How to Update DataFrame Column Values in Python with Pandas Using a Dictionary
Learn how to efficiently update your DataFrame column values in Python's Pandas based on another column using a dictionary, without losing existing data.
---
This video is based on the question https://stackoverflow.com/q/72515864/ asked by the user 'AmanArora' ( https://stackoverflow.com/u/2781402/ ) and on the answer https://stackoverflow.com/a/72515975/ provided by the user 'Nick' ( https://stackoverflow.com/u/9473764/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Change column values based on another column (don't change if not in dictionary)
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Update DataFrame Column Values in Python with Pandas Using a Dictionary
When working with data in Python, using the Pandas library offers powerful tools to manipulate and process your datasets. A common scenario many data analysts encounter is updating one column of a DataFrame based on the values found in another column.
In this post, we will focus on a particular problem: how to update the "Pack_Size" column based on the "Product_ID" using a provided dictionary without losing the existing values in the "Pack_Size" column.
The Problem
You have a DataFrame that contains a column named "Product_ID" and you wish to update the "Pack_Size" column based on a pre-defined dictionary. Each key in this dictionary corresponds to a "Product_ID" and its associated value is the desired "Pack_Size". The challenge arises when you attempt to map your dictionary to the DataFrame. If a "Product_ID" is not found in your dictionary, Pandas will replace the "Pack_Size" with a NaN value.
For example, your dictionary looks like this:
[[See Video to Reveal this Text or Code Snippet]]
When applying the mapping, you might have used the following code:
[[See Video to Reveal this Text or Code Snippet]]
As a result, any "Product_ID" not present in pack_size_dict would lead to NaN values in the "Pack_Size" column, which is not the desired outcome.
The Solution
To solve this problem, you can use the fillna() function combined with your mapping. This way, you can replace only the NaN values with the existing "Pack_Size" values from the DataFrame. Here’s how to do it correctly:
Step-by-Step Instructions
Map the Dictionary: Use the map() function to create a new column with updated values from your dictionary.
Fill NaN Values: Use fillna() to keep existing values in "Pack_Size" where "Product_ID" is not found in the dictionary.
Implementation
Here's the complete line of code you'd need to use:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
df['Product_ID'].map(pack_size_dict): This line creates a Series where each "Product_ID" is replaced with the corresponding "Pack_Size" from the dictionary. If there is no match, it will result in NaN.
.fillna(df['Pack_Size']): This method checks the newly created Series for any NaN values and replaces them with the original values from the "Pack_Size" column in the DataFrame.
Conclusion
By using this method, you ensure that your DataFrame's "Pack_Size" is updated efficiently without losing any existing data. This approach is versatile and can be applied to many similar problems where conditional updates are needed based on dictionaries.
Feel free to try out this solution in your programs and improve your data handling techniques with Pandas!
Видео How to Update DataFrame Column Values in Python with Pandas Using a Dictionary канала vlogize
---
This video is based on the question https://stackoverflow.com/q/72515864/ asked by the user 'AmanArora' ( https://stackoverflow.com/u/2781402/ ) and on the answer https://stackoverflow.com/a/72515975/ provided by the user 'Nick' ( https://stackoverflow.com/u/9473764/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Change column values based on another column (don't change if not in dictionary)
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Update DataFrame Column Values in Python with Pandas Using a Dictionary
When working with data in Python, using the Pandas library offers powerful tools to manipulate and process your datasets. A common scenario many data analysts encounter is updating one column of a DataFrame based on the values found in another column.
In this post, we will focus on a particular problem: how to update the "Pack_Size" column based on the "Product_ID" using a provided dictionary without losing the existing values in the "Pack_Size" column.
The Problem
You have a DataFrame that contains a column named "Product_ID" and you wish to update the "Pack_Size" column based on a pre-defined dictionary. Each key in this dictionary corresponds to a "Product_ID" and its associated value is the desired "Pack_Size". The challenge arises when you attempt to map your dictionary to the DataFrame. If a "Product_ID" is not found in your dictionary, Pandas will replace the "Pack_Size" with a NaN value.
For example, your dictionary looks like this:
[[See Video to Reveal this Text or Code Snippet]]
When applying the mapping, you might have used the following code:
[[See Video to Reveal this Text or Code Snippet]]
As a result, any "Product_ID" not present in pack_size_dict would lead to NaN values in the "Pack_Size" column, which is not the desired outcome.
The Solution
To solve this problem, you can use the fillna() function combined with your mapping. This way, you can replace only the NaN values with the existing "Pack_Size" values from the DataFrame. Here’s how to do it correctly:
Step-by-Step Instructions
Map the Dictionary: Use the map() function to create a new column with updated values from your dictionary.
Fill NaN Values: Use fillna() to keep existing values in "Pack_Size" where "Product_ID" is not found in the dictionary.
Implementation
Here's the complete line of code you'd need to use:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
df['Product_ID'].map(pack_size_dict): This line creates a Series where each "Product_ID" is replaced with the corresponding "Pack_Size" from the dictionary. If there is no match, it will result in NaN.
.fillna(df['Pack_Size']): This method checks the newly created Series for any NaN values and replaces them with the original values from the "Pack_Size" column in the DataFrame.
Conclusion
By using this method, you ensure that your DataFrame's "Pack_Size" is updated efficiently without losing any existing data. This approach is versatile and can be applied to many similar problems where conditional updates are needed based on dictionaries.
Feel free to try out this solution in your programs and improve your data handling techniques with Pandas!
Видео How to Update DataFrame Column Values in Python with Pandas Using a Dictionary канала vlogize
Комментарии отсутствуют
Информация о видео
18 апреля 2025 г. 0:20:06
00:01:33
Другие видео канала
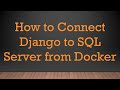
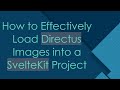


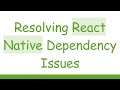

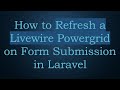
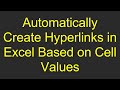



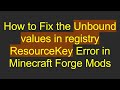

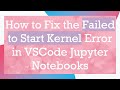
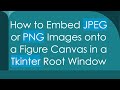

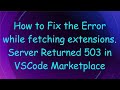
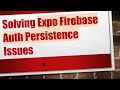
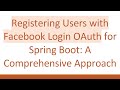

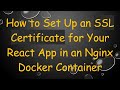