How to Fix Java Sorting Test Failures
Learn how to troubleshoot and fix Java sorting test failures in this comprehensive guide. We'll walk you through essential debugging strategies and best practices to ensure your sorting algorithm functions correctly.
---
This video is based on the question https://stackoverflow.com/q/69210937/ asked by the user 'user13921628' ( https://stackoverflow.com/u/13921628/ ) and on the answer https://stackoverflow.com/a/69211130/ provided by the user 'Navaneeth Sen' ( https://stackoverflow.com/u/449378/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Problem with -test a java sorting program
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Java Sorting Program Test Failures
When developing a Java sorting program, it's not just essential to sort an array correctly but also to ensure that the associated tests are functioning as intended. If your tests are failing, as in the case below, it can be frustrating. Fortunately, we can unpack the problem and find solutions together.
The Problem: Test Method Failures
You implemented a Java sorting algorithm designed to sort an integer array in ascending order. Your program structure includes a Sorting class that performs the sorting and a SortingTest class that tests various scenarios. However, you encounter failures with several test methods, which you need to resolve. Here’s the critical part of your Sorting class:
[[See Video to Reveal this Text or Code Snippet]]
While the implementation appears straightforward, there are pitfalls in your test methods that can lead to unexpected results, especially with null inputs or edge cases. Let’s discuss how to improve these test methods to accurately assess the functionality of your sorting algorithm.
Common Pitfalls in Sorting Test Cases
1. Handling Null Values
One of the tests in your SortingTest class is designed to check how your sorting method reacts to null input values. Instead of just asserting that a null input equals another null output, you're missing the exception check. Consider the following:
[[See Video to Reveal this Text or Code Snippet]]
2. Testing Edge Cases
Your sorting algorithm should also be tested against various scenarios, not just typical cases. Make sure to include tests for the following:
Empty Arrays: Ensure your algorithm can handle an empty array without errors.
Single Element Arrays: An array with only one element should return that element.
Already Sorted Arrays: Provide an already sorted array and check if the output matches the input.
Randomized Arrays: Include random arrays to evaluate the performance of your sorting algorithm.
3. Reviewing Failure Counts
For tests like testLazySorting() and testTrickySorting(), it's crucial to analyze why they fail. Here’s how you might write one correctly:
[[See Video to Reveal this Text or Code Snippet]]
Make sure to compare the expected output against what the sorting method generates. If the arrays do not match, debug the sorting method to see if it is functioning as intended.
Conclusion: Best Practices for Java Testing
Here are some takeaways to ensure your sorting program is functioning well with associated tests:
Implement Exception Handling: Always account for null inputs and other edge cases.
Use Assert Statements Wisely: Make sure that your assertions accurately reflect the expected outcomes based on the inputs.
Run a Variety of Test Cases: Test against different scenarios, not just the typical cases, to ensure robustness.
Debugging: When tests fail, take time to isolate and debug the specific sections of code that aren't performing as expected.
By following these strategies, your Java sorting program should be well-equipped to handle various inputs and outputs effectively, all while passing the comprehensive tests you set up.
Final Thoughts
Debugging can often be a daunting task, especially when dealing with multiple test cases. Remember, coding is a skill honed through practice and patience. Keep testing, refactoring, and improving your code, and you’ll become a more proficient developer.
Видео How to Fix Java Sorting Test Failures канала vlogize
Problem with @test a java sorting program, java, arrays, sorting, testing
---
This video is based on the question https://stackoverflow.com/q/69210937/ asked by the user 'user13921628' ( https://stackoverflow.com/u/13921628/ ) and on the answer https://stackoverflow.com/a/69211130/ provided by the user 'Navaneeth Sen' ( https://stackoverflow.com/u/449378/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Problem with -test a java sorting program
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Java Sorting Program Test Failures
When developing a Java sorting program, it's not just essential to sort an array correctly but also to ensure that the associated tests are functioning as intended. If your tests are failing, as in the case below, it can be frustrating. Fortunately, we can unpack the problem and find solutions together.
The Problem: Test Method Failures
You implemented a Java sorting algorithm designed to sort an integer array in ascending order. Your program structure includes a Sorting class that performs the sorting and a SortingTest class that tests various scenarios. However, you encounter failures with several test methods, which you need to resolve. Here’s the critical part of your Sorting class:
[[See Video to Reveal this Text or Code Snippet]]
While the implementation appears straightforward, there are pitfalls in your test methods that can lead to unexpected results, especially with null inputs or edge cases. Let’s discuss how to improve these test methods to accurately assess the functionality of your sorting algorithm.
Common Pitfalls in Sorting Test Cases
1. Handling Null Values
One of the tests in your SortingTest class is designed to check how your sorting method reacts to null input values. Instead of just asserting that a null input equals another null output, you're missing the exception check. Consider the following:
[[See Video to Reveal this Text or Code Snippet]]
2. Testing Edge Cases
Your sorting algorithm should also be tested against various scenarios, not just typical cases. Make sure to include tests for the following:
Empty Arrays: Ensure your algorithm can handle an empty array without errors.
Single Element Arrays: An array with only one element should return that element.
Already Sorted Arrays: Provide an already sorted array and check if the output matches the input.
Randomized Arrays: Include random arrays to evaluate the performance of your sorting algorithm.
3. Reviewing Failure Counts
For tests like testLazySorting() and testTrickySorting(), it's crucial to analyze why they fail. Here’s how you might write one correctly:
[[See Video to Reveal this Text or Code Snippet]]
Make sure to compare the expected output against what the sorting method generates. If the arrays do not match, debug the sorting method to see if it is functioning as intended.
Conclusion: Best Practices for Java Testing
Here are some takeaways to ensure your sorting program is functioning well with associated tests:
Implement Exception Handling: Always account for null inputs and other edge cases.
Use Assert Statements Wisely: Make sure that your assertions accurately reflect the expected outcomes based on the inputs.
Run a Variety of Test Cases: Test against different scenarios, not just the typical cases, to ensure robustness.
Debugging: When tests fail, take time to isolate and debug the specific sections of code that aren't performing as expected.
By following these strategies, your Java sorting program should be well-equipped to handle various inputs and outputs effectively, all while passing the comprehensive tests you set up.
Final Thoughts
Debugging can often be a daunting task, especially when dealing with multiple test cases. Remember, coding is a skill honed through practice and patience. Keep testing, refactoring, and improving your code, and you’ll become a more proficient developer.
Видео How to Fix Java Sorting Test Failures канала vlogize
Problem with @test a java sorting program, java, arrays, sorting, testing
Показать
Комментарии отсутствуют
Информация о видео
Вчера, 7:06:11
00:01:53
Другие видео канала


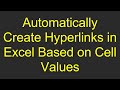
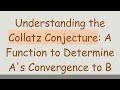


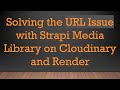
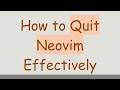

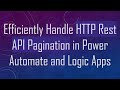
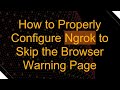


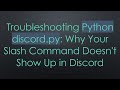

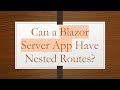
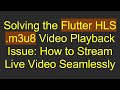
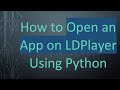
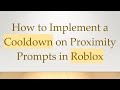

