Efficiently Filtering Parent Records Using Entity Framework Core in .NET 5
Discover how to effectively filter parent records based on specific child rows using LINQ queries with Entity Framework Core in .NET 5. Get practical tips and code examples!
---
This video is based on the question https://stackoverflow.com/q/73109803/ asked by the user 'Dantre' ( https://stackoverflow.com/u/19617189/ ) and on the answer https://stackoverflow.com/a/73110455/ provided by the user 'Svyatoslav Danyliv' ( https://stackoverflow.com/u/10646316/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to efficiently filter parent records based on a specific children row
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Filtering Parent Records Using Entity Framework Core in .NET 5
In modern application development, it's common to work with related data stored in different tables. This relationship often presents challenges in querying data efficiently. In this post, we will explore a specific problem faced when attempting to filter parent records based on recent child rows in a SQL Server database, using Entity Framework Core in .NET 5.
The Problem: Filtering Transactions based on TransactionHistory
In our scenario, we have two related tables: Transaction and TransactionHistory where a single transaction can have multiple history records. Each TransactionHistory record contains a status that determines the state of the transaction.
The problem arises when trying to filter Transaction records according to the most recent status from the TransactionHistory. For instance, we want to fetch transactions that are either in a "ready" state or in a retriable "error" state, allowing for a maximum number of tries. The initial LINQ implementation repeated subqueries that would negatively affect performance and readability.
Current Approach (Inefficient)
The existing LINQ query looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This approach generates a SQL query with multiple subqueries, leading to potential performance issues:
Repetitive subqueries fetching the same TransactionHistory rows.
The generated SQL includes complex joins that could be simplified.
The Solution: A More Efficient Query
To improve performance and streamline the query, we can leverage a LINQ syntax that minimizes redundancy. Here's a more effective way to structure the query using a combination of LINQ’s from syntax and concise filtering.
Efficient LINQ Query
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Improvements
Single Subquery: By using the from clause effectively, we only retrieve the latest TransactionHistory for each transaction once.
Clear Conditions: The where clause is now clear and comprehensible, providing a straightforward filter based on the latest status and error types.
Result Projection: The use of an anonymous type helps in organizing the returned results but if the middle step isn't necessary, you can still return the transaction type directly as needed.
Conclusion
Efficient data retrieval in applications involving relationships is crucial for maintaining performance and manageability. By structuring our LINQ queries correctly, we can avoid redundancy, improve readability, and enhance overall application efficiency.
If you have similar issues or further queries regarding Entity Framework Core and LINQ queries in .NET, feel free to share your thoughts in the comments below!
Видео Efficiently Filtering Parent Records Using Entity Framework Core in .NET 5 канала vlogize
How to efficiently filter parent records based on a specific children row, c#, sql server, linq, entity framework core, .net 5
---
This video is based on the question https://stackoverflow.com/q/73109803/ asked by the user 'Dantre' ( https://stackoverflow.com/u/19617189/ ) and on the answer https://stackoverflow.com/a/73110455/ provided by the user 'Svyatoslav Danyliv' ( https://stackoverflow.com/u/10646316/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to efficiently filter parent records based on a specific children row
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Filtering Parent Records Using Entity Framework Core in .NET 5
In modern application development, it's common to work with related data stored in different tables. This relationship often presents challenges in querying data efficiently. In this post, we will explore a specific problem faced when attempting to filter parent records based on recent child rows in a SQL Server database, using Entity Framework Core in .NET 5.
The Problem: Filtering Transactions based on TransactionHistory
In our scenario, we have two related tables: Transaction and TransactionHistory where a single transaction can have multiple history records. Each TransactionHistory record contains a status that determines the state of the transaction.
The problem arises when trying to filter Transaction records according to the most recent status from the TransactionHistory. For instance, we want to fetch transactions that are either in a "ready" state or in a retriable "error" state, allowing for a maximum number of tries. The initial LINQ implementation repeated subqueries that would negatively affect performance and readability.
Current Approach (Inefficient)
The existing LINQ query looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This approach generates a SQL query with multiple subqueries, leading to potential performance issues:
Repetitive subqueries fetching the same TransactionHistory rows.
The generated SQL includes complex joins that could be simplified.
The Solution: A More Efficient Query
To improve performance and streamline the query, we can leverage a LINQ syntax that minimizes redundancy. Here's a more effective way to structure the query using a combination of LINQ’s from syntax and concise filtering.
Efficient LINQ Query
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Improvements
Single Subquery: By using the from clause effectively, we only retrieve the latest TransactionHistory for each transaction once.
Clear Conditions: The where clause is now clear and comprehensible, providing a straightforward filter based on the latest status and error types.
Result Projection: The use of an anonymous type helps in organizing the returned results but if the middle step isn't necessary, you can still return the transaction type directly as needed.
Conclusion
Efficient data retrieval in applications involving relationships is crucial for maintaining performance and manageability. By structuring our LINQ queries correctly, we can avoid redundancy, improve readability, and enhance overall application efficiency.
If you have similar issues or further queries regarding Entity Framework Core and LINQ queries in .NET, feel free to share your thoughts in the comments below!
Видео Efficiently Filtering Parent Records Using Entity Framework Core in .NET 5 канала vlogize
How to efficiently filter parent records based on a specific children row, c#, sql server, linq, entity framework core, .net 5
Показать
Комментарии отсутствуют
Информация о видео
11 ч. 9 мин. назад
00:01:55
Другие видео канала

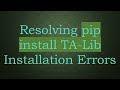
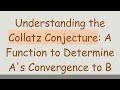


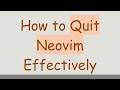
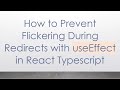

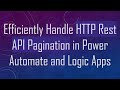
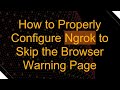


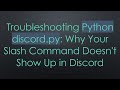
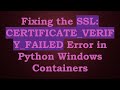
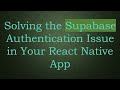

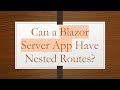
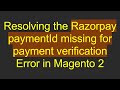
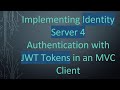

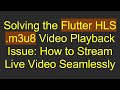