How to build your own Wolfram Alpha — Math Equation Visualization with Tkinter and wolframalpha API
Hello Everyone! My name is Andrew Fung, in this video, I will be showing you how to create your own Math Equation Visualiser using python’s Tkinter Library and Wolfram Alpha API.
#wolframalpha #tkinter #datavisualization #python #replit
Installation and Setup!
Replit: https://replit.com/
Wolfram Alpha: https://www.wolframalpha.com
Wolfram Alpha API: https://products.wolframalpha.com/api/
Documentation: https://products.wolframalpha.com/simple-api/documentation/
Tkinter documentation: https://docs.python.org/3/library/tkinter.html
Source code for this project:
https://github.com/Andrew-FungKinHo/YouTube/blob/main/wolframAlpha.py
Check out my Github!
https://github.com/Andrew-FungKinHo
How I make my YouTube videos:
⌨️ Keyboard - Nuphy Air75 Mechanical Keyboard - https://amzn.to/3Xu4PD3
🎙 Microphone - MAONO A04 Professional Podcaster USB Microphone - https://amzn.to/3k8ocD5
🖱 Mouse - Microsoft Bluetooth Ergonomic Mouse - https://amzn.to/3CHhdHJ
🔌 Accessories - Laptop Docking Station for MacBook Pro - https://amzn.to/3CHi5Mv
Timestamps
0:00 | Intro
1:56 | Data Fetching from API
9:10 | User Interface with Tkinter
22:28 | Project improvement
24:02 | Out tro
Full code:
———————————————————————————————
import wolframalpha
import base64
import tkinter as tk
from urllib.request import urlopen
API_KEY = ‘INSERT YOUR API KEY HERE’
client = wolframalpha.Client(API_KEY)
def math():
equation = entry1.get()
res = client.query(equation.strip(),params=(("format", "image,plaintext"),))
data = {}
for p in res.pods:
for s in p.subpods:
if s.img.alt.lower() == "root plot":
data['rootPlot'] = s.img.src
elif s.img.alt.lower() == "number line":
data['numberLine'] = s.img.src
data['results'] = [i.texts for i in list(res.results)][0]
image1_url = data['rootPlot']
image2_url = data['numberLine']
image1_byt = urlopen(image1_url).read()
image1_b64 = base64.encodebytes(image1_byt)
photo1 = tk.PhotoImage(data=image1_b64)
image2_byt = urlopen(image2_url).read()
image2_b64 = base64.encodebytes(image2_byt)
photo2 = tk.PhotoImage(data=image2_b64)
canvas.photo1 = photo1
canvas.photo2 = photo2
canvas.create_image(10,30, image=canvas.photo1, anchor='nw')
canvas.create_text(175,40, fill='darkblue',font='Arial 10',text='Root Plot')
canvas.create_image(10,240, image=canvas.photo2, anchor='nw')
canvas.create_text(175,230, fill='darkblue',font='Arial 10',text='Number Line')
canvas.create_text(175,320,fill='darkblue',font='Arial 15',text=data['results'])
root = tk.Tk()
root.title("Math Visualization")
w = 500
h = 500
x = 50
y = 100
root.geometry("%dx%d+%d+%d" % (w,h,x,y))
canvas = tk.Canvas(bg='white')
canvas.config(height=350)
canvas.pack()
# entry
entry1 = tk.Entry(root)
canvas.create_window(150,15,window=entry1)
# submit button
submit = tk.Button(text="Solve",command=math,height=1, width=3)
canvas.create_window(280,15,window=submit)
root.mainloop()
———————————————————————————————
Feel free to drop a like and comment if you enjoy and video and let me know if you want me to do other types of programming videos ;) !!!
Видео How to build your own Wolfram Alpha — Math Equation Visualization with Tkinter and wolframalpha API канала Andrew Fung
#wolframalpha #tkinter #datavisualization #python #replit
Installation and Setup!
Replit: https://replit.com/
Wolfram Alpha: https://www.wolframalpha.com
