How to Update Multiple Entries of a Certain Column in a Pandas Dataframe
Learn how to update multiple entries in a Pandas dataframe column based on a specific order using mapping techniques.
---
This video is based on the question https://stackoverflow.com/q/73838199/ asked by the user 'Shaun Han' ( https://stackoverflow.com/u/13860719/ ) and on the answer https://stackoverflow.com/a/73838477/ provided by the user 'Quang Hoang' ( https://stackoverflow.com/u/4238408/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to update multiple entries of a certain column of a Pandas dataframe in certain order?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Update Multiple Entries of a Certain Column in a Pandas Dataframe
Updating multiple entries in a dataframe can be tricky, especially when you need to maintain a specific order for your updates. In this post, we will address a common problem faced by data analysts and developers when working with Pandas, a powerful data manipulation library in Python. Let's dive into the scenario, understand the challenge, and explore the solution step by step.
The Problem
Imagine you have a Pandas dataframe that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This will output:
[[See Video to Reveal this Text or Code Snippet]]
In this dataframe, the key column contains unique strings, and you want to update the corresponding value column entries based on a predefined list of keys and values:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to update the value column such that:
Key 'a' should map to value 1
Key 'b' should map to value 2
Key 'c' should map to value 3
Key 'd' should map to value 4
However, when you use the following code to perform the update, you may encounter unexpected results:
[[See Video to Reveal this Text or Code Snippet]]
The output may not be as expected, often leading to a mismatch in the values assigned to the keys.
The Solution
To achieve the desired result, we can utilize the map function in Pandas, which allows us to map values from one column based on another column's corresponding keys efficiently. Here’s how to do it:
Step-by-Step Guide
Create a Mapping Dictionary: Use the zip function to create a dictionary that pairs each key with its corresponding value.
[[See Video to Reveal this Text or Code Snippet]]
This dd dictionary will look like:
[[See Video to Reveal this Text or Code Snippet]]
Update the DataFrame Using map: Next, we will use the map method on the key column to update the value column.
[[See Video to Reveal this Text or Code Snippet]]
Here, the fillna function ensures that any keys not found in the dd dictionary will retain their original values, preventing the loss of data in other rows.
Final Output: Running the above code will now give you the desired dataframe:
[[See Video to Reveal this Text or Code Snippet]]
Which outputs:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following the steps outlined above, you can now easily update multiple entries in a Pandas dataframe based on specific orders without running into confusion. Using the map function streamlines the process and ensures data integrity. This method is not only applicable in this scenario but can also be generally useful when working with large datasets that require similar transformations. Happy coding!
Видео How to Update Multiple Entries of a Certain Column in a Pandas Dataframe канала vlogize
---
This video is based on the question https://stackoverflow.com/q/73838199/ asked by the user 'Shaun Han' ( https://stackoverflow.com/u/13860719/ ) and on the answer https://stackoverflow.com/a/73838477/ provided by the user 'Quang Hoang' ( https://stackoverflow.com/u/4238408/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to update multiple entries of a certain column of a Pandas dataframe in certain order?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Update Multiple Entries of a Certain Column in a Pandas Dataframe
Updating multiple entries in a dataframe can be tricky, especially when you need to maintain a specific order for your updates. In this post, we will address a common problem faced by data analysts and developers when working with Pandas, a powerful data manipulation library in Python. Let's dive into the scenario, understand the challenge, and explore the solution step by step.
The Problem
Imagine you have a Pandas dataframe that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
This will output:
[[See Video to Reveal this Text or Code Snippet]]
In this dataframe, the key column contains unique strings, and you want to update the corresponding value column entries based on a predefined list of keys and values:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to update the value column such that:
Key 'a' should map to value 1
Key 'b' should map to value 2
Key 'c' should map to value 3
Key 'd' should map to value 4
However, when you use the following code to perform the update, you may encounter unexpected results:
[[See Video to Reveal this Text or Code Snippet]]
The output may not be as expected, often leading to a mismatch in the values assigned to the keys.
The Solution
To achieve the desired result, we can utilize the map function in Pandas, which allows us to map values from one column based on another column's corresponding keys efficiently. Here’s how to do it:
Step-by-Step Guide
Create a Mapping Dictionary: Use the zip function to create a dictionary that pairs each key with its corresponding value.
[[See Video to Reveal this Text or Code Snippet]]
This dd dictionary will look like:
[[See Video to Reveal this Text or Code Snippet]]
Update the DataFrame Using map: Next, we will use the map method on the key column to update the value column.
[[See Video to Reveal this Text or Code Snippet]]
Here, the fillna function ensures that any keys not found in the dd dictionary will retain their original values, preventing the loss of data in other rows.
Final Output: Running the above code will now give you the desired dataframe:
[[See Video to Reveal this Text or Code Snippet]]
Which outputs:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following the steps outlined above, you can now easily update multiple entries in a Pandas dataframe based on specific orders without running into confusion. Using the map function streamlines the process and ensures data integrity. This method is not only applicable in this scenario but can also be generally useful when working with large datasets that require similar transformations. Happy coding!
Видео How to Update Multiple Entries of a Certain Column in a Pandas Dataframe канала vlogize
Комментарии отсутствуют
Информация о видео
14 апреля 2025 г. 15:42:23
00:01:42
Другие видео канала
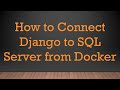
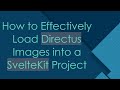


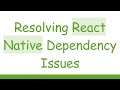
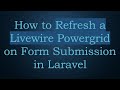
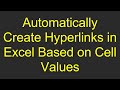



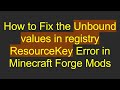

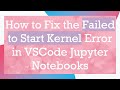
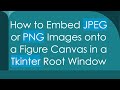

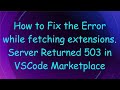
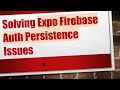
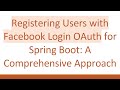

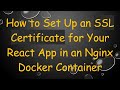
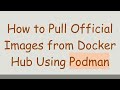