Top 5 Java Coding Interview Questions: Solutions and Explanations #java #interview #programming
Description: Essential Java Coding Interview Questions and Answers.
Overview:
In this comprehensive video, we delve into five critical coding challenges that are commonly encountered in Java interviews. Whether you're a beginner or an experienced programmer, these problems will help you sharpen your skills and prepare for technical interviews.
Topics Covered
1. Finding the Second Highest Number in an Array
We start by solving a classic problem: finding the second highest number in a given array of integers.
int[] numbers = {5, 9, 11, 2, 8, 21, 1};
We will walk through the step-by-step solution to identify the second largest element efficiently.
2. Identifying the Longest String in an Array
Next, we tackle the task of determining the longest string from a given array of strings.
String[] strArray = {"java", "geeks", "springboot", "microservice"};
This section covers how to iterate through the array and find the longest string using Java.
3. Finding Elements Starting with '1'
In this segment, we explore how to filter elements from an array that start with '1'.
int[] a = {2, 5, 11, 10, 20, 13};
We will demonstrate how to use Java's built-in methods and loops to achieve this.
4. Eliminating Duplicate Elements and Displaying First Three Unique Elements
Here we address another common problem: removing duplicate elements from an array and displaying only the first three unique elements.
int[] number = {5, 9, 11, 9, 8, 11, 1};
This part includes using sets or other data structures to eliminate duplicates and then displaying the first three unique elements.
5. Printing Integer Numbers from 1 to 100 Using Java 8 Features
Finally, we show how to leverage Java 8 features such as streams to print integer numbers from 1 to 100 efficiently.
IntStream.rangeClosed(1, 100).forEach(System.out::println);
What You Will Learn
How to solve common coding interview problems in Java
Efficient algorithms for finding second highest numbers and longest strings
Techniques for filtering arrays based on specific criteria
Methods for eliminating duplicate elements in arrays
Modern Java 8 features like streams for simple tasks
Who Should Watch This Video?
Beginners looking to improve their coding skills in Java
Experienced programmers preparing for technical interviews
Anyone interested in learning practical solutions to common coding challenges
Видео Top 5 Java Coding Interview Questions: Solutions and Explanations #java #interview #programming канала Java Geeks
Overview:
In this comprehensive video, we delve into five critical coding challenges that are commonly encountered in Java interviews. Whether you're a beginner or an experienced programmer, these problems will help you sharpen your skills and prepare for technical interviews.
Topics Covered
1. Finding the Second Highest Number in an Array
We start by solving a classic problem: finding the second highest number in a given array of integers.
int[] numbers = {5, 9, 11, 2, 8, 21, 1};
We will walk through the step-by-step solution to identify the second largest element efficiently.
2. Identifying the Longest String in an Array
Next, we tackle the task of determining the longest string from a given array of strings.
String[] strArray = {"java", "geeks", "springboot", "microservice"};
This section covers how to iterate through the array and find the longest string using Java.
3. Finding Elements Starting with '1'
In this segment, we explore how to filter elements from an array that start with '1'.
int[] a = {2, 5, 11, 10, 20, 13};
We will demonstrate how to use Java's built-in methods and loops to achieve this.
4. Eliminating Duplicate Elements and Displaying First Three Unique Elements
Here we address another common problem: removing duplicate elements from an array and displaying only the first three unique elements.
int[] number = {5, 9, 11, 9, 8, 11, 1};
This part includes using sets or other data structures to eliminate duplicates and then displaying the first three unique elements.
5. Printing Integer Numbers from 1 to 100 Using Java 8 Features
Finally, we show how to leverage Java 8 features such as streams to print integer numbers from 1 to 100 efficiently.
IntStream.rangeClosed(1, 100).forEach(System.out::println);
What You Will Learn
How to solve common coding interview problems in Java
Efficient algorithms for finding second highest numbers and longest strings
Techniques for filtering arrays based on specific criteria
Methods for eliminating duplicate elements in arrays
Modern Java 8 features like streams for simple tasks
Who Should Watch This Video?
Beginners looking to improve their coding skills in Java
Experienced programmers preparing for technical interviews
Anyone interested in learning practical solutions to common coding challenges
Видео Top 5 Java Coding Interview Questions: Solutions and Explanations #java #interview #programming канала Java Geeks
Комментарии отсутствуют
Информация о видео
27 ноября 2024 г. 10:20:42
00:09:46
Другие видео канала
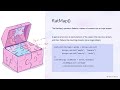

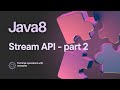
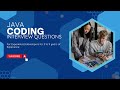
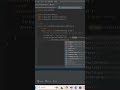

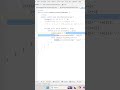


