Understanding Python's append Method: Fixing the Zero Sequence Issue
Learn how to use the `append` method correctly in Python to find the longest sequence of integers whose sum is zero. This guide provides a clear explanation and solution to a common problem.
---
This video is based on the question https://stackoverflow.com/q/76799022/ asked by the user 'juangalicia' ( https://stackoverflow.com/u/14355045/ ) and on the answer https://stackoverflow.com/a/76799121/ provided by the user 'Nonlinear' ( https://stackoverflow.com/u/17800066/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: what's going with the append method here in python
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python's append Method: Fixing the Zero Sequence Issue
In Python, the append method is widely used to add elements to lists. However, it can lead to unexpected behavior, especially when dealing with mutable objects, like lists, within loops. If you’ve ever faced a problem where your program seems to yield incorrect results due to how it manages lists, you’re not alone. Today, we're going to explore one such case—finding the longest sequence of integers that sums to zero using the append method correctly.
The Problem at Hand
Consider the following function designed to find the longest subsequence of integers that sums to zero:
[[See Video to Reveal this Text or Code Snippet]]
When the function is called with the input arr = [1, 2, -3, 7, 8, -16], you might expect the output to simply be [[1, 2, -3]], but instead, you receive [[[1, 2, -3, 7, 8, -16]]]. This unexpected result can be traced back to how you're using the append method and managing the sequence variable.
Understanding the Issue
The core of the issue lies in the way lists reference data in Python. When you append sequence to answers, you're not storing a snapshot of sequence at that moment. Instead, you're storing a reference to the same list. This means that if you later modify sequence, that change is also reflected in the list stored in answers.
In the original function:
sequence is modified during each iteration of the loop.
When the condition if sum(sequence) == 0 is satisfied, the current state of sequence is appended to answers.
However, sequence is later reset to an empty list before moving to the next iteration, meaning any future changes are also reflected in the already appended lists.
The Solution
To resolve the problem and ensure that answers contains distinct lists, you should append a copy of sequence instead of a reference. This can be accomplished by changing the line that appends sequence in the loop. Here’s the modified function:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Change answers.append(sequence) to answers.append(sequence.copy()).
Benefits of Using sequence.copy():
Preservation: This creates a new list that has the same elements as sequence at that moment and appends it to answers.
Isolation: Subsequent modifications to sequence do not affect the already recorded subsequences.
Conclusion
By adjusting how we manage lists in Python, particularly with the append and copy methods, we can avoid unexpected behavior and ensure our functions provide the desired output. This technique is invaluable when working with mutable objects, especially within iterative constructs. If you're dealing with lists and want to preserve their state, remember to create a copy before appending!
Feel free to apply this enhanced understanding of the append method in your projects, and watch out for similar issues in your work!
Видео Understanding Python's append Method: Fixing the Zero Sequence Issue канала vlogize
---
This video is based on the question https://stackoverflow.com/q/76799022/ asked by the user 'juangalicia' ( https://stackoverflow.com/u/14355045/ ) and on the answer https://stackoverflow.com/a/76799121/ provided by the user 'Nonlinear' ( https://stackoverflow.com/u/17800066/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: what's going with the append method here in python
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python's append Method: Fixing the Zero Sequence Issue
In Python, the append method is widely used to add elements to lists. However, it can lead to unexpected behavior, especially when dealing with mutable objects, like lists, within loops. If you’ve ever faced a problem where your program seems to yield incorrect results due to how it manages lists, you’re not alone. Today, we're going to explore one such case—finding the longest sequence of integers that sums to zero using the append method correctly.
The Problem at Hand
Consider the following function designed to find the longest subsequence of integers that sums to zero:
[[See Video to Reveal this Text or Code Snippet]]
When the function is called with the input arr = [1, 2, -3, 7, 8, -16], you might expect the output to simply be [[1, 2, -3]], but instead, you receive [[[1, 2, -3, 7, 8, -16]]]. This unexpected result can be traced back to how you're using the append method and managing the sequence variable.
Understanding the Issue
The core of the issue lies in the way lists reference data in Python. When you append sequence to answers, you're not storing a snapshot of sequence at that moment. Instead, you're storing a reference to the same list. This means that if you later modify sequence, that change is also reflected in the list stored in answers.
In the original function:
sequence is modified during each iteration of the loop.
When the condition if sum(sequence) == 0 is satisfied, the current state of sequence is appended to answers.
However, sequence is later reset to an empty list before moving to the next iteration, meaning any future changes are also reflected in the already appended lists.
The Solution
To resolve the problem and ensure that answers contains distinct lists, you should append a copy of sequence instead of a reference. This can be accomplished by changing the line that appends sequence in the loop. Here’s the modified function:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Change answers.append(sequence) to answers.append(sequence.copy()).
Benefits of Using sequence.copy():
Preservation: This creates a new list that has the same elements as sequence at that moment and appends it to answers.
Isolation: Subsequent modifications to sequence do not affect the already recorded subsequences.
Conclusion
By adjusting how we manage lists in Python, particularly with the append and copy methods, we can avoid unexpected behavior and ensure our functions provide the desired output. This technique is invaluable when working with mutable objects, especially within iterative constructs. If you're dealing with lists and want to preserve their state, remember to create a copy before appending!
Feel free to apply this enhanced understanding of the append method in your projects, and watch out for similar issues in your work!
Видео Understanding Python's append Method: Fixing the Zero Sequence Issue канала vlogize
Комментарии отсутствуют
Информация о видео
8 апреля 2025 г. 8:56:00
00:01:42
Другие видео канала




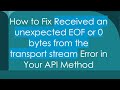
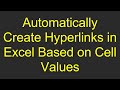

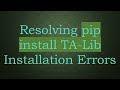
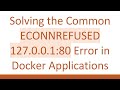

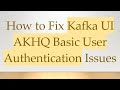
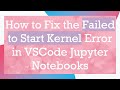

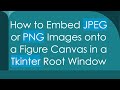

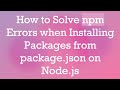
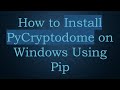
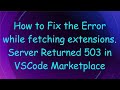
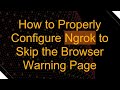
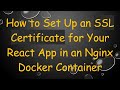
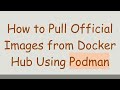