring Robust Error Handling in Python Class Constructors канала vlogize
Error handling in the values in a class constructor, python, class, error handling
Показать
Комментарии отсутствуют
Информация о видео
2 апреля 2025 г. 20:13:54
00:01:41
Другие видео канала
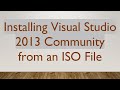
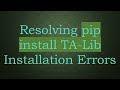
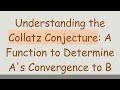


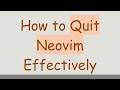
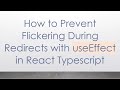

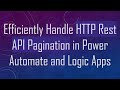
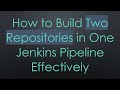

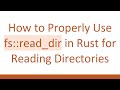

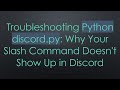
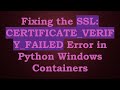
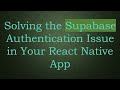

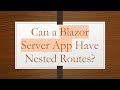
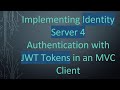
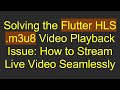
