Managing Version Numbers in Blazor Apps
Discover how to set, read, and manage version numbers in Blazor apps effectively. Learn tips for auto-incrementing version numbers on GitHub.
---
This video is based on the question https://stackoverflow.com/q/77565930/ asked by the user 'David Thielen' ( https://stackoverflow.com/u/509627/ ) and on the answer https://stackoverflow.com/a/77566194/ provided by the user 'LoneSpawn' ( https://stackoverflow.com/u/3786270/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Is there a version number for Blazor apps?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Managing Version Numbers in Blazor Apps: A Comprehensive Guide
When developing applications, it's essential to keep track of changes, improvements, and releases. In traditional Win Forms applications, maintaining a version number was quite straightforward through an AssemblyInfo properties file. With the rise of Blazor apps, many developers wonder how to implement similar versioning.
This guide will address the question of whether there is a version number for Blazor apps, along with providing practical steps for implementation.
The Problem: Versioning in Blazor Apps
Just like Win Forms, Blazor applications benefit from versioning. It provides a clear reference for users and developers alike. The following points outline the key concerns regarding versioning in Blazor apps:
Where to define the version number?
How to read the version number programmatically?
Is there a way to auto-increment the version number on GitHub?
Let’s delve into each of these questions to uncover the best practices for managing version numbers in your Blazor applications.
Solution Breakdown
1. Where to Define the Version Number?
In Blazor applications, you can specify the version number directly in your project’s .csproj file. Follow these steps:
Open your .csproj file, which is located in the root directory of your Blazor project.
Add a <Version> tag within a <PropertyGroup>.
Here’s an example of how it should look:
[[See Video to Reveal this Text or Code Snippet]]
2. How to Read the Version Number Programmatically?
To check the version number programmatically, you can create an extension method that retrieves the version from the assembly. This helps you access the version number easily wherever needed in your code.
Here’s how you can implement it:
Create a static class for the extension method:
[[See Video to Reveal this Text or Code Snippet]]
To read the assembly's version, use the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
By using this approach, you can easily retrieve the version number of your Blazor application at runtime.
3. Is There an Easy Way to Tell GitHub to Auto-Increment It?
This is where things can get a bit tricky. At the moment, there isn't a straightforward built-in mechanism to auto-increment your version number directly from GitHub during publishing. Developers typically require additional tools or scripts to manage this process.
However, there are workarounds:
GitHub Actions: You might consider setting up a GitHub Action that modifies your .csproj file to increment the version number automatically upon each release.
Semantic Versioning: Enforce a pattern for your versioning system, allowing team members to manually update it following a pre-agreed system each time there’s a significant change.
Conclusion
Proper version management is crucial for any software application, including Blazor apps. By defining where to set the version number, learning how to read it programmatically, and exploring potential ways to automate its incrementation, you can effectively manage version numbers in your Blazor projects.
If you have further queries about managing version numbers or other aspects of your Blazor applications, don't hesitate to reach out or explore community forums. Happy coding!
Видео Managing Version Numbers in Blazor Apps канала vlogize
Is there a version number for Blazor apps?, blazor, blazor server side, github
---
This video is based on the question https://stackoverflow.com/q/77565930/ asked by the user 'David Thielen' ( https://stackoverflow.com/u/509627/ ) and on the answer https://stackoverflow.com/a/77566194/ provided by the user 'LoneSpawn' ( https://stackoverflow.com/u/3786270/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Is there a version number for Blazor apps?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Managing Version Numbers in Blazor Apps: A Comprehensive Guide
When developing applications, it's essential to keep track of changes, improvements, and releases. In traditional Win Forms applications, maintaining a version number was quite straightforward through an AssemblyInfo properties file. With the rise of Blazor apps, many developers wonder how to implement similar versioning.
This guide will address the question of whether there is a version number for Blazor apps, along with providing practical steps for implementation.
The Problem: Versioning in Blazor Apps
Just like Win Forms, Blazor applications benefit from versioning. It provides a clear reference for users and developers alike. The following points outline the key concerns regarding versioning in Blazor apps:
Where to define the version number?
How to read the version number programmatically?
Is there a way to auto-increment the version number on GitHub?
Let’s delve into each of these questions to uncover the best practices for managing version numbers in your Blazor applications.
Solution Breakdown
1. Where to Define the Version Number?
In Blazor applications, you can specify the version number directly in your project’s .csproj file. Follow these steps:
Open your .csproj file, which is located in the root directory of your Blazor project.
Add a <Version> tag within a <PropertyGroup>.
Here’s an example of how it should look:
[[See Video to Reveal this Text or Code Snippet]]
2. How to Read the Version Number Programmatically?
To check the version number programmatically, you can create an extension method that retrieves the version from the assembly. This helps you access the version number easily wherever needed in your code.
Here’s how you can implement it:
Create a static class for the extension method:
[[See Video to Reveal this Text or Code Snippet]]
To read the assembly's version, use the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
By using this approach, you can easily retrieve the version number of your Blazor application at runtime.
3. Is There an Easy Way to Tell GitHub to Auto-Increment It?
This is where things can get a bit tricky. At the moment, there isn't a straightforward built-in mechanism to auto-increment your version number directly from GitHub during publishing. Developers typically require additional tools or scripts to manage this process.
However, there are workarounds:
GitHub Actions: You might consider setting up a GitHub Action that modifies your .csproj file to increment the version number automatically upon each release.
Semantic Versioning: Enforce a pattern for your versioning system, allowing team members to manually update it following a pre-agreed system each time there’s a significant change.
Conclusion
Proper version management is crucial for any software application, including Blazor apps. By defining where to set the version number, learning how to read it programmatically, and exploring potential ways to automate its incrementation, you can effectively manage version numbers in your Blazor projects.
If you have further queries about managing version numbers or other aspects of your Blazor applications, don't hesitate to reach out or explore community forums. Happy coding!
Видео Managing Version Numbers in Blazor Apps канала vlogize
Is there a version number for Blazor apps?, blazor, blazor server side, github
Показать
Комментарии отсутствуют
Информация о видео
3 марта 2025 г. 11:16:45
00:01:49
Другие видео канала
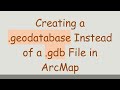
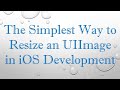

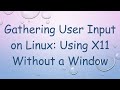
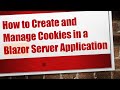

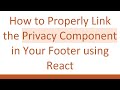


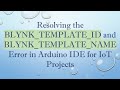
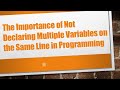


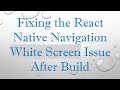
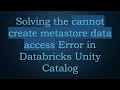
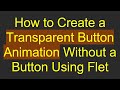

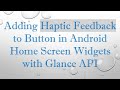
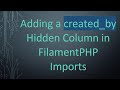
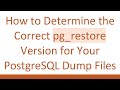