Implementing Memory Allocation and Deallocation in C: A Linked List Approach
Discover how to implement memory allocation and deallocation in C using linked lists without malloc, along with a robust code example.
---
This video is based on the question https://stackoverflow.com/q/77949126/ asked by the user '22 22' ( https://stackoverflow.com/u/23348047/ ) and on the answer https://stackoverflow.com/a/77954854/ provided by the user 'Lundin' ( https://stackoverflow.com/u/584518/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Implementing memory allocation and deallocation
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Implementing Memory Allocation and Deallocation in C: A Linked List Approach
In C programming, memory management is crucial, especially when dealing with dynamic data structures like linked lists. A common challenge arises when one needs to implement memory allocation and deallocation without using the standard malloc function. In this guide, we will explore how to implement a memory management system using a linked list, focusing on allocating and reusing memory efficiently.
The Problem Statement
The objective is to create a linked list structure in C, where memory allocation occurs without using malloc. The requirements are:
The initial memory allocated must be 1MB.
Memory for each node should be allocated based on its size, returning the address of the new node.
A custom myfree function should allow reusing deleted nodes instead of freeing memory.
Understanding the Solution
Memory Management Structure
The solution consists of several components:
Memory Block: A fixed-size array that simulates available memory.
Linked List Nodes: Each node contains data and a pointer to the next node.
Memory Blocks Management: A structure to track which parts of the memory are free or allocated.
Code Breakdown
Let's break down the key components of the code implementation:
Define Memory Size:
[[See Video to Reveal this Text or Code Snippet]]
Node and Linked List Structures:
[[See Video to Reveal this Text or Code Snippet]]
Memory Management Structures:
[[See Video to Reveal this Text or Code Snippet]]
Memory Status Function:
This function helps monitor the current memory usage and availability:
[[See Video to Reveal this Text or Code Snippet]]
Custom Memory Allocation (mymalloc):
The custom allocation function checks the free memory list first and then allocates from the main memory block:
[[See Video to Reveal this Text or Code Snippet]]
Custom Memory Deallocation (myfree):
This function provides a way to reclaim deleted nodes so memory can be reused:
[[See Video to Reveal this Text or Code Snippet]]
Linked List Operations:
Functions to initialize the linked list, create nodes, append, delete nodes, and print the list.
Key Operations
The key functions for managing the linked list include:
appendNode(LinkedList* list, int data): Adds a new node with the specified data.
deleteNode(LinkedList* list, int data): Removes a node containing the specified data while reclaiming memory.
printList(LinkedList* list): Displays the current linked list.
Example Program Execution
Here’s a simplified main function showcasing how the linked list is manipulated by allowing the user to add, delete, and print nodes:
[[See Video to Reveal this Text or Code Snippet]]
Final Thoughts
Implementing memory allocation and deallocation through linked lists in C without the malloc function presents a unique challenge that can be efficiently tackled with proper understanding and structure. Instead of relying on dynamic memory allocation directly, we utilized a fixed memory pool and implemented our own allocation/deallocation logic.
By taking this approach, not only do we gain familiarity with pointers and memory management in C, but we also build a robust linked list implementation that can be adapted or used in various applications.
Conclusion
This blog has provided insight into how to tackle memory management using linked lists without using the conventional malloc function, addressing the challenge while demonstrating practical coding solutions. Remember, memory management is a critical aspect of C programming that deserves thorough understanding to avoid pitfalls like memory leaks and undefined behaviors.
Видео Implementing Memory Allocation and Deallocation in C: A Linked List Approach канала vlogize
Implementing memory allocation and deallocation
---
This video is based on the question https://stackoverflow.com/q/77949126/ asked by the user '22 22' ( https://stackoverflow.com/u/23348047/ ) and on the answer https://stackoverflow.com/a/77954854/ provided by the user 'Lundin' ( https://stackoverflow.com/u/584518/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Implementing memory allocation and deallocation
