Mastering Dynamic Memory Allocation for Matrices in C: A Guide to Double and Triple Pointers
Learn how to efficiently allocate dynamic memory for matrices in C using double and triple pointers. Understand the differences and best practices on matrix handling in C programming.
---
This video is based on the question https://stackoverflow.com/q/77914520/ asked by the user 'user23326904' ( https://stackoverflow.com/u/23326904/ ) and on the answer https://stackoverflow.com/a/77914766/ provided by the user 'John Bollinger' ( https://stackoverflow.com/u/2402272/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is it correct this declaration of a matrix as a double pointer in C?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Dynamic Memory Allocation for Matrices in C: A Guide to Double and Triple Pointers
Dynamic memory allocation is a powerful feature in C programming that allows you to create data structures of varying sizes at runtime. One common task where this feature is particularly useful is when working with matrices. If you're new to C and find yourself confused about how to declare and initialize matrices using pointers, you’re not alone. In this post, we’ll clarify how to correctly declare a matrix as a double pointer and when you might need a triple pointer.
Understanding the Problem
You may have encountered a situation where you need to allocate memory for a matrix with n rows and m columns. The challenge arises when trying to determine the correct pointer type to use and how to allocate memory efficiently. A common starting point is to use a double pointer (double **matrix) to represent your matrix, but you might have seen some examples using triple pointers instead and wondered about the difference.
The Double Pointer Approach
Let's start by examining the code you provided. You’re using a double pointer for your matrix:
[[See Video to Reveal this Text or Code Snippet]]
Memory Structure Explained
First Allocation: The first line of allocation (using malloc(n * sizeof(double*))) creates an array of pointers. Each pointer will later point to a row of the matrix.
Second Allocation: The second line of allocation (inside the loop) allocates memory for each individual row, where each row holds m double values.
Thus, your understanding was mostly correct: you are indeed allocating space for the first element of each row before allocating memory for each element of the rows themselves.
The Triple Pointer Confusion
You might be asking yourself why some examples use a triple pointer (e.g., double ***matrix). This is primarily used in cases where you want to allow a function to modify the original pointer to the matrix itself, not just the data it points to. However, in your code, you're not using this mechanism—your goal is simply to initialize and print matrix values.
Is Your Code Correct?
Yes! Your use of a double pointer is appropriate for your needs. Your code correctly allocates memory and accesses the matrix. Just remember the actual structure: you have an array (points to rows) of double arrays (each row contains values). While it's a common approach, there are other efficient methods for representing matrices, especially if you are dealing with fixed sizes.
Alternative Approaches
If matrix dimensions are fixed or can be defined using variable-length types, consider using a single allocation for the entire matrix:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of a Contiguous Memory Allocation
Performance: Accessing elements through a contiguous block of memory is often faster due to better cache performance.
Simplicity: You only need one free(matrix) call at the end instead of freeing each row individually.
Here's what the complete function might look like:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Dynamic memory management in C can be complex, especially when dealing with multidimensional arrays. While using double pointers is perfectly valid for matrix representation, understanding the underlying memory structure and alternative approaches will help improve both the efficiency and clarity of your code.
By grasping these concepts, you'll be able to handle matrices with confidence in your C programs, ensuring that they run smoothly and efficiently. If you ever encounter doubts or confusion, remember, practice is key to mastering C programming!
Видео Mastering Dynamic Memory Allocation for Matrices in C: A Guide to Double and Triple Pointers канала vlogize
Is it correct this declaration of a matrix as a double pointer in C?, pointers, matrix
---
This video is based on the question https://stackoverflow.com/q/77914520/ asked by the user 'user23326904' ( https://stackoverflow.com/u/23326904/ ) and on the answer https://stackoverflow.com/a/77914766/ provided by the user 'John Bollinger' ( https://stackoverflow.com/u/2402272/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is it correct this declaration of a matrix as a double pointer in C?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Dynamic Memory Allocation for Matrices in C: A Guide to Double and Triple Pointers
Dynamic memory allocation is a powerful feature in C programming that allows you to create data structures of varying sizes at runtime. One common task where this feature is particularly useful is when working with matrices. If you're new to C and find yourself confused about how to declare and initialize matrices using pointers, you’re not alone. In this post, we’ll clarify how to correctly declare a matrix as a double pointer and when you might need a triple pointer.
Understanding the Problem
You may have encountered a situation where you need to allocate memory for a matrix with n rows and m columns. The challenge arises when trying to determine the correct pointer type to use and how to allocate memory efficiently. A common starting point is to use a double pointer (double **matrix) to represent your matrix, but you might have seen some examples using triple pointers instead and wondered about the difference.
The Double Pointer Approach
Let's start by examining the code you provided. You’re using a double pointer for your matrix:
[[See Video to Reveal this Text or Code Snippet]]
Memory Structure Explained
First Allocation: The first line of allocation (using malloc(n * sizeof(double*))) creates an array of pointers. Each pointer will later point to a row of the matrix.
Second Allocation: The second line of allocation (inside the loop) allocates memory for each individual row, where each row holds m double values.
Thus, your understanding was mostly correct: you are indeed allocating space for the first element of each row before allocating memory for each element of the rows themselves.
The Triple Pointer Confusion
You might be asking yourself why some examples use a triple pointer (e.g., double ***matrix). This is primarily used in cases where you want to allow a function to modify the original pointer to the matrix itself, not just the data it points to. However, in your code, you're not using this mechanism—your goal is simply to initialize and print matrix values.
Is Your Code Correct?
Yes! Your use of a double pointer is appropriate for your needs. Your code correctly allocates memory and accesses the matrix. Just remember the actual structure: you have an array (points to rows) of double arrays (each row contains values). While it's a common approach, there are other efficient methods for representing matrices, especially if you are dealing with fixed sizes.
Alternative Approaches
If matrix dimensions are fixed or can be defined using variable-length types, consider using a single allocation for the entire matrix:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of a Contiguous Memory Allocation
Performance: Accessing elements through a contiguous block of memory is often faster due to better cache performance.
Simplicity: You only need one free(matrix) call at the end instead of freeing each row individually.
Here's what the complete function might look like:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Dynamic memory management in C can be complex, especially when dealing with multidimensional arrays. While using double pointers is perfectly valid for matrix representation, understanding the underlying memory structure and alternative approaches will help improve both the efficiency and clarity of your code.
By grasping these concepts, you'll be able to handle matrices with confidence in your C programs, ensuring that they run smoothly and efficiently. If you ever encounter doubts or confusion, remember, practice is key to mastering C programming!
Видео Mastering Dynamic Memory Allocation for Matrices in C: A Guide to Double and Triple Pointers канала vlogize
Is it correct this declaration of a matrix as a double pointer in C?, pointers, matrix
Показать
Комментарии отсутствуют
Информация о видео
5 апреля 2025 г. 20:40:25
00:01:47
Другие видео канала
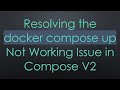


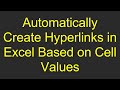

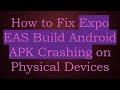
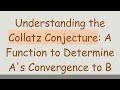

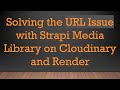
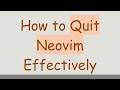

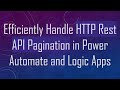
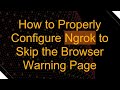


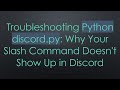

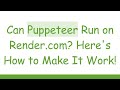
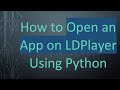
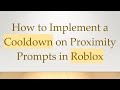
