How to Delete All Recordings in Rails
Learn how to create an endpoint that can `delete all products` in a Ruby on Rails application. This guide will provide a clear solution and an easy-to-follow implementation process.
---
This video is based on the question https://stackoverflow.com/q/70022380/ asked by the user 'Дмитро Горецький' ( https://stackoverflow.com/u/14974783/ ) and on the answer https://stackoverflow.com/a/70022445/ provided by the user 'Sergio Tulentsev' ( https://stackoverflow.com/u/125816/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to delete all recordings in rails?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Delete All Recordings in Rails: A Step-by-Step Guide
In the world of web applications, managing the data stored in your database is crucial. Sometimes, you may find the need to delete multiple records — perhaps to clean up outdated information or reset the application's state. In Ruby on Rails, while deleting individual records can be done easily, creating an endpoint to delete all records requires a bit more setup.
In this guide, we'll tackle the problem of creating an endpoint in your Rails application that allows you to delete all products from your storage. We'll break down the problem and walk through the implementation step by step.
The Challenge: Deleting All Products
You currently have an endpoint that deletes a single product based on its ID. However, the need arises to have a more robust solution: an endpoint that will delete all products in one action.
Your current destroy method in the Products Controller looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
To enable bulk deletions, we need to create a custom action in the controller and define the corresponding route.
Step-by-Step Solution
Step 1: Update the Routes
First, you'll need to define a new route in your routes.rb file for the custom delete action. Here's how to do it:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create the Custom Action in the Controller
Next, you should implement the delete_all action in your ProductsController. This action will handle the deletion of all product records.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Adding the Button to Trigger Deletion
Now that you have the backend set up, it's time to add a button in your index.html.erb file that users can click to delete all products.
Add the following line to create a link that triggers a POST request to your new endpoint:
[[See Video to Reveal this Text or Code Snippet]]
This code creates a button labeled "Destroy All," which, when clicked, will prompt the user for confirmation before sending a POST request to the /products/delete_all route.
Conclusion
With these three steps, you've successfully implemented a custom action in your Rails application to delete all products at once. This approach allows for efficient bulk deletions and keeps your application's data management streamlined.
Key Points to Remember
Custom Route: You must explicitly define a custom route for non-standard REST actions.
Controller Action: Create a specific method in your controller to handle the bulk delete logic.
User Interface: Provide a clear button that guides users to execute the action with confirmation.
Implementing this functionality will greatly enhance the management features of your Rails application, providing users with a powerful tool for maintaining their products.
Now you’re ready to clean up your application easily!
Видео How to Delete All Recordings in Rails канала vlogize
---
This video is based on the question https://stackoverflow.com/q/70022380/ asked by the user 'Дмитро Горецький' ( https://stackoverflow.com/u/14974783/ ) and on the answer https://stackoverflow.com/a/70022445/ provided by the user 'Sergio Tulentsev' ( https://stackoverflow.com/u/125816/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to delete all recordings in rails?
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Delete All Recordings in Rails: A Step-by-Step Guide
In the world of web applications, managing the data stored in your database is crucial. Sometimes, you may find the need to delete multiple records — perhaps to clean up outdated information or reset the application's state. In Ruby on Rails, while deleting individual records can be done easily, creating an endpoint to delete all records requires a bit more setup.
In this guide, we'll tackle the problem of creating an endpoint in your Rails application that allows you to delete all products from your storage. We'll break down the problem and walk through the implementation step by step.
The Challenge: Deleting All Products
You currently have an endpoint that deletes a single product based on its ID. However, the need arises to have a more robust solution: an endpoint that will delete all products in one action.
Your current destroy method in the Products Controller looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
To enable bulk deletions, we need to create a custom action in the controller and define the corresponding route.
Step-by-Step Solution
Step 1: Update the Routes
First, you'll need to define a new route in your routes.rb file for the custom delete action. Here's how to do it:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create the Custom Action in the Controller
Next, you should implement the delete_all action in your ProductsController. This action will handle the deletion of all product records.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Adding the Button to Trigger Deletion
Now that you have the backend set up, it's time to add a button in your index.html.erb file that users can click to delete all products.
Add the following line to create a link that triggers a POST request to your new endpoint:
[[See Video to Reveal this Text or Code Snippet]]
This code creates a button labeled "Destroy All," which, when clicked, will prompt the user for confirmation before sending a POST request to the /products/delete_all route.
Conclusion
With these three steps, you've successfully implemented a custom action in your Rails application to delete all products at once. This approach allows for efficient bulk deletions and keeps your application's data management streamlined.
Key Points to Remember
Custom Route: You must explicitly define a custom route for non-standard REST actions.
Controller Action: Create a specific method in your controller to handle the bulk delete logic.
User Interface: Provide a clear button that guides users to execute the action with confirmation.
Implementing this functionality will greatly enhance the management features of your Rails application, providing users with a powerful tool for maintaining their products.
Now you’re ready to clean up your application easily!
Видео How to Delete All Recordings in Rails канала vlogize
Комментарии отсутствуют
Информация о видео
17 апреля 2025 г. 19:27:53
00:01:42
Другие видео канала





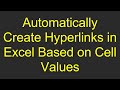
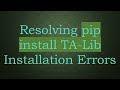
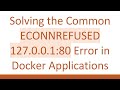

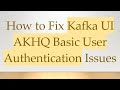


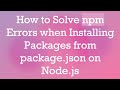
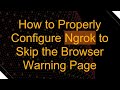
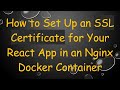
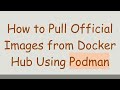


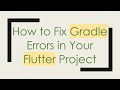
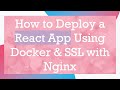
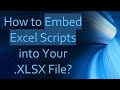