AWS: Frontend web application with Javascript, DynamoDB and Amazon Cognito
This tutorial will explain how to create a serverless and backendless javascript frontend application using DynamoDB and Cognito.
User ► HTML/JS ► Cognito / DynamoDB
Reference of my previous video for more information on AWS using a backend service and API gateway and hosting static HTML files:
https://www.youtube.com/watch?v=SgvkU1yNuGM
My first video where I explain how to configure AWS:
https://www.youtube.com/watch?v=Yl3mCQns4iQ
AWS Javascript API files:
https://github.com/amazon-archives/amazon-cognito-identity-js/tree/master/dist
https://github.com/amazon-archives/amazon-cognito-auth-js/tree/master/dist
Javascript Examples for DynamoDB:
https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/GettingStarted.JavaScript.html
-----
Javascript:
var idToken = null;
function checkLogin() {
var url_string = window.location.href;
var url = new URL(url_string);
idToken = url.searchParams.get("id_token");
if (idToken != null) {
document.getElementById("welcomeMsg").innerHTML = "signed in";
auth();
}
}
function auth() {
AWS.config.update({
region: "us-east-2",
// endpoint: 'http://localhost:8000', // If you use dynamoDB installed locally
// accessKeyId: "(ACCESS_KEY_ID)",
// secretAccessKey: "(SECRET_ACCESS_KEY)"
});
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId : '(IDENTITY POOL ID)',
Logins : {
"cognito-idp.(AWS_REGION).amazonaws.com/(POOL_ID)": idToken
}
});
}
function insertItem() {
var docClient = new AWS.DynamoDB.DocumentClient();
var params = {
TableName :"Person",
Item:{
"FirstName": "John", // Partition Key
"LastName": "Smith", // Sort Key
"info": {
"FavoriteColor": "blue",
"YearOfBirth": 1942
}
}
};
docClient.put(params, function(err, data) {
if (err) {
document.getElementById('textarea').innerHTML = "Unable to add item: " + "\n" + JSON.stringify(err, undefined, 2);
} else {
document.getElementById('textarea').innerHTML = "PutItem succeeded: " + "\n" + JSON.stringify(data, undefined, 2);
}
});
}
function readItem() {
var docClient = new AWS.DynamoDB.DocumentClient();
var params = {
TableName: "Person",
Key:{
"FirstName": "John",// Partition Key
"LastName": "Smith" // Sort/Range Key
}
};
docClient.get(params, function(err, data) {
if (err) {
document.getElementById('textarea').innerHTML = "Unable to read item: " + "\n" + JSON.stringify(err, undefined, 2);
} else {
document.getElementById('textarea').innerHTML = "GetItem succeeded: " + "\n" + JSON.stringify(data, undefined, 2);
}
});
}
-----
Javascript if you want to login internally inside your page.
Recommended video for that: https://www.youtube.com/watch?v=rOaUyCM-fcE
function signInButton() {
var authenticationData = {
Username : document.getElementById("inputUsername").value,
Password : document.getElementById("inputPassword").value,
};
var authenticationDetails = new AmazonCognitoIdentity.AuthenticationDetails(authenticationData);
var poolData = {
UserPoolId : "(POOL_ID)", // Your user pool id here
ClientId : "(APP_CLIENT_ID)", // Your client id here
};
var userPool = new AmazonCognitoIdentity.CognitoUserPool(poolData);
var userData = {
Username : document.getElementById("inputUsername").value,
Pool : userPool,
};
var cognitoUser = new AmazonCognitoIdentity.CognitoUser(userData);
cognitoUser.authenticateUser(authenticationDetails, {
onSuccess: function (result) {
console.log(JSON.stringify(result));
// var accessToken = result.getAccessToken().getJwtToken();
// console.log(accessToken);
},
onFailure: function(err) {
alert(err.message || JSON.stringify(err));
},
});
}
-- Contents of this video --
0:00 Intro
1:52 Configure Cognito
7:40 Create DynamoDB table
8:20 Create IAM role and permission
10:52 More Cognito configuration
16:02 Javascript and HTML code
22:41 Tests and final comments
Видео AWS: Frontend web application with Javascript, DynamoDB and Amazon Cognito канала Learn By Examples
User ► HTML/JS ► Cognito / DynamoDB
Reference of my previous video for more information on AWS using a backend service and API gateway and hosting static HTML files:
https://www.youtube.com/watch?v=SgvkU1yNuGM
My first video where I explain how to configure AWS:
