How to Access useParams with Redux Selectors in React
Learn how to effectively access `useParams` values within Redux selectors using `mapStateToProps` and React-Redux hooks.
---
This video is based on the question https://stackoverflow.com/q/70473730/ asked by the user 'Mr.Z' ( https://stackoverflow.com/u/14180429/ ) and on the answer https://stackoverflow.com/a/70473862/ provided by the user 'HDM91' ( https://stackoverflow.com/u/1861144/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I access useParams value inside redux selector as ownProps
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Access useParams with Redux Selectors in React
When developing a React application, particularly when using React Router and Redux, you might encounter scenarios where you need to access the route parameters. Specifically, you might want to use the useParams hook from React Router to pass a parameter value into a Redux selector. This can sometimes be tricky, especially when you're trying to use useParams() inside the mapStateToProps function. Let's break down this problem and explore potential solutions.
The Problem
You might be working with a scenario similar to the following:
[[See Video to Reveal this Text or Code Snippet]]
Here, you want to access the parameters retrieved by the useParams hook and pass them to the selectCollection function. However, you might find that ownProps doesn't recognize the useParams in the expected way, leading to undefined values. This often happens because of scope issues or incorrect passing of props.
Solution Approaches
There are two effective approaches to handle this scenario: creating a top-level component or utilizing React-Redux hooks. Let's explore each method in detail.
1. Create a Top-Level Component
One approach is to create a top-level component that can handle the routing parameters. This component will use the useParams hook to get the parameters and then pass them down to your CollectionPage component. Here's how that looks:
[[See Video to Reveal this Text or Code Snippet]]
Key Points of This Approach:
The TopLevelCollectionPage component acts as a mediator, effectively passing the route parameters into CollectionPage as props.
This allows you to access and use those params directly in mapStateToProps.
2. Using React-Redux Hooks
Another modern approach is to utilize the useSelector hook from React-Redux directly inside your CollectionPage. This eliminates the need for mapStateToProps and can simplify your state management within the component:
[[See Video to Reveal this Text or Code Snippet]]
Advantages of This Method:
Cleaner and more concise: You no longer need to define mapStateToProps which can result in easier maintenance.
Directly accessing the state allows for more straightforward logic flow within the component.
Conclusion
Incorporating useParams into a Redux selector can be a bit challenging due to the way React components manage their scope and props. However, by creating a top-level component or directly using React-Redux hooks, you can effectively access the necessary parameters and streamline your application. Choose the approach that best fits your project structure and coding style.
Feel free to adapt these strategies to your needs, and happy coding!
Видео How to Access useParams with Redux Selectors in React канала vlogize
---
This video is based on the question https://stackoverflow.com/q/70473730/ asked by the user 'Mr.Z' ( https://stackoverflow.com/u/14180429/ ) and on the answer https://stackoverflow.com/a/70473862/ provided by the user 'HDM91' ( https://stackoverflow.com/u/1861144/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I access useParams value inside redux selector as ownProps
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Access useParams with Redux Selectors in React
When developing a React application, particularly when using React Router and Redux, you might encounter scenarios where you need to access the route parameters. Specifically, you might want to use the useParams hook from React Router to pass a parameter value into a Redux selector. This can sometimes be tricky, especially when you're trying to use useParams() inside the mapStateToProps function. Let's break down this problem and explore potential solutions.
The Problem
You might be working with a scenario similar to the following:
[[See Video to Reveal this Text or Code Snippet]]
Here, you want to access the parameters retrieved by the useParams hook and pass them to the selectCollection function. However, you might find that ownProps doesn't recognize the useParams in the expected way, leading to undefined values. This often happens because of scope issues or incorrect passing of props.
Solution Approaches
There are two effective approaches to handle this scenario: creating a top-level component or utilizing React-Redux hooks. Let's explore each method in detail.
1. Create a Top-Level Component
One approach is to create a top-level component that can handle the routing parameters. This component will use the useParams hook to get the parameters and then pass them down to your CollectionPage component. Here's how that looks:
[[See Video to Reveal this Text or Code Snippet]]
Key Points of This Approach:
The TopLevelCollectionPage component acts as a mediator, effectively passing the route parameters into CollectionPage as props.
This allows you to access and use those params directly in mapStateToProps.
2. Using React-Redux Hooks
Another modern approach is to utilize the useSelector hook from React-Redux directly inside your CollectionPage. This eliminates the need for mapStateToProps and can simplify your state management within the component:
[[See Video to Reveal this Text or Code Snippet]]
Advantages of This Method:
Cleaner and more concise: You no longer need to define mapStateToProps which can result in easier maintenance.
Directly accessing the state allows for more straightforward logic flow within the component.
Conclusion
Incorporating useParams into a Redux selector can be a bit challenging due to the way React components manage their scope and props. However, by creating a top-level component or directly using React-Redux hooks, you can effectively access the necessary parameters and streamline your application. Choose the approach that best fits your project structure and coding style.
Feel free to adapt these strategies to your needs, and happy coding!
Видео How to Access useParams with Redux Selectors in React канала vlogize
Комментарии отсутствуют
Информация о видео
29 марта 2025 г. 18:05:10
00:01:59
Другие видео канала





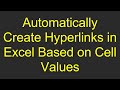

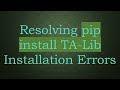
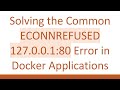

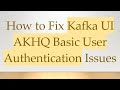
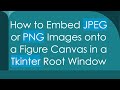


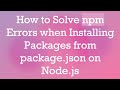
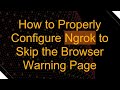
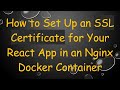
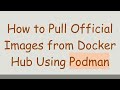
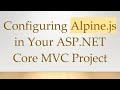

