Unlocking the Mystery of Unnamed Namespaces in C++: Access Beyond File Boundaries
Discover how you can access `unnamed namespaces` in C++. Explore the implications of using unnamed namespaces across different files and understand the rules that govern their visibility in C++.
---
This video is based on the question https://stackoverflow.com/q/70299936/ asked by the user 'Vasu saini' ( https://stackoverflow.com/u/17514610/ ) and on the answer https://stackoverflow.com/a/70300054/ provided by the user 'eerorika' ( https://stackoverflow.com/u/2079303/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can we access an unnamed namespace outside the file it is created in?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Unlocking the Mystery of Unnamed Namespaces in C++: Access Beyond File Boundaries
C++ provides developers with powerful features to manage and encapsulate code, and one such feature is namespaces. While most developers are familiar with named namespaces, many are puzzled by unnamed namespaces. They are touted as being exclusively accessible within the file they were created in, but what if that isn't entirely accurate? In this guide, we’ll clarify how access to unnamed namespaces works, especially when it involves multiple files in your project.
What are Unnamed Namespaces?
An unnamed namespace is a special kind of namespace that doesn't have a name. It is used to restrict the visibility of its contents to the file (or translation unit) that includes it. This means that functions, variables, and other entities declared within an unnamed namespace are not visible outside of that particular source file. But, there are some nuances to this rule.
Key Characteristics of Unnamed Namespaces:
File Scope: Entities declared within an unnamed namespace have file-level scope.
No External Access: By default, these entities cannot be accessed outside the source file they were declared in.
The Problem: Accessing Unnamed Namespaces
Let’s refer to a practical scenario based on your question. You attempted to access an unnamed namespace in C++ across multiple files, specifically between file1 and file2. Here's a brief overview of what you were working with:
Code Structure
file1.cpp: Contains an unnamed namespace and a named namespace.
file2.cpp: Includes file1 and attempts to access its contents.
What You Did:
In file1.cpp, you defined an unnamed namespace:
[[See Video to Reveal this Text or Code Snippet]]
Then, in file2.cpp, you included file1.cpp, bypassing the rules you expected:
[[See Video to Reveal this Text or Code Snippet]]
The Reality: Accessing Unnamed Namespaces
So, how can you access this unnamed namespace from outside its own file? The answer lies in understanding translation units and the scope of namespaces.
Translation Units Explained:
A translation unit is essentially a source file plus all the headers and source files included within it.
If you refer to a header file (like file1.cpp included in file2.cpp), the contents of the unnamed namespace become available within the translation unit formed by combining file2.cpp and the included file1.cpp.
Visibility Context:
Within a Single Translation Unit: Access is granted since the unnamed namespace's contents are visible within the same inclusion context.
Across Different Translation Units: If you were to create another file that includes file1 separately from file2, then those entities in the unnamed namespace wouldn’t be accessible.
Conclusion: Managing Access to Unnamed Namespaces
In conclusion, while unnamed namespaces are largely designed to restrict access, their content can be accessed when properly included in the same translation unit. It's essential to remember that these namespaces increase encapsulation within a file but open the door for access through included files. Just ensure that you're working within the same translation unit if you want to avoid any scope-related surprises!
By understanding the rules governing unnamed namespaces, you will be better equipped to manage your C++ projects efficiently.
Feel free to share your thoughts and experiences regarding unnamed namespaces in the comments below!
Видео Unlocking the Mystery of Unnamed Namespaces in C++: Access Beyond File Boundaries канала vlogize
How can we access an unnamed namespace outside the file it is created in?, c++, scope, namespaces
---
