Resolving realloc Failures in C: A Guide to Handling Pointer Arrays
Learn how to fix `realloc()` failures in C when managing dynamic arrays of pointers with this comprehensive guide. Perfect for tackling memory management issues effectively!
---
This video is based on the question https://stackoverflow.com/q/69647063/ asked by the user 'octowaddle' ( https://stackoverflow.com/u/15140026/ ) and on the answer https://stackoverflow.com/a/69647133/ provided by the user 'dbush' ( https://stackoverflow.com/u/1687119/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: realloc fails with pointer array
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving realloc Failures in C: A Guide to Handling Pointer Arrays
Memory management in C can be tricky, especially when using dynamic memory allocation functions like malloc and realloc. Many developers encounter issues, often leading to frustrating crashes. One common problem arises when trying to resize an array of pointers, particularly with the realloc function. In this guide, we will explore a specific instance of this problem and break down the solution, step by step.
The Problem: Crashing Code Due to Improper Memory Management
Consider the following code snippet that attempts to create and resize an array of pointers to an arbitrary type:
[[See Video to Reveal this Text or Code Snippet]]
This code runs smoothly for the first four iterations, but during the fifth iteration, it crashes with the error message:
[[See Video to Reveal this Text or Code Snippet]]
After examining a plethora of discussions regarding the invalid next size error, it becomes clear that something basic is being overlooked.
The Cause of the Issue: Insufficient Memory Allocation
The critical mistake made here lies in how memory is being allocated for the data array during resizing. When using realloc, you have to consider the size of the data type you are working with. Specifically, the line causing the crash is:
[[See Video to Reveal this Text or Code Snippet]]
This instructs realloc to allocate ++size bytes. This means:
On the first iteration: 1 byte allocated
On the second iteration: 2 bytes allocated
On the third iteration: 3 bytes allocated
And so on...
This does not account for the actual size needed to store the pointers—it's far too little, leading to writing past the allocated memory and triggering undefined behavior.
The Solution: Correcting Memory Allocation
To fix this problem, you must multiply the number of elements by the size of each pointer to ensure enough memory is allocated. The corrected line should be:
[[See Video to Reveal this Text or Code Snippet]]
This change assures that enough space is allocated to accommodate all pointers within the data array.
Additional Fixes: Correcting Format Specifiers
Another crucial point to note in the original code is the use of the wrong format specifier in the printf function. When printing values of type size_t, which is what i is being incremented as, you should use the %zu format specifier. Here's the updated line:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion: Learning from Mistakes
Dynamic memory management in C requires careful handling to prevent crashes and undefined behavior. In this case, the issues stemmed from allocating an insufficient number of bytes with realloc and using the wrong format specifier in output statements.
By ensuring proper memory allocation and employing the correct format specs, your application runs more smoothly. Remember to always multiply the number of elements by the element's size when using realloc, and use designated format specifiers for data types!
By keeping these considerations in mind, you can avoid many pitfalls associated with managing dynamic memory in C. Happy coding!
Видео Resolving realloc Failures in C: A Guide to Handling Pointer Arrays канала vlogize
realloc fails with pointer array, memory
---
This video is based on the question https://stackoverflow.com/q/69647063/ asked by the user 'octowaddle' ( https://stackoverflow.com/u/15140026/ ) and on the answer https://stackoverflow.com/a/69647133/ provided by the user 'dbush' ( https://stackoverflow.com/u/1687119/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: realloc fails with pointer array
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving realloc Failures in C: A Guide to Handling Pointer Arrays
Memory management in C can be tricky, especially when using dynamic memory allocation functions like malloc and realloc. Many developers encounter issues, often leading to frustrating crashes. One common problem arises when trying to resize an array of pointers, particularly with the realloc function. In this guide, we will explore a specific instance of this problem and break down the solution, step by step.
The Problem: Crashing Code Due to Improper Memory Management
Consider the following code snippet that attempts to create and resize an array of pointers to an arbitrary type:
[[See Video to Reveal this Text or Code Snippet]]
This code runs smoothly for the first four iterations, but during the fifth iteration, it crashes with the error message:
[[See Video to Reveal this Text or Code Snippet]]
After examining a plethora of discussions regarding the invalid next size error, it becomes clear that something basic is being overlooked.
The Cause of the Issue: Insufficient Memory Allocation
The critical mistake made here lies in how memory is being allocated for the data array during resizing. When using realloc, you have to consider the size of the data type you are working with. Specifically, the line causing the crash is:
[[See Video to Reveal this Text or Code Snippet]]
This instructs realloc to allocate ++size bytes. This means:
On the first iteration: 1 byte allocated
On the second iteration: 2 bytes allocated
On the third iteration: 3 bytes allocated
And so on...
This does not account for the actual size needed to store the pointers—it's far too little, leading to writing past the allocated memory and triggering undefined behavior.
The Solution: Correcting Memory Allocation
To fix this problem, you must multiply the number of elements by the size of each pointer to ensure enough memory is allocated. The corrected line should be:
[[See Video to Reveal this Text or Code Snippet]]
This change assures that enough space is allocated to accommodate all pointers within the data array.
Additional Fixes: Correcting Format Specifiers
Another crucial point to note in the original code is the use of the wrong format specifier in the printf function. When printing values of type size_t, which is what i is being incremented as, you should use the %zu format specifier. Here's the updated line:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion: Learning from Mistakes
Dynamic memory management in C requires careful handling to prevent crashes and undefined behavior. In this case, the issues stemmed from allocating an insufficient number of bytes with realloc and using the wrong format specifier in output statements.
By ensuring proper memory allocation and employing the correct format specs, your application runs more smoothly. Remember to always multiply the number of elements by the element's size when using realloc, and use designated format specifiers for data types!
By keeping these considerations in mind, you can avoid many pitfalls associated with managing dynamic memory in C. Happy coding!
Видео Resolving realloc Failures in C: A Guide to Handling Pointer Arrays канала vlogize
realloc fails with pointer array, memory
Показать
Комментарии отсутствуют
Информация о видео
4 апреля 2025 г. 9:27:53
00:01:47
Другие видео канала


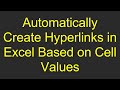
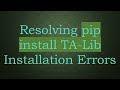
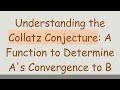


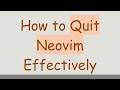

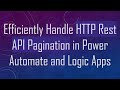
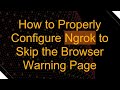


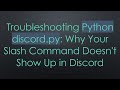

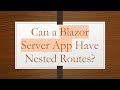
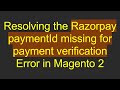
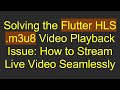
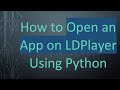

