Understanding Label Encoding, Handling Missing Values, and Inverse Transformation in Python
This guide delves into the process of label encoding categorical data, handling missing values with imputations, and using inverse transformation in Python. Join us to uncover the steps needed to clean and prepare your dataset!
---
This video is based on the question https://stackoverflow.com/q/68574230/ asked by the user 'Mario Aguilar' ( https://stackoverflow.com/u/15360508/ ) and on the answer https://stackoverflow.com/a/68584102/ provided by the user 'Andrew Humphrey' ( https://stackoverflow.com/u/12507965/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Label encode then impute missing then inverse encoding
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Data Imputation: Label Encoding, Handling Missing Values, and Inverse Transformation
In the world of data science, overcoming missing values is a significant hurdle to effective data analysis and modeling. If you're working with a dataset that includes key information—like police killings data—and you've encountered missing values in categorical columns, it's essential to handle these values correctly. In this post, we’ll explore a well-structured approach to label encoding, imputing missing values, and finally, performing inverse transformation using Python.
The Challenge: Missing Data in Categorical Columns
When dealing with datasets, it's common to encounter missing values, as highlighted in the example dataset on police killings. The following columns contain varying degrees of missing data:
Age: 1.87%
Gender: 0.06%
Race: 31.74%
City: 0.03%
State: 0.0%
Armed: 45.45%
Such high percentages of missing values, especially in the Race and Armed columns, necessitate a comprehensive handling approach before any analysis can proceed.
The Solution: Step-by-Step Method
Step 1: Label Encoding Categorical Columns
One of the first steps in cleaning up the dataset is to use label encoding for all categorical columns. This process involves converting string values into numeric values that machine learning models can work with. Here's how we can do it with Python's LabelEncoder from the sklearn.preprocessing module:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, each categorical column is encoded, allowing for numerical representation of the categories.
Step 2: Identifying and Replacing NaN Values
Next, it's essential to identify the rows with missing values in the original dataset and replace these values in the encoded dataframe. You can achieve this with:
[[See Video to Reveal this Text or Code Snippet]]
With this code, you can quickly count the occurrences of missing values in any categorical column, guiding your next steps.
For example:
Gender has 8 missing entries.
Race has a total of 3965 missing entries.
City shows few missing entries but is non-trivial owing to its dimensionality.
After determining the number of missing values, we've identified specific indices where these missing values exist and can replace them with np.nan:
[[See Video to Reveal this Text or Code Snippet]]
This process is repeated for each column with missing values.
Step 3: Imputing Missing Values with Iterative Imputation
To handle these np.nan entries, we can apply IterativeImputer:
[[See Video to Reveal this Text or Code Snippet]]
The result is a new dataframe (itimplpf) with imputed values based on the observed data.
Step 4: Inverse Transformation to Recover Original Labels
Lastly, it’s crucial to convert the imputed numeric values back into their original categorical form. However, if you encounter an error during this step (like “contains previously unseen labels”), it’s a sign that some values after imputation correspond to categories that weren’t present in the initial dataset.
To do this, you can use:
[[See Video to Reveal this Text or Code Snippet]]
If errors arise, you may need to revise your imputation strategy or check the completeness of your label encoding.
Conclusion
Encoding categorical variables and imputing missing data not only prepares your dataset for analysis but can also significantly enhance the predictive power of your machine learning models. Given the complexities of encoding and missing data, it may be helpful to consider further methods like using a machine learning model (e.g., Random Forest) to predict and replace missi
Видео Understanding Label Encoding, Handling Missing Values, and Inverse Transformation in Python канала vlogize
---
