Introduction to Python Classes & Objects in the context of ML
Code: https://colab.research.google.com/drive/1MQ-GfHjClO8kMOs8YIR7lUUf4-R0iGy5?usp=sharing
Neural Network from scratch: https://www.youtube.com/watch?v=A83BbHFoKb8&ab_channel=Vizuara
Notes link: https://miro.com/app/board/uXjVIMtrsNw=/?share_link_id=480195777402
📘 Python Classes for Machine Learning: Linear Regression & Neural Network Explained | Beginner-Friendly
Description:
💥 Want to understand Python classes AND Machine Learning in one go?
In this hands-on, beginner-friendly lecture, we explore how Python’s object-oriented programming (OOP) — especially classes — can be used to build real ML models like Linear Regression and a Simple Neural Network from scratch.
This isn’t just a tutorial — it’s a step-by-step guided class where we build two ML models from the ground up using Python classes, while also learning why you should use classes instead of plain functions in machine learning code.
🧠 What You’ll Learn:
What are Python classes and how they work
Key concepts: __init__(), self, attributes, methods
Difference between a class and a function, and why classes are more powerful
Building a Linear Regression model using a Python class
Implementing a simple Neural Network using OOP
How model parameters like weights and biases are stored inside class objects
How to train, evaluate, and predict using ML models structured as classes
🛠️ Models Built in This Video:
Linear Regression from Scratch
🎯 Predict house prices based on size
💡 Learn how to minimize loss using gradient descent
🧱 Implemented using Python class step by step
Simple Neural Network
🔍 Task: Classify whether x2 greater than x1 (binary classification)
🧠 One hidden layer, ReLU + Sigmoid activations
🧪 Implemented with forward pass, backpropagation & loss computation
🧰 Fully class-based with encapsulated logic
📚 Time Stamps:
00:00 – Intro: What is this lecture about?
00:32 – 4 Things You’ll Learn
01:00 – Understanding Classes with Real-World Examples
05:00 – Building a Dog class in Python
07:00 – Why __init__ and self are useful
09:00 – Difference between Object vs Class
10:00 – Introduction to Linear Regression
12:00 – What is Mean Squared Error?
14:00 – Gradient Descent explained simply
20:00 – Full implementation of Linear Regression using a class
30:00 – Predicting new data using trained model
35:00 – Why use classes instead of functions? (Clear explanation)
37:00 – Intro to Neural Networks
39:00 – Neural Network structure & logic
44:00 – Implementing Neural Network using a class
50:00 – Testing predictions: Does it work?
55:00 – Final summary and why classes are used in real ML libraries
💻 Access the Code:
📓 Google Colab Notebook (Link in pinned comment & description)
🔗 Play with the model, tweak hyperparameters, and explore!
📦 Bonus:
🎁 I’ve added test cases, new dataset examples, and how to create multiple models using different objects from the same class!
🙋♂️ For Beginners Who:
Struggle with understanding how Python classes work
Want to build ML models from scratch without relying on libraries
Are curious why ML libraries like Scikit-learn, PyTorch, and TensorFlow all use classes
Want a clean, elegant, scalable way to structure their ML code
📢 Watch Next:
👉 Learn Neural Networks from Scratch in 60 Minutes
👉 Gradient Descent Visualized Intuitively
👉 OOP Concepts in Python Explained Simply
💬 Drop a comment if:
You have a question
You want me to add more projects using classes
You found this helpful and want a part 2
Like 👍 | Share 🔁 | Subscribe 🔔
Thanks for learning with me — see you in the next one!
Видео Introduction to Python Classes & Objects in the context of ML канала Vizuara
machine learning, python, objects, classes, machine learning foundations, object oriented programming, oop
Neural Network from scratch: https://www.youtube.com/watch?v=A83BbHFoKb8&ab_channel=Vizuara
Notes link: https://miro.com/app/board/uXjVIMtrsNw=/?share_link_id=480195777402
📘 Python Classes for Machine Learning: Linear Regression & Neural Network Explained | Beginner-Friendly
Description:
💥 Want to understand Python classes AND Machine Learning in one go?
In this hands-on, beginner-friendly lecture, we explore how Python’s object-oriented programming (OOP) — especially classes — can be used to build real ML models like Linear Regression and a Simple Neural Network from scratch.
