Achieving Persistent Storage in Lambda C++ Functions
Learn how to implement `persistent storage` in Lambda expressions using C++ by creating a unique buffer to store intermediate data between calls.
---
This video is based on the question https://stackoverflow.com/q/71879408/ asked by the user 'user1020788' ( https://stackoverflow.com/u/1020788/ ) and on the answer https://stackoverflow.com/a/71886825/ provided by the user 'user1020788' ( https://stackoverflow.com/u/1020788/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Persistent storage in Lambda C++
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating Persistent Storage in Lambda C++ Functions
In the world of programming, we often face the challenge of managing data flow, especially when working with asynchronous tasks. One common scenario arises when dealing with Lambda functions in C++: how to store intermediate data between calls when the need for persistence is critical. In this guide, we'll dive into a problem faced by many developers, demonstrating how to effectively implement persistent storage in Lambda expressions using C++.
Problem Overview
Imagine you are working with a Lambda function that processes a sequence of inputs, and for each invocation, you require the ability to store intermediary results - essentially allowing your function to “remember” things between calls. This is particularly important in situations where data needs to be preserved due to backpressure or other conditions during processing.
You might write something like the following:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, you might have noticed that even after printing "Storing Tokens," the function doesn't persist the tokens between calls.
Solution to Persistent Storage
To achieve the desired persistent storage in your Lambda function, the solution lies in utilizing a shared_ptr. This approach allows each instantiation of the processing Lambda to maintain its own unique buffer, enabling it to store results even amidst backpressure from the output channel. Here is how to implement it:
Step 1: Create a Shared Pointer
Begin by creating a std::shared_ptr that holds a std::deque to serve as your storage mechanism. This will enable multiple calls to reference the same storage:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Modify the Lambda Function
Next, modify your Lambda function to reference this shared pointer instead of using a local deque:
[[See Video to Reveal this Text or Code Snippet]]
Why This Works
By using a shared_ptr, you ensure that the deque remains alive and accessible across different invocations of the Lambda. Whenever your function runs, it will check if there are existing tokens to read or write, effectively allowing it to accumulate and manage data over time.
Conclusion
Implementing persistent storage in Lambda functions using C++ does not need to be a daunting task. By leveraging shared_ptr to manage your token buffer, you can create robust and efficient solutions that are capable of handling intermediate data between calls. This approach introduces greater flexibility in your code and facilitates complex data handling scenarios in asynchronous programming.
In summary, persistent storage in Lambda expressions is achievable through straightforward modifications, paving the way for more efficient data processing. Happy coding!
Видео Achieving Persistent Storage in Lambda C++ Functions канала vlogize
---
This video is based on the question https://stackoverflow.com/q/71879408/ asked by the user 'user1020788' ( https://stackoverflow.com/u/1020788/ ) and on the answer https://stackoverflow.com/a/71886825/ provided by the user 'user1020788' ( https://stackoverflow.com/u/1020788/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Persistent storage in Lambda C++
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating Persistent Storage in Lambda C++ Functions
In the world of programming, we often face the challenge of managing data flow, especially when working with asynchronous tasks. One common scenario arises when dealing with Lambda functions in C++: how to store intermediate data between calls when the need for persistence is critical. In this guide, we'll dive into a problem faced by many developers, demonstrating how to effectively implement persistent storage in Lambda expressions using C++.
Problem Overview
Imagine you are working with a Lambda function that processes a sequence of inputs, and for each invocation, you require the ability to store intermediary results - essentially allowing your function to “remember” things between calls. This is particularly important in situations where data needs to be preserved due to backpressure or other conditions during processing.
You might write something like the following:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, you might have noticed that even after printing "Storing Tokens," the function doesn't persist the tokens between calls.
Solution to Persistent Storage
To achieve the desired persistent storage in your Lambda function, the solution lies in utilizing a shared_ptr. This approach allows each instantiation of the processing Lambda to maintain its own unique buffer, enabling it to store results even amidst backpressure from the output channel. Here is how to implement it:
Step 1: Create a Shared Pointer
Begin by creating a std::shared_ptr that holds a std::deque to serve as your storage mechanism. This will enable multiple calls to reference the same storage:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Modify the Lambda Function
Next, modify your Lambda function to reference this shared pointer instead of using a local deque:
[[See Video to Reveal this Text or Code Snippet]]
Why This Works
By using a shared_ptr, you ensure that the deque remains alive and accessible across different invocations of the Lambda. Whenever your function runs, it will check if there are existing tokens to read or write, effectively allowing it to accumulate and manage data over time.
Conclusion
Implementing persistent storage in Lambda functions using C++ does not need to be a daunting task. By leveraging shared_ptr to manage your token buffer, you can create robust and efficient solutions that are capable of handling intermediate data between calls. This approach introduces greater flexibility in your code and facilitates complex data handling scenarios in asynchronous programming.
In summary, persistent storage in Lambda expressions is achievable through straightforward modifications, paving the way for more efficient data processing. Happy coding!
Видео Achieving Persistent Storage in Lambda C++ Functions канала vlogize
Комментарии отсутствуют
Информация о видео
26 марта 2025 г. 3:16:02
00:01:58
Другие видео канала




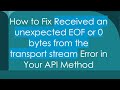
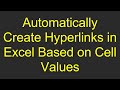

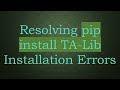

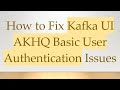
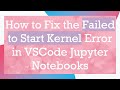
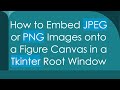


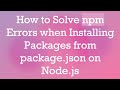
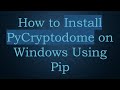
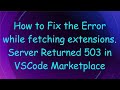
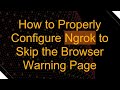
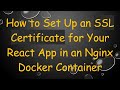
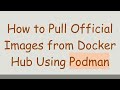
