Solving the WebP to JPG Conversion Issue in Python PIL
Learn how to effectively convert an image format from WebP to JPG using Python's PIL library without running into common errors.
---
This video is based on the question https://stackoverflow.com/q/68877883/ asked by the user 'José Guedes' ( https://stackoverflow.com/u/4892527/ ) and on the answer https://stackoverflow.com/a/68878308/ provided by the user 'simpleApp' ( https://stackoverflow.com/u/15568504/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why can't I convert an image I downloaded from WebP to JPG using PIL?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Why You Can't Convert WebP to JPG Using PIL in Python
In the world of web images, formats like WebP and JPG are common, but sometimes you'll run into issues when trying to convert between them using Python's PIL (Python Imaging Library). If you've tried converting a WebP image to JPG and faced errors, you're not alone. This post will explain the problem and provide a clear and effective solution.
Understanding the Problem
When working with images from the web, you often download them using libraries like requests. The challenge arises when you attempt to convert the image format immediately after downloading, as shown in the following code snippets.
Here’s what you might have tried:
[[See Video to Reveal this Text or Code Snippet]]
Or:
[[See Video to Reveal this Text or Code Snippet]]
The Error Message
When you run the above code, you might encounter an error that looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
This error typically indicates that the data fed into Image.open() isn’t in the format that this function expects.
A Clear Solution
To effectively convert WebP images to JPG, you need to ensure that you're correctly handling the image data you download. The flow should be to retrieve the image content, wrap it in a BytesIO object, then pass it to Image.open() for conversion. Here’s how you can do it:
Step-by-Step Code
Here’s a corrected version of the previous attempts that will work seamlessly:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Solution
Download Image: Use requests.get() to fetch the image content from the provided URL.
Wrap Content: Use BytesIO to create an in-memory byte-stream. This allows the PIL library to read the image data directly.
Open and Convert: Use Image.open() to convert the byte-stream to an image, then apply the .convert("RGB") method to change the image mode if needed.
Prepare for Saving or Further Use: Store the converted image in a format ready for saving or further processing.
Conclusion
Converting images from WebP to JPG in Python using PIL can be straightforward once you understand how to handle the image data correctly. By ensuring that you wrap the content with BytesIO before opening it with Image.open(), you'll avoid common pitfalls related to decoding errors.
If you encounter this issue in your projects, simply refer back to this guide to get you back on track. Happy coding!
Видео Solving the WebP to JPG Conversion Issue in Python PIL канала vlogize
Why can't I convert an image I downloaded from WebP to JPG using PIL?, python, python requests, python imaging library
---
This video is based on the question https://stackoverflow.com/q/68877883/ asked by the user 'José Guedes' ( https://stackoverflow.com/u/4892527/ ) and on the answer https://stackoverflow.com/a/68878308/ provided by the user 'simpleApp' ( https://stackoverflow.com/u/15568504/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why can't I convert an image I downloaded from WebP to JPG using PIL?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Why You Can't Convert WebP to JPG Using PIL in Python
In the world of web images, formats like WebP and JPG are common, but sometimes you'll run into issues when trying to convert between them using Python's PIL (Python Imaging Library). If you've tried converting a WebP image to JPG and faced errors, you're not alone. This post will explain the problem and provide a clear and effective solution.
Understanding the Problem
When working with images from the web, you often download them using libraries like requests. The challenge arises when you attempt to convert the image format immediately after downloading, as shown in the following code snippets.
Here’s what you might have tried:
[[See Video to Reveal this Text or Code Snippet]]
Or:
[[See Video to Reveal this Text or Code Snippet]]
The Error Message
When you run the above code, you might encounter an error that looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
This error typically indicates that the data fed into Image.open() isn’t in the format that this function expects.
A Clear Solution
To effectively convert WebP images to JPG, you need to ensure that you're correctly handling the image data you download. The flow should be to retrieve the image content, wrap it in a BytesIO object, then pass it to Image.open() for conversion. Here’s how you can do it:
Step-by-Step Code
Here’s a corrected version of the previous attempts that will work seamlessly:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Solution
Download Image: Use requests.get() to fetch the image content from the provided URL.
Wrap Content: Use BytesIO to create an in-memory byte-stream. This allows the PIL library to read the image data directly.
Open and Convert: Use Image.open() to convert the byte-stream to an image, then apply the .convert("RGB") method to change the image mode if needed.
Prepare for Saving or Further Use: Store the converted image in a format ready for saving or further processing.
Conclusion
Converting images from WebP to JPG in Python using PIL can be straightforward once you understand how to handle the image data correctly. By ensuring that you wrap the content with BytesIO before opening it with Image.open(), you'll avoid common pitfalls related to decoding errors.
If you encounter this issue in your projects, simply refer back to this guide to get you back on track. Happy coding!
Видео Solving the WebP to JPG Conversion Issue in Python PIL канала vlogize
Why can't I convert an image I downloaded from WebP to JPG using PIL?, python, python requests, python imaging library
Показать
Комментарии отсутствуют
Информация о видео
5 апреля 2025 г. 15:05:30
00:01:49
Другие видео канала
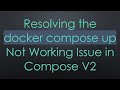


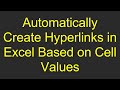

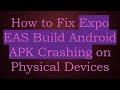
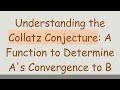


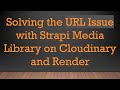
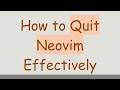

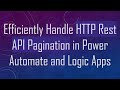
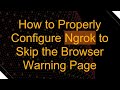

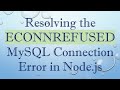

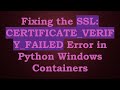

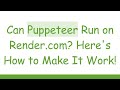
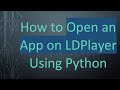