A Guide to Socket.io Implementation: Solving Reconnection Issues
Learn how to properly implement `Socket.io` in your React application and troubleshoot common socket reconnection problems.
---
This video is based on the question https://stackoverflow.com/q/76337390/ asked by the user 'Novast071' ( https://stackoverflow.com/u/14553848/ ) and on the answer https://stackoverflow.com/a/76337910/ provided by the user 'Abubakr' ( https://stackoverflow.com/u/18149805/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: correct socket.io implementation
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
A Guide to Socket.io Implementation: Solving Reconnection Issues
When working on a project that involves real-time features like showing online friends, integrating Socket.io can be a game changer. However, you may run into issues, particularly with how sockets connect and disconnect upon state changes or user actions. If you've found yourself facing issues with unwanted reconnections — especially during profile updates or similar processes — you're not alone.
In this guide, we’ll dive into the common problems associated with Socket.io reconnections and explore a structured solution that could enhance the stability of your socket implementation.
Understanding the Problem
One of the frequent pitfalls developers encounter while using Socket.io in their React applications is that the socket disconnects and reconnects unexpectedly. This typically happens when the component re-renders due to changing dependencies, such as state updates or fetched data changes. This behavior, while sometimes expected, can be frustrating when you're trying to maintain a constant WebSocket connection during less critical updates (like profile changes).
Key Indicators of the Issue:
Socket Disconnecting: The socket disconnects whenever there is a re-render.
Socket Not Reconnecting: After disconnection, the socket fails to reconnect unless the page is refreshed.
Excessive Socket Creation: Each change triggers a new socket instance instead of reusing the existing one.
Implementing a Better Structure
To combat the issues above, let's break down the implementation into a clearer, more organized structure. Here’s a structured way to handle socket connections effectively:
Step 1: Create a Socket Context
By utilizing a context, you can provide the socket instance throughout your application without having it tied directly to any specific component that might re-render frequently.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Socket in Components
Now that we have a dedicated context for our socket connection, we can consume it easily in our components without worrying about reconnections on every state update.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Socket Emission in Event Handlers
You can now use socket.emit("signal", data) in your event handlers for actions like friend requests or profile updates without worrying about affecting the connection stability.
Conclusion
By separating the socket logic from other state management in your application, you can effectively eliminate unnecessary reconnections and maintain a stable WebSocket connection. This reduces the complexities associated with managing online statuses or friend notifications.
Embrace this structured approach with Socket.io and see how it enhances your real-time application experience. Happy coding!
Видео A Guide to Socket.io Implementation: Solving Reconnection Issues канала vlogize
correct socket.io implementation, javascript, reactjs, websocket, socket.io
---
This video is based on the question https://stackoverflow.com/q/76337390/ asked by the user 'Novast071' ( https://stackoverflow.com/u/14553848/ ) and on the answer https://stackoverflow.com/a/76337910/ provided by the user 'Abubakr' ( https://stackoverflow.com/u/18149805/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: correct socket.io implementation
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
A Guide to Socket.io Implementation: Solving Reconnection Issues
When working on a project that involves real-time features like showing online friends, integrating Socket.io can be a game changer. However, you may run into issues, particularly with how sockets connect and disconnect upon state changes or user actions. If you've found yourself facing issues with unwanted reconnections — especially during profile updates or similar processes — you're not alone.
In this guide, we’ll dive into the common problems associated with Socket.io reconnections and explore a structured solution that could enhance the stability of your socket implementation.
Understanding the Problem
One of the frequent pitfalls developers encounter while using Socket.io in their React applications is that the socket disconnects and reconnects unexpectedly. This typically happens when the component re-renders due to changing dependencies, such as state updates or fetched data changes. This behavior, while sometimes expected, can be frustrating when you're trying to maintain a constant WebSocket connection during less critical updates (like profile changes).
Key Indicators of the Issue:
Socket Disconnecting: The socket disconnects whenever there is a re-render.
Socket Not Reconnecting: After disconnection, the socket fails to reconnect unless the page is refreshed.
Excessive Socket Creation: Each change triggers a new socket instance instead of reusing the existing one.
Implementing a Better Structure
To combat the issues above, let's break down the implementation into a clearer, more organized structure. Here’s a structured way to handle socket connections effectively:
Step 1: Create a Socket Context
By utilizing a context, you can provide the socket instance throughout your application without having it tied directly to any specific component that might re-render frequently.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Socket in Components
Now that we have a dedicated context for our socket connection, we can consume it easily in our components without worrying about reconnections on every state update.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Socket Emission in Event Handlers
You can now use socket.emit("signal", data) in your event handlers for actions like friend requests or profile updates without worrying about affecting the connection stability.
Conclusion
By separating the socket logic from other state management in your application, you can effectively eliminate unnecessary reconnections and maintain a stable WebSocket connection. This reduces the complexities associated with managing online statuses or friend notifications.
Embrace this structured approach with Socket.io and see how it enhances your real-time application experience. Happy coding!
Видео A Guide to Socket.io Implementation: Solving Reconnection Issues канала vlogize
correct socket.io implementation, javascript, reactjs, websocket, socket.io
Показать
Комментарии отсутствуют
Информация о видео
Вчера, 11:35:22
00:02:21
Другие видео канала

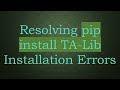
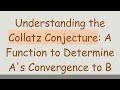


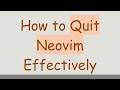
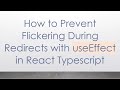

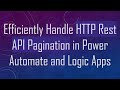
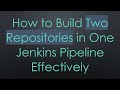

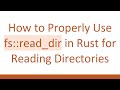

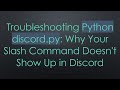
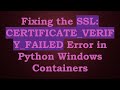
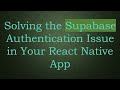

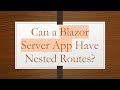
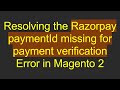
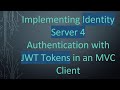
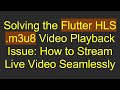