This isn’t just a tutorial — it’s a step-by-step guided class where we build two ML models from the ground up using Python classes, while also learning why you should use classes instead of plain functions in machine learning code.
🧠 What You’ll Learn:
What are Python classes and how they work
Key concepts: __init__(), self, attributes, methods
Difference between a class and a function, and why classes are more powerful
Building a Linear Regression model using a Python class
Implementing a simple Neural Network using OOP
How model parameters like weights and biases are stored inside class objects
How to train, evaluate, and predict using ML models structured as classes
🛠️ Models Built in This Video:
Linear Regression from Scratch
🎯 Predict house prices based on size
💡 Learn how to minimize loss using gradient descent
🧱 Implemented using Python class step by step
Simple Neural Network
🔍 Task: Classify whether x2 greater than x1 (binary classification)
🧠 One hidden layer, ReLU + Sigmoid activations
🧪 Implemented with forward pass, backpropagation & loss computation
🧰 Fully class-based with encapsulated logic
📚 Time Stamps:
00:00 – Intro: What is this lecture about?
00:32 – 4 Things You’ll Learn
01:00 – Understanding Classes with Real-World Examples
05:00 – Building a Dog class in Python
07:00 – Why __init__ and self are useful
09:00 – Difference between Object vs Class
10:00 – Introduction to Linear Regression
12:00 – What is Mean Squared Error?
14:00 – Gradient Descent explained simply
20:00 – Full implementation of Linear Regression using a class
30:00 – Predicting new data using trained model
35:00 – Why use classes instead of functions? (Clear explanation)
37:00 – Intro to Neural Networks
39:00 – Neural Network structure & logic
44:00 – Implementing Neural Network using a class
50:00 – Testing predictions: Does it work?
55:00 – Final summary and why classes are used in real ML libraries
💻 Access the Code:
📓 Google Colab Notebook (Link in pinned comment & description)
🔗 Play with the model, tweak hyperparameters, and explore!
📦 Bonus:
🎁 I’ve added test cases, new dataset examples, and how to create multiple models using different objects from the same class!
🙋♂️ For Beginners Who:
Struggle with understanding how Python classes work
Want to build ML models from scratch without relying on libraries
Are curious why ML libraries like Scikit-learn, PyTorch, and TensorFlow all use classes
Want a clean, elegant, scalable way to structure their ML code
📢 Watch Next:
👉 Learn Neural Networks from Scratch in 60 Minutes
👉 Gradient Descent Visualized Intuitively
👉 OOP Concepts in Python Explained Simply
💬 Drop a comment if:
You have a question
You want me to add more projects using classes
You found this helpful and want a part 2
Like 👍 | Share 🔁 | Subscribe 🔔
Thanks for learning with me — see you in the next one!
Видео Introduction to Python Classes & Objects in the context of ML канала Vizuara
machine learning, python, objects, classes, machine learning foundations, object oriented programming, oop
Показать
Комментарии отсутствуют
Информация о видео
24 марта 2025 г. 14:30:06
00:55:42
Другие видео канала
![Enhance ML Model Accuracy with Hyperparameter Tuning: Grid Search vs. Random Search [Lecture 16]](https://i.ytimg.com/vi/cIFngVWhETU/default.jpg)
![What exactly does version control mean in Git? [Lecture 3]](https://i.ytimg.com/vi/AbGRsCNHsyw/default.jpg)
![Probability Distribution | Normal Distribution | Gaussian Distribution | CDF PDF [Lecture 7]](https://i.ytimg.com/vi/ZErgGvZXpKM/default.jpg)
![What are Git branches? | A lecture for beginners [Lecture 8]](https://i.ytimg.com/vi/ThpSIYuYfZ8/default.jpg)
![GitHub for students [Lecture 14]](https://i.ytimg.com/vi/y6-Jb6zApxc/default.jpg)

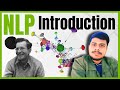
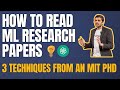

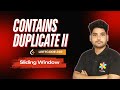

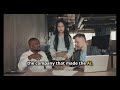

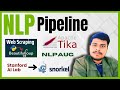

![K-Fold Cross-Validation: A Complete Guide for Model Evaluation | In Hindi [Lecture 17]](https://i.ytimg.com/vi/9VNcB2oxPI4/default.jpg)


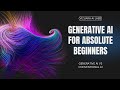
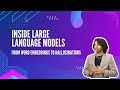
