How to Extract Rows Within a datetime Range in Python DataFrames
Learn how to efficiently filter rows in a Python DataFrame that fall within a specific `datetime` range, using logical operators correctly.
---
This video is based on the question https://stackoverflow.com/q/76724701/ asked by the user '1324241421' ( https://stackoverflow.com/u/14560840/ ) and on the answer https://stackoverflow.com/a/76724716/ provided by the user 'not_speshal' ( https://stackoverflow.com/u/9857631/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Extracting rows that satisfy the condition of being within a datetime range
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Extracting Rows That Fall Within a datetime Range in Python
When working with data in Python, especially in the form of DataFrames using libraries like Pandas, you may encounter situations where you need to filter data based on date ranges. For instance, you might have a DataFrame containing columns for operation start and end dates, and you want to extract rows where a particular date falls between these start and end dates. This guide addresses a common issue that can arise during this process and provides a simple solution.
The Problem
Imagine you have a DataFrame called df_new with the following structure:
Start: The starting date for an operation
End: The ending date for an operation
Your task is to check if a specific currentdate (also in datetime format) is within the range of these start and end dates. However, you're experiencing errors when using boolean operators in your attempts to filter the DataFrame. Specifically, you've encountered errors like:
TypeError: Unsupported operand type(s) for &: 'datetime.datetime' and 'datetime.datetime'
ValueError: The truth value of a Series is ambiguous
These errors stem from a misunderstanding of how to correctly perform element-wise comparisons in Pandas.
The Solution
Here’s how you can solve the problem effectively by using the appropriate logical operator and methods within Pandas.
Step-by-Step Solution
Use the Correct Operator: Instead of using and, which checks the truth value of single boolean conditions, you should utilize the bitwise operator &. This operator is designed for element-wise logical operations on Series objects.
Pandas Comparison Methods: To filter your DataFrame, leverage Pandas comparison methods such as .le() for "less than or equal to" and .ge() for "greater than or equal to". These methods will allow you to compare each element efficiently.
Filtering the DataFrame: The final code to extract rows where currentdate is between the Start and End dates looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
df_new['Start'].le(currentdate): This returns a boolean Series indicating where each entry in the Start column is less than or equal to currentdate.
df_new['End'].ge(currentdate): Similarly, this checks if each entry in the End column is greater than or equal to currentdate.
The & operator combines these two conditions to create a final boolean Series that can be used to filter df_new.
Final Thoughts
Filtering rows in a DataFrame based on datetime ranges may seem tricky at first, especially if you're facing errors with boolean logic. By employing the correct logical operator and utilizing the appropriate comparison methods in Pandas, you can easily isolate the data you need. Mastering these techniques will enhance your data manipulation skills, making it easier to work with date ranges in your projects.
Now that you've got a clearer understanding of how to extract rows within a datetime range, feel free to implement this solution in your own DataFrames. Happy coding!
Видео How to Extract Rows Within a datetime Range in Python DataFrames канала vlogize
Extracting rows that satisfy the condition of being within a datetime range, python, datetime
---
This video is based on the question https://stackoverflow.com/q/76724701/ asked by the user '1324241421' ( https://stackoverflow.com/u/14560840/ ) and on the answer https://stackoverflow.com/a/76724716/ provided by the user 'not_speshal' ( https://stackoverflow.com/u/9857631/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Extracting rows that satisfy the condition of being within a datetime range
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Extracting Rows That Fall Within a datetime Range in Python
When working with data in Python, especially in the form of DataFrames using libraries like Pandas, you may encounter situations where you need to filter data based on date ranges. For instance, you might have a DataFrame containing columns for operation start and end dates, and you want to extract rows where a particular date falls between these start and end dates. This guide addresses a common issue that can arise during this process and provides a simple solution.
The Problem
Imagine you have a DataFrame called df_new with the following structure:
Start: The starting date for an operation
End: The ending date for an operation
Your task is to check if a specific currentdate (also in datetime format) is within the range of these start and end dates. However, you're experiencing errors when using boolean operators in your attempts to filter the DataFrame. Specifically, you've encountered errors like:
TypeError: Unsupported operand type(s) for &: 'datetime.datetime' and 'datetime.datetime'
ValueError: The truth value of a Series is ambiguous
These errors stem from a misunderstanding of how to correctly perform element-wise comparisons in Pandas.
The Solution
Here’s how you can solve the problem effectively by using the appropriate logical operator and methods within Pandas.
Step-by-Step Solution
Use the Correct Operator: Instead of using and, which checks the truth value of single boolean conditions, you should utilize the bitwise operator &. This operator is designed for element-wise logical operations on Series objects.
Pandas Comparison Methods: To filter your DataFrame, leverage Pandas comparison methods such as .le() for "less than or equal to" and .ge() for "greater than or equal to". These methods will allow you to compare each element efficiently.
Filtering the DataFrame: The final code to extract rows where currentdate is between the Start and End dates looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
df_new['Start'].le(currentdate): This returns a boolean Series indicating where each entry in the Start column is less than or equal to currentdate.
df_new['End'].ge(currentdate): Similarly, this checks if each entry in the End column is greater than or equal to currentdate.
The & operator combines these two conditions to create a final boolean Series that can be used to filter df_new.
Final Thoughts
Filtering rows in a DataFrame based on datetime ranges may seem tricky at first, especially if you're facing errors with boolean logic. By employing the correct logical operator and utilizing the appropriate comparison methods in Pandas, you can easily isolate the data you need. Mastering these techniques will enhance your data manipulation skills, making it easier to work with date ranges in your projects.
Now that you've got a clearer understanding of how to extract rows within a datetime range, feel free to implement this solution in your own DataFrames. Happy coding!
Видео How to Extract Rows Within a datetime Range in Python DataFrames канала vlogize
Extracting rows that satisfy the condition of being within a datetime range, python, datetime
Показать
Комментарии отсутствуют
Информация о видео
13 ч. 32 мин. назад
00:01:27
Другие видео канала
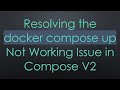


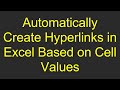

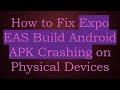
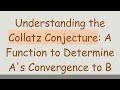

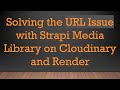
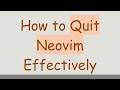

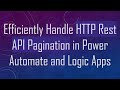
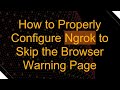

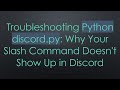

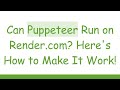
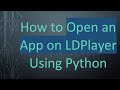
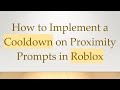

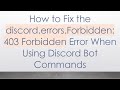