Resolving the useSelector Null Value Issue With next-redux-wrapper in Next.js
Learn how to fix the problem of `useSelector` returning `null` after hydration in Next.js when using `next-redux-wrapper`. Discover the solution to ensure data is accessible seamlessly across your pages.
---
This video is based on the question https://stackoverflow.com/q/74110837/ asked by the user 'Abdul Mahamaliyev' ( https://stackoverflow.com/u/8933996/ ) and on the answer https://stackoverflow.com/a/74119568/ provided by the user 'Abdul Mahamaliyev' ( https://stackoverflow.com/u/8933996/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: next-redux-wrapper: after hydration useSelector returns initial value (null), but getServerSideProps passes the correct value to the page
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the useSelector Behavior with next-redux-wrapper and Next.js
When working with Next.js and Redux, particularly using libraries like next-redux-wrapper, developers can sometimes run into unexpected issues. One common concern is when the useSelector hook returns an initial value (such as null) after hydration, even though the server-side data fetch seems to be functioning correctly. This guide will take you through the issue, explain how the data flows, and present an effective solution to ensure that you can avoid unnecessary prop-drilling and seamlessly access your data across your application.
The Problem Defined
In your Next.js application, you might have set up getServerSideProps to fetch user data based on a token stored in cookies. While this approach works well on the server-side and correctly populates your Redux state during that phase, you may notice that when the application moves to the client-side, useSelector is not returning the expected user data. Instead, it returns null, even though the props passed to the component are accurate.
Example Scenario
Here's a quick rundown of the scenario:
Fetching User Data: You use getServerSideProps to get user data based on a device_access_token from cookies.
Updating the Redux Store: Once you fetch the data, it's dispatched to the Redux store.
Client-side Access: On the indexed page, while the passed auth prop contains correct user information, useSelector(selectCurrentUser) does not reflect this updated state.
Here’s a brief code snippet illustrating the situation:
[[See Video to Reveal this Text or Code Snippet]]
The issue arises because while the props are correct, the selector doesn’t return the expected result.
Unpacking the Solution
After digging into the code, the root cause of the problem became clear. The issue lies in the implementation of how the Redux store is being provided to the application. Specifically, there’s a common mistake when wrapping the main component with both the Provider and the wrapper.withRedux. Here’s how to solve this issue step-by-step:
Step 1: Check Your _app.tsx File
The crucial aspect is examining your _app.tsx. Ensure that you are correctly setting up the Redux provider without redundancy or conflicts. Your implementation should look similar to this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Combine with the Store Properly
When exporting the component using wrapper.withRedux, it should already ensure that the necessary Redux store, including the correct provider, is established. Thus, avoid wrapping the Component with <Provider store={store}> because this could conflict with next-redux-wrapper which has already done that for you.
Step 3: Test the Fix
After making these changes, you should check if useSelector(selectCurrentUser) returns the correct user data. This adjustment should resolve the hydration issue you were encountering.
Conclusion
In conclusion, when facing issues with useSelector returning null after hydration with next-redux-wrapper, one key aspect to verify is the setup in your _app.tsx file. Double-check that you're not redundantly wrapping your component with the Redux provider. Instead, rely on next-redux-wrapper to handle the store injection and provider setup effectively.
By following these guidelines, you can avoid prop-drilling and efficiently utilize selectors to access state throughout your application.
Feel free to implement these changes in your application, and let your Next.js project shine with elegantly managed state!
Видео Resolving the useSelector Null Value Issue With next-redux-wrapper in Next.js канала vlogize
---
This video is based on the question https://stackoverflow.com/q/74110837/ asked by the user 'Abdul Mahamaliyev' ( https://stackoverflow.com/u/8933996/ ) and on the answer https://stackoverflow.com/a/74119568/ provided by the user 'Abdul Mahamaliyev' ( https://stackoverflow.com/u/8933996/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: next-redux-wrapper: after hydration useSelector returns initial value (null), but getServerSideProps passes the correct value to the page
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the useSelector Behavior with next-redux-wrapper and Next.js
When working with Next.js and Redux, particularly using libraries like next-redux-wrapper, developers can sometimes run into unexpected issues. One common concern is when the useSelector hook returns an initial value (such as null) after hydration, even though the server-side data fetch seems to be functioning correctly. This guide will take you through the issue, explain how the data flows, and present an effective solution to ensure that you can avoid unnecessary prop-drilling and seamlessly access your data across your application.
The Problem Defined
In your Next.js application, you might have set up getServerSideProps to fetch user data based on a token stored in cookies. While this approach works well on the server-side and correctly populates your Redux state during that phase, you may notice that when the application moves to the client-side, useSelector is not returning the expected user data. Instead, it returns null, even though the props passed to the component are accurate.
Example Scenario
Here's a quick rundown of the scenario:
Fetching User Data: You use getServerSideProps to get user data based on a device_access_token from cookies.
Updating the Redux Store: Once you fetch the data, it's dispatched to the Redux store.
Client-side Access: On the indexed page, while the passed auth prop contains correct user information, useSelector(selectCurrentUser) does not reflect this updated state.
Here’s a brief code snippet illustrating the situation:
[[See Video to Reveal this Text or Code Snippet]]
The issue arises because while the props are correct, the selector doesn’t return the expected result.
Unpacking the Solution
After digging into the code, the root cause of the problem became clear. The issue lies in the implementation of how the Redux store is being provided to the application. Specifically, there’s a common mistake when wrapping the main component with both the Provider and the wrapper.withRedux. Here’s how to solve this issue step-by-step:
Step 1: Check Your _app.tsx File
The crucial aspect is examining your _app.tsx. Ensure that you are correctly setting up the Redux provider without redundancy or conflicts. Your implementation should look similar to this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Combine with the Store Properly
When exporting the component using wrapper.withRedux, it should already ensure that the necessary Redux store, including the correct provider, is established. Thus, avoid wrapping the Component with <Provider store={store}> because this could conflict with next-redux-wrapper which has already done that for you.
Step 3: Test the Fix
After making these changes, you should check if useSelector(selectCurrentUser) returns the correct user data. This adjustment should resolve the hydration issue you were encountering.
Conclusion
In conclusion, when facing issues with useSelector returning null after hydration with next-redux-wrapper, one key aspect to verify is the setup in your _app.tsx file. Double-check that you're not redundantly wrapping your component with the Redux provider. Instead, rely on next-redux-wrapper to handle the store injection and provider setup effectively.
By following these guidelines, you can avoid prop-drilling and efficiently utilize selectors to access state throughout your application.
Feel free to implement these changes in your application, and let your Next.js project shine with elegantly managed state!
Видео Resolving the useSelector Null Value Issue With next-redux-wrapper in Next.js канала vlogize
Комментарии отсутствуют
Информация о видео
27 марта 2025 г. 5:19:00
00:01:45
Другие видео канала



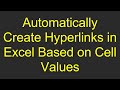


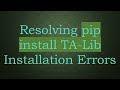
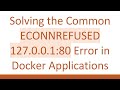

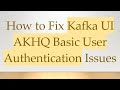
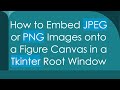
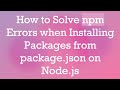
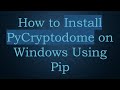
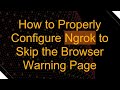
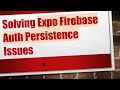
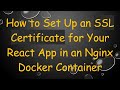
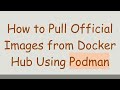

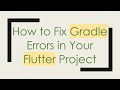
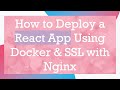
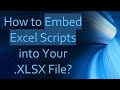