This video is based on the question https://stackoverflow.com/q/68574230/ asked by the user 'Mario Aguilar' ( https://stackoverflow.com/u/15360508/ ) and on the answer https://stackoverflow.com/a/68584102/ provided by the user 'Andrew Humphrey' ( https://stackoverflow.com/u/12507965/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Label encode then impute missing then inverse encoding
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Data Imputation: Label Encoding, Handling Missing Values, and Inverse Transformation
In the world of data science, overcoming missing values is a significant hurdle to effective data analysis and modeling. If you're working with a dataset that includes key information—like police killings data—and you've encountered missing values in categorical columns, it's essential to handle these values correctly. In this post, we’ll explore a well-structured approach to label encoding, imputing missing values, and finally, performing inverse transformation using Python.
The Challenge: Missing Data in Categorical Columns
When dealing with datasets, it's common to encounter missing values, as highlighted in the example dataset on police killings. The following columns contain varying degrees of missing data:
Age: 1.87%
Gender: 0.06%
Race: 31.74%
City: 0.03%
State: 0.0%
Armed: 45.45%
Such high percentages of missing values, especially in the Race and Armed columns, necessitate a comprehensive handling approach before any analysis can proceed.
The Solution: Step-by-Step Method
Step 1: Label Encoding Categorical Columns
One of the first steps in cleaning up the dataset is to use label encoding for all categorical columns. This process involves converting string values into numeric values that machine learning models can work with. Here's how we can do it with Python's LabelEncoder from the sklearn.preprocessing module:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, each categorical column is encoded, allowing for numerical representation of the categories.
Step 2: Identifying and Replacing NaN Values
Next, it's essential to identify the rows with missing values in the original dataset and replace these values in the encoded dataframe. You can achieve this with:
[[See Video to Reveal this Text or Code Snippet]]
With this code, you can quickly count the occurrences of missing values in any categorical column, guiding your next steps.
For example:
Gender has 8 missing entries.
Race has a total of 3965 missing entries.
City shows few missing entries but is non-trivial owing to its dimensionality.
After determining the number of missing values, we've identified specific indices where these missing values exist and can replace them with np.nan:
[[See Video to Reveal this Text or Code Snippet]]
This process is repeated for each column with missing values.
Step 3: Imputing Missing Values with Iterative Imputation
To handle these np.nan entries, we can apply IterativeImputer:
[[See Video to Reveal this Text or Code Snippet]]
The result is a new dataframe (itimplpf) with imputed values based on the observed data.
Step 4: Inverse Transformation to Recover Original Labels
Lastly, it’s crucial to convert the imputed numeric values back into their original categorical form. However, if you encounter an error during this step (like “contains previously unseen labels”), it’s a sign that some values after imputation correspond to categories that weren’t present in the initial dataset.
To do this, you can use:
[[See Video to Reveal this Text or Code Snippet]]
If errors arise, you may need to revise your imputation strategy or check the completeness of your label encoding.
Conclusion
Encoding categorical variables and imputing missing data not only prepares your dataset for analysis but can also significantly enhance the predictive power of your machine learning models. Given the complexities of encoding and missing data, it may be helpful to consider further methods like using a machine learning model (e.g., Random Forest) to predict and replace missi
Видео Understanding Label Encoding, Handling Missing Values, and Inverse Transformation in Python канала vlogize
Комментарии отсутствуют
Информация о видео
14 апреля 2025 г. 17:06:14
00:02:20
Другие видео канала



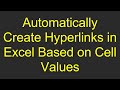


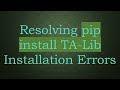
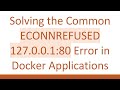

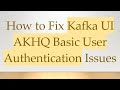
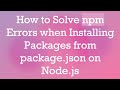
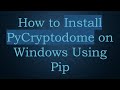
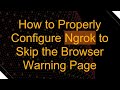
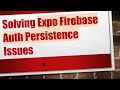
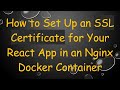
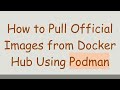

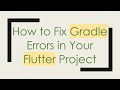
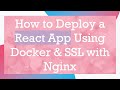
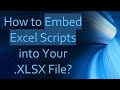
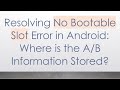