Understanding the Left Circular Bit Shift in Java
Learn how to implement a left circular bit shift function in Java. This guide explains the concept, provides a step-by-step solution, and helps you overcome common challenges.
---
This video is based on the question https://stackoverflow.com/q/70674496/ asked by the user 'Samuele1818' ( https://stackoverflow.com/u/12119966/ ) and on the answer https://stackoverflow.com/a/70674572/ provided by the user 'Amir Kirsh' ( https://stackoverflow.com/u/2085626/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Left circular bit shift
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Left Circular Bit Shift in Java: A Comprehensive Guide
Bit manipulation is an exciting and essential aspect of programming that opens the door to optimization and efficient coding practices. One common operation involves performing a left circular shift on bits. In this guide, we will explore how to create a function that accomplishes this task in Java, overcoming common challenges along the way.
What is a Left Circular Bit Shift?
A left circular bit shift is a technique where the bits of a binary number are shifted to the left, and the bits that overflow to the left end are wrapped around to the right end of the number. This is commonly used in low-level programming such as embedded systems, cryptography, and performance optimization tasks.
Example
Consider the binary number 01011000. If we perform a left circular shift by 2 positions, the result should be 01100001. Here’s how the bits move:
Initial: 01011000
After shifting left by 2: 11000000
Wrapping the bits around: 01100001
Creating a Left Circular Bit Shift Function in Java
Let's delve into how we can implement the left circular bit shift function in Java.
Step 1: Handling the Input Parameters
To perform a left circular shift, we must accept two parameters: the binary value and the number of positions (y) to shift. However, we need to be cautious with the value of y to ensure it’s within a feasible range.
[[See Video to Reveal this Text or Code Snippet]]
This expression ensures y stays within the bounds of 0 to 7, which corresponds to the valid bit positions for an 8-bit number. Alternatively, a simpler approach to trim down y is by using a bitwise AND operation:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Shifting the Bits
Once we have a valid y, we can shift the bits left by y positions. Here's the critical piece of code:
[[See Video to Reveal this Text or Code Snippet]]
This line serves two purposes:
Shift Left and Truncate: (val << y) & 0xFF shifts the bits to the left and truncates any bits that go beyond the 8th position (only keeping the least significant 8 bits).
Wrap the Overflowed Bits: (val >> (8 - y)) moves the bits that overflowed from the left end back around to the right end of the number.
Final Code Implementation
Here is the complete implementation of the left circular bit shift function in Java:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Implementing a left circular bit shift in Java is a powerful skill that enhances your programming toolkit. By understanding how to manipulate bits and manage conditions effectively, you can employ efficient algorithms that optimize your code's performance. Remember, the key steps include validating the y value, executing the shift and wrap-around correctly, and testing the function to ensure it behaves as expected.
Happy coding! If you have any questions or thoughts, feel free to leave them in the comments below.
Видео Understanding the Left Circular Bit Shift in Java канала vlogize
---
This video is based on the question https://stackoverflow.com/q/70674496/ asked by the user 'Samuele1818' ( https://stackoverflow.com/u/12119966/ ) and on the answer https://stackoverflow.com/a/70674572/ provided by the user 'Amir Kirsh' ( https://stackoverflow.com/u/2085626/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Left circular bit shift
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Left Circular Bit Shift in Java: A Comprehensive Guide
Bit manipulation is an exciting and essential aspect of programming that opens the door to optimization and efficient coding practices. One common operation involves performing a left circular shift on bits. In this guide, we will explore how to create a function that accomplishes this task in Java, overcoming common challenges along the way.
What is a Left Circular Bit Shift?
A left circular bit shift is a technique where the bits of a binary number are shifted to the left, and the bits that overflow to the left end are wrapped around to the right end of the number. This is commonly used in low-level programming such as embedded systems, cryptography, and performance optimization tasks.
Example
Consider the binary number 01011000. If we perform a left circular shift by 2 positions, the result should be 01100001. Here’s how the bits move:
Initial: 01011000
After shifting left by 2: 11000000
Wrapping the bits around: 01100001
Creating a Left Circular Bit Shift Function in Java
Let's delve into how we can implement the left circular bit shift function in Java.
Step 1: Handling the Input Parameters
To perform a left circular shift, we must accept two parameters: the binary value and the number of positions (y) to shift. However, we need to be cautious with the value of y to ensure it’s within a feasible range.
[[See Video to Reveal this Text or Code Snippet]]
This expression ensures y stays within the bounds of 0 to 7, which corresponds to the valid bit positions for an 8-bit number. Alternatively, a simpler approach to trim down y is by using a bitwise AND operation:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Shifting the Bits
Once we have a valid y, we can shift the bits left by y positions. Here's the critical piece of code:
[[See Video to Reveal this Text or Code Snippet]]
This line serves two purposes:
Shift Left and Truncate: (val << y) & 0xFF shifts the bits to the left and truncates any bits that go beyond the 8th position (only keeping the least significant 8 bits).
Wrap the Overflowed Bits: (val >> (8 - y)) moves the bits that overflowed from the left end back around to the right end of the number.
Final Code Implementation
Here is the complete implementation of the left circular bit shift function in Java:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Implementing a left circular bit shift in Java is a powerful skill that enhances your programming toolkit. By understanding how to manipulate bits and manage conditions effectively, you can employ efficient algorithms that optimize your code's performance. Remember, the key steps include validating the y value, executing the shift and wrap-around correctly, and testing the function to ensure it behaves as expected.
Happy coding! If you have any questions or thoughts, feel free to leave them in the comments below.
Видео Understanding the Left Circular Bit Shift in Java канала vlogize
Комментарии отсутствуют
Информация о видео
30 марта 2025 г. 19:32:42
00:01:30
Другие видео канала




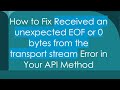
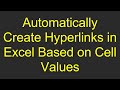

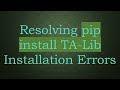

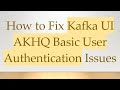

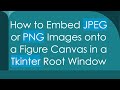
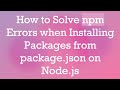
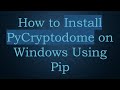
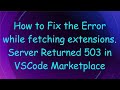
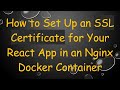
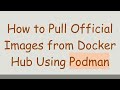


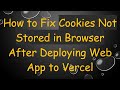
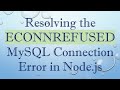