Wolfram Alpha API: https://products.wolframalpha.com/api/
Documentation: https://products.wolframalpha.com/simple-api/documentation/
Tkinter documentation: https://docs.python.org/3/library/tkinter.html
Source code for this project:
https://github.com/Andrew-FungKinHo/YouTube/blob/main/wolframAlpha.py
Check out my Github!
https://github.com/Andrew-FungKinHo
How I make my YouTube videos:
⌨️ Keyboard - Nuphy Air75 Mechanical Keyboard - https://amzn.to/3Xu4PD3
🎙 Microphone - MAONO A04 Professional Podcaster USB Microphone - https://amzn.to/3k8ocD5
🖱 Mouse - Microsoft Bluetooth Ergonomic Mouse - https://amzn.to/3CHhdHJ
🔌 Accessories - Laptop Docking Station for MacBook Pro - https://amzn.to/3CHi5Mv
Timestamps
0:00 | Intro
1:56 | Data Fetching from API
9:10 | User Interface with Tkinter
22:28 | Project improvement
24:02 | Out tro
Full code:
———————————————————————————————
import wolframalpha
import base64
import tkinter as tk
from urllib.request import urlopen
API_KEY = ‘INSERT YOUR API KEY HERE’
client = wolframalpha.Client(API_KEY)
def math():
equation = entry1.get()
res = client.query(equation.strip(),params=(("format", "image,plaintext"),))
data = {}
for p in res.pods:
for s in p.subpods:
if s.img.alt.lower() == "root plot":
data['rootPlot'] = s.img.src
elif s.img.alt.lower() == "number line":
data['numberLine'] = s.img.src
data['results'] = [i.texts for i in list(res.results)][0]
image1_url = data['rootPlot']
image2_url = data['numberLine']
image1_byt = urlopen(image1_url).read()
image1_b64 = base64.encodebytes(image1_byt)
photo1 = tk.PhotoImage(data=image1_b64)
image2_byt = urlopen(image2_url).read()
image2_b64 = base64.encodebytes(image2_byt)
photo2 = tk.PhotoImage(data=image2_b64)
canvas.photo1 = photo1
canvas.photo2 = photo2
canvas.create_image(10,30, image=canvas.photo1, anchor='nw')
canvas.create_text(175,40, fill='darkblue',font='Arial 10',text='Root Plot')
canvas.create_image(10,240, image=canvas.photo2, anchor='nw')
canvas.create_text(175,230, fill='darkblue',font='Arial 10',text='Number Line')
canvas.create_text(175,320,fill='darkblue',font='Arial 15',text=data['results'])
root = tk.Tk()
root.title("Math Visualization")
w = 500
h = 500
x = 50
y = 100
root.geometry("%dx%d+%d+%d" % (w,h,x,y))
canvas = tk.Canvas(bg='white')
canvas.config(height=350)
canvas.pack()
# entry
entry1 = tk.Entry(root)
canvas.create_window(150,15,window=entry1)
# submit button
submit = tk.Button(text="Solve",command=math,height=1, width=3)
canvas.create_window(280,15,window=submit)
root.mainloop()
———————————————————————————————
Feel free to drop a like and comment if you enjoy and video and let me know if you want me to do other types of programming videos ;) !!!
Видео How to build your own Wolfram Alpha — Math Equation Visualization with Tkinter and wolframalpha API канала Andrew Fung
Показать
Комментарии отсутствуют
Информация о видео
Другие видео канала
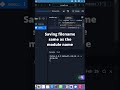
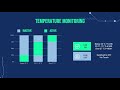
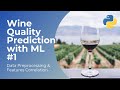
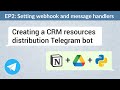
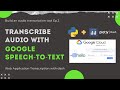
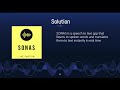

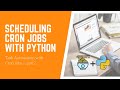



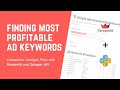
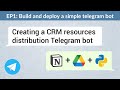

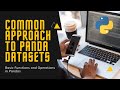
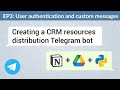

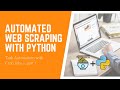