https://www.youtube.com/watch?v=Yl3mCQns4iQ
AWS Javascript API files:
https://github.com/amazon-archives/amazon-cognito-identity-js/tree/master/dist
https://github.com/amazon-archives/amazon-cognito-auth-js/tree/master/dist
Javascript Examples for DynamoDB:
https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/GettingStarted.JavaScript.html
-----
Javascript:
var idToken = null;
function checkLogin() {
var url_string = window.location.href;
var url = new URL(url_string);
idToken = url.searchParams.get("id_token");
if (idToken != null) {
document.getElementById("welcomeMsg").innerHTML = "signed in";
auth();
}
}
function auth() {
AWS.config.update({
region: "us-east-2",
// endpoint: 'http://localhost:8000', // If you use dynamoDB installed locally
// accessKeyId: "(ACCESS_KEY_ID)",
// secretAccessKey: "(SECRET_ACCESS_KEY)"
});
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId : '(IDENTITY POOL ID)',
Logins : {
"cognito-idp.(AWS_REGION).amazonaws.com/(POOL_ID)": idToken
}
});
}
function insertItem() {
var docClient = new AWS.DynamoDB.DocumentClient();
var params = {
TableName :"Person",
Item:{
"FirstName": "John", // Partition Key
"LastName": "Smith", // Sort Key
"info": {
"FavoriteColor": "blue",
"YearOfBirth": 1942
}
}
};
docClient.put(params, function(err, data) {
if (err) {
document.getElementById('textarea').innerHTML = "Unable to add item: " + "\n" + JSON.stringify(err, undefined, 2);
} else {
document.getElementById('textarea').innerHTML = "PutItem succeeded: " + "\n" + JSON.stringify(data, undefined, 2);
}
});
}
function readItem() {
var docClient = new AWS.DynamoDB.DocumentClient();
var params = {
TableName: "Person",
Key:{
"FirstName": "John",// Partition Key
"LastName": "Smith" // Sort/Range Key
}
};
docClient.get(params, function(err, data) {
if (err) {
document.getElementById('textarea').innerHTML = "Unable to read item: " + "\n" + JSON.stringify(err, undefined, 2);
} else {
document.getElementById('textarea').innerHTML = "GetItem succeeded: " + "\n" + JSON.stringify(data, undefined, 2);
}
});
}
-----
Javascript if you want to login internally inside your page.
Recommended video for that: https://www.youtube.com/watch?v=rOaUyCM-fcE
function signInButton() {
var authenticationData = {
Username : document.getElementById("inputUsername").value,
Password : document.getElementById("inputPassword").value,
};
var authenticationDetails = new AmazonCognitoIdentity.AuthenticationDetails(authenticationData);
var poolData = {
UserPoolId : "(POOL_ID)", // Your user pool id here
ClientId : "(APP_CLIENT_ID)", // Your client id here
};
var userPool = new AmazonCognitoIdentity.CognitoUserPool(poolData);
var userData = {
Username : document.getElementById("inputUsername").value,
Pool : userPool,
};
var cognitoUser = new AmazonCognitoIdentity.CognitoUser(userData);
cognitoUser.authenticateUser(authenticationDetails, {
onSuccess: function (result) {
console.log(JSON.stringify(result));
// var accessToken = result.getAccessToken().getJwtToken();
// console.log(accessToken);
},
onFailure: function(err) {
alert(err.message || JSON.stringify(err));
},
});
}
-- Contents of this video --
0:00 Intro
1:52 Configure Cognito
7:40 Create DynamoDB table
8:20 Create IAM role and permission
10:52 More Cognito configuration
16:02 Javascript and HTML code
22:41 Tests and final comments
Видео AWS: Frontend web application with Javascript, DynamoDB and Amazon Cognito канала Learn By Examples
Показать
Комментарии отсутствуют
Информация о видео
Другие видео канала
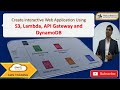

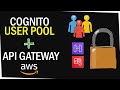

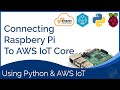
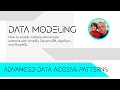
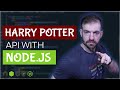
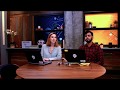
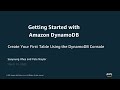
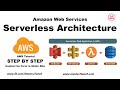
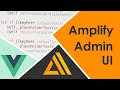

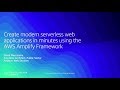
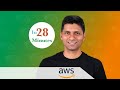
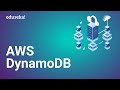

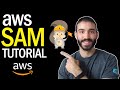
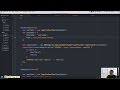
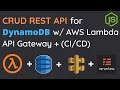
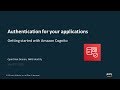