Navigating A Matrix with Recursion: Overcoming Obstacles with Python
Learn how to navigate a binary `matrix` using a recursive function in Python, ensuring you avoid obstacles while reaching your destination.
---
This video is based on the question https://stackoverflow.com/q/72848479/ asked by the user 'PythonNoob' ( https://stackoverflow.com/u/19366797/ ) and on the answer https://stackoverflow.com/a/72848514/ provided by the user 'Game Developement' ( https://stackoverflow.com/u/16715608/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Check the values of a matrix and jump in columns, using a recursion function
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Navigating A Matrix with Recursion: Overcoming Obstacles with Python
Working with matrices can often present unique challenges, particularly when obstacles are present in your intended path. In this guide, we'll explore how to navigate a binary matrix containing only 0s and 1s using a recursive approach in Python. Our goal is to move from the first column to the last column while avoiding 1s, which act as barriers.
The Problem: Understanding the Matrix
Consider a binary matrix structured like this:
[[See Video to Reveal this Text or Code Snippet]]
Here’s the essence of the task:
You start at the first column (j=0).
You can move to three potential positions in the next column (j+ 1):
Downwards: [i+ 1][j+ 1]
Forward: [i][j+ 1]
Upwards: [i-1][j+ 1]
Your aim is to navigate to the last column (len(matrix[0]) - 1), preferably in the lowest row possible (i).
The Solution: A Recursive Approach
To achieve this, you will use a recursive function that examines each movement option at each step. Let’s break down the solution step-by-step.
1. Define the Recursive Function
The first step is to define our recursive function, jumpFromOnes(matrix, i, j), which accepts the matrix and the current row and column indices.
2. Set Base Cases
At the core of recursion, base cases determine when the recursion should stop. In our case, these are:
If you reach the last row: if i == len(matrix) - 1.
If you reach the last column: if j == len(matrix[0]) - 1.
3. Recursion Logic
The function should check whether it's possible to move to the next three potential positions:
[[See Video to Reveal this Text or Code Snippet]]
4. Handling Dead Ends
If none of the movement options are available (when hitting a 1), the function should return a message indicating that it’s impossible to continue:
[[See Video to Reveal this Text or Code Snippet]]
Full Implementation
Here’s how the entire function looks, integrating all the parts discussed:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In this guide, we covered how to structure a recursive function in Python to navigate through a binary matrix filled with obstacles (1s) while seeking a path towards the last column. This tips not only help you solve matrix navigation problems but also strengthen your understanding of recursion in programming. You can adapt, modify, and leverage this logic to various applications where matrix navigation or obstacle avoidance is required.
Happy coding!
Видео Navigating A Matrix with Recursion: Overcoming Obstacles with Python канала vlogize
---
This video is based on the question https://stackoverflow.com/q/72848479/ asked by the user 'PythonNoob' ( https://stackoverflow.com/u/19366797/ ) and on the answer https://stackoverflow.com/a/72848514/ provided by the user 'Game Developement' ( https://stackoverflow.com/u/16715608/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Check the values of a matrix and jump in columns, using a recursion function
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Navigating A Matrix with Recursion: Overcoming Obstacles with Python
Working with matrices can often present unique challenges, particularly when obstacles are present in your intended path. In this guide, we'll explore how to navigate a binary matrix containing only 0s and 1s using a recursive approach in Python. Our goal is to move from the first column to the last column while avoiding 1s, which act as barriers.
The Problem: Understanding the Matrix
Consider a binary matrix structured like this:
[[See Video to Reveal this Text or Code Snippet]]
Here’s the essence of the task:
You start at the first column (j=0).
You can move to three potential positions in the next column (j+ 1):
Downwards: [i+ 1][j+ 1]
Forward: [i][j+ 1]
Upwards: [i-1][j+ 1]
Your aim is to navigate to the last column (len(matrix[0]) - 1), preferably in the lowest row possible (i).
The Solution: A Recursive Approach
To achieve this, you will use a recursive function that examines each movement option at each step. Let’s break down the solution step-by-step.
1. Define the Recursive Function
The first step is to define our recursive function, jumpFromOnes(matrix, i, j), which accepts the matrix and the current row and column indices.
2. Set Base Cases
At the core of recursion, base cases determine when the recursion should stop. In our case, these are:
If you reach the last row: if i == len(matrix) - 1.
If you reach the last column: if j == len(matrix[0]) - 1.
3. Recursion Logic
The function should check whether it's possible to move to the next three potential positions:
[[See Video to Reveal this Text or Code Snippet]]
4. Handling Dead Ends
If none of the movement options are available (when hitting a 1), the function should return a message indicating that it’s impossible to continue:
[[See Video to Reveal this Text or Code Snippet]]
Full Implementation
Here’s how the entire function looks, integrating all the parts discussed:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In this guide, we covered how to structure a recursive function in Python to navigate through a binary matrix filled with obstacles (1s) while seeking a path towards the last column. This tips not only help you solve matrix navigation problems but also strengthen your understanding of recursion in programming. You can adapt, modify, and leverage this logic to various applications where matrix navigation or obstacle avoidance is required.
Happy coding!
Видео Navigating A Matrix with Recursion: Overcoming Obstacles with Python канала vlogize
Комментарии отсутствуют
Информация о видео
7 апреля 2025 г. 1:51:17
00:01:53
Другие видео канала


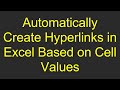
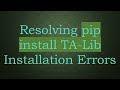
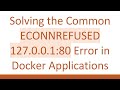




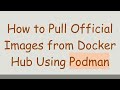
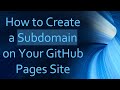

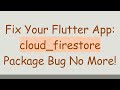
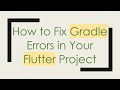
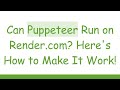
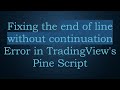
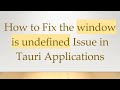
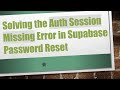