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Implementing Memory Allocation and Deallocation in C: A Linked List Approach
In C programming, memory management is crucial, especially when dealing with dynamic data structures like linked lists. A common challenge arises when one needs to implement memory allocation and deallocation without using the standard malloc function. In this guide, we will explore how to implement a memory management system using a linked list, focusing on allocating and reusing memory efficiently.
The Problem Statement
The objective is to create a linked list structure in C, where memory allocation occurs without using malloc. The requirements are:
The initial memory allocated must be 1MB.
Memory for each node should be allocated based on its size, returning the address of the new node.
A custom myfree function should allow reusing deleted nodes instead of freeing memory.
Understanding the Solution
Memory Management Structure
The solution consists of several components:
Memory Block: A fixed-size array that simulates available memory.
Linked List Nodes: Each node contains data and a pointer to the next node.
Memory Blocks Management: A structure to track which parts of the memory are free or allocated.
Code Breakdown
Let's break down the key components of the code implementation:
Define Memory Size:
[[See Video to Reveal this Text or Code Snippet]]
Node and Linked List Structures:
[[See Video to Reveal this Text or Code Snippet]]
Memory Management Structures:
[[See Video to Reveal this Text or Code Snippet]]
Memory Status Function:
This function helps monitor the current memory usage and availability:
[[See Video to Reveal this Text or Code Snippet]]
Custom Memory Allocation (mymalloc):
The custom allocation function checks the free memory list first and then allocates from the main memory block:
[[See Video to Reveal this Text or Code Snippet]]
Custom Memory Deallocation (myfree):
This function provides a way to reclaim deleted nodes so memory can be reused:
[[See Video to Reveal this Text or Code Snippet]]
Linked List Operations:
Functions to initialize the linked list, create nodes, append, delete nodes, and print the list.
Key Operations
The key functions for managing the linked list include:
appendNode(LinkedList* list, int data): Adds a new node with the specified data.
deleteNode(LinkedList* list, int data): Removes a node containing the specified data while reclaiming memory.
printList(LinkedList* list): Displays the current linked list.
Example Program Execution
Here’s a simplified main function showcasing how the linked list is manipulated by allowing the user to add, delete, and print nodes:
[[See Video to Reveal this Text or Code Snippet]]
Final Thoughts
Implementing memory allocation and deallocation through linked lists in C without the malloc function presents a unique challenge that can be efficiently tackled with proper understanding and structure. Instead of relying on dynamic memory allocation directly, we utilized a fixed memory pool and implemented our own allocation/deallocation logic.
By taking this approach, not only do we gain familiarity with pointers and memory management in C, but we also build a robust linked list implementation that can be adapted or used in various applications.
Conclusion
This blog has provided insight into how to tackle memory management using linked lists without using the conventional malloc function, addressing the challenge while demonstrating practical coding solutions. Remember, memory management is a critical aspect of C programming that deserves thorough understanding to avoid pitfalls like memory leaks and undefined behaviors.
Видео Implementing Memory Allocation and Deallocation in C: A Linked List Approach канала vlogize
Implementing memory allocation and deallocation
Показать
Комментарии отсутствуют
Информация о видео
5 апреля 2025 г. 23:57:56
00:02:23
Другие видео канала
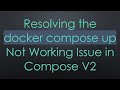


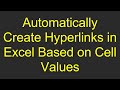

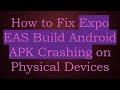
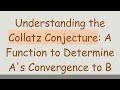

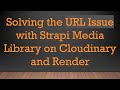
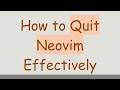

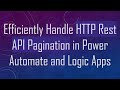
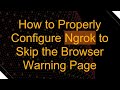


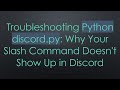

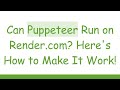
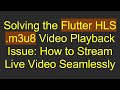
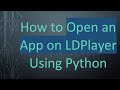