This video is based on the question https://stackoverflow.com/q/70299936/ asked by the user 'Vasu saini' ( https://stackoverflow.com/u/17514610/ ) and on the answer https://stackoverflow.com/a/70300054/ provided by the user 'eerorika' ( https://stackoverflow.com/u/2079303/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can we access an unnamed namespace outside the file it is created in?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Unlocking the Mystery of Unnamed Namespaces in C++: Access Beyond File Boundaries
C++ provides developers with powerful features to manage and encapsulate code, and one such feature is namespaces. While most developers are familiar with named namespaces, many are puzzled by unnamed namespaces. They are touted as being exclusively accessible within the file they were created in, but what if that isn't entirely accurate? In this guide, we’ll clarify how access to unnamed namespaces works, especially when it involves multiple files in your project.
What are Unnamed Namespaces?
An unnamed namespace is a special kind of namespace that doesn't have a name. It is used to restrict the visibility of its contents to the file (or translation unit) that includes it. This means that functions, variables, and other entities declared within an unnamed namespace are not visible outside of that particular source file. But, there are some nuances to this rule.
Key Characteristics of Unnamed Namespaces:
File Scope: Entities declared within an unnamed namespace have file-level scope.
No External Access: By default, these entities cannot be accessed outside the source file they were declared in.
The Problem: Accessing Unnamed Namespaces
Let’s refer to a practical scenario based on your question. You attempted to access an unnamed namespace in C++ across multiple files, specifically between file1 and file2. Here's a brief overview of what you were working with:
Code Structure
file1.cpp: Contains an unnamed namespace and a named namespace.
file2.cpp: Includes file1 and attempts to access its contents.
What You Did:
In file1.cpp, you defined an unnamed namespace:
[[See Video to Reveal this Text or Code Snippet]]
Then, in file2.cpp, you included file1.cpp, bypassing the rules you expected:
[[See Video to Reveal this Text or Code Snippet]]
The Reality: Accessing Unnamed Namespaces
So, how can you access this unnamed namespace from outside its own file? The answer lies in understanding translation units and the scope of namespaces.
Translation Units Explained:
A translation unit is essentially a source file plus all the headers and source files included within it.
If you refer to a header file (like file1.cpp included in file2.cpp), the contents of the unnamed namespace become available within the translation unit formed by combining file2.cpp and the included file1.cpp.
Visibility Context:
Within a Single Translation Unit: Access is granted since the unnamed namespace's contents are visible within the same inclusion context.
Across Different Translation Units: If you were to create another file that includes file1 separately from file2, then those entities in the unnamed namespace wouldn’t be accessible.
Conclusion: Managing Access to Unnamed Namespaces
In conclusion, while unnamed namespaces are largely designed to restrict access, their content can be accessed when properly included in the same translation unit. It's essential to remember that these namespaces increase encapsulation within a file but open the door for access through included files. Just ensure that you're working within the same translation unit if you want to avoid any scope-related surprises!
By understanding the rules governing unnamed namespaces, you will be better equipped to manage your C++ projects efficiently.
Feel free to share your thoughts and experiences regarding unnamed namespaces in the comments below!
Видео Unlocking the Mystery of Unnamed Namespaces in C++: Access Beyond File Boundaries канала vlogize
How can we access an unnamed namespace outside the file it is created in?, c++, scope, namespaces
Показать
Комментарии отсутствуют
Информация о видео
3 апреля 2025 г. 16:19:21
00:01:37
Другие видео канала

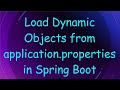
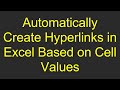
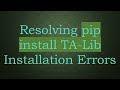
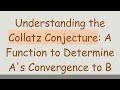


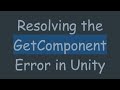

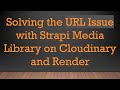
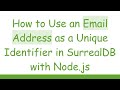
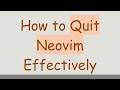
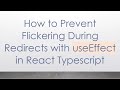
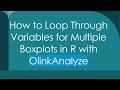
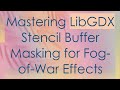

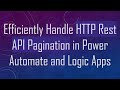
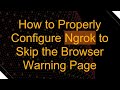
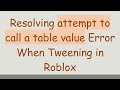

