An Introduction to Networking with URLSession and SwiftUI - raywenderlich.com
Learn about the URLSession and how the framework evolved in the way that it did. Also learn about the course goals.
---
Watch the full course over here:
https://www.raywenderlich.com/10376245-networking-with-urlsession
---
Learn how to use URLSession, Apple's networking API, including when and how to use data, download and upload tasks, with or without a custom session delegate, and how the system manages background sessions and WebSockets. Leverage the Combine API and SwiftUI to take your networking to the next level. Updated for iOS 13 and Swift 5.2.
About URLSession:
(from Apple's Developer Documentation):
https://developer.apple.com/documentation/foundation/urlsession
The URLSession class and related classes provide an API for downloading data from and uploading data to endpoints indicated by URLs. Your app can also use this API to perform background downloads when your app isn’t running or, in iOS, while your app is suspended. You can use the related URLSessionDelegate and URLSessionTaskDelegate to support authentication and receive events like redirection and task completion.
Note: The URLSession API involves many different classes that work together in a fairly complex way which may not be obvious if you read the reference documentation by itself. Before using the API, read the overview in the URL Loading System topic. The articles in the Essentials, Uploading, and Downloading sections offer examples of performing common tasks with URLSession.
Your app creates one or more URLSession instances, each of which coordinates a group of related data-transfer tasks. For example, if you’re creating a web browser, your app might create one session per tab or window, or one session for interactive use and another for background downloads. Within each session, your app adds a series of tasks, each of which represents a request for a specific URL (following HTTP redirects, if necessary).
Types of URL Sessions
The tasks within a given URL session share a common session configuration object, which defines connection behavior, like the maximum number of simultaneous connections to make to a single host, whether connections can use the cellular network, and so on.
URLSession has a singleton shared session (which doesn’t have a configuration object) for basic requests. It’s not as customizable as sessions you create, but it serves as a good starting point if you have very limited requirements. You access this session by calling the shared class method. For other kinds of sessions, you create a URLSession with one of three kinds of configurations:
* A default session behaves much like the shared session, but lets you configure it. You can also assign a delegate to the default session to obtain data incrementally.
* Ephemeral sessions are similar to shared sessions, but don’t write caches, cookies, or credentials to disk.
* Background sessions let you perform uploads and downloads of content in the background while your app isn't running.
See Creating a Session Configuration Object in the URLSessionConfiguration class for details on creating each type of configuration.
Types of URL Session Tasks
Within a session, you create tasks that optionally upload data to a server and then retrieve data from the server either as a file on disk or as one or more NSData objects in memory. The URLSession API provides four types of tasks:
* Data tasks send and receive data using NSData objects. Data tasks are intended for short, often interactive requests to a server.
* Upload tasks are similar to data tasks, but they also send data (often in the form of a file), and support background uploads while the app isn’t running.
* Download tasks retrieve data in the form of a file, and support background downloads and uploads while the app isn’t running.
* WebSocket tasks exchange messages over TCP and TLS, using the WebSocket protocol defined in RFC 6455.
Using a Session Delegate
Tasks in a session also share a common delegate object. You implement this delegate to provide and obtain information when various events occur, including when:
* Authentication fails.
* Data arrives from the server.
* Data becomes available for caching.
If you don’t need the features provided by a delegate, you can use this API without providing one by passing nil when you create a session.
Important: The session object keeps a strong reference to the delegate until your app exits or explicitly invalidates the session. If you don’t invalidate the session, your app leaks memory until the app terminates.
Asynchronicity and URL Sessions
Like most networking APIs, the URLSession API is highly asynchronous. It returns data to your app in one of two ways, depending on the methods you call:
* By calling a completion handler block when a transfer finishes successfully or with an error.
* By calling methods on the session’s delegate as data arrives and when the transfer is complete.
Видео An Introduction to Networking with URLSession and SwiftUI - raywenderlich.com канала Kodeco
---
Watch the full course over here:
https://www.raywenderlich.com/10376245-networking-with-urlsession
---
Learn how to use URLSession, Apple's networking API, including when and how to use data, download and upload tasks, with or without a custom session delegate, and how the system manages background sessions and WebSockets. Leverage the Combine API and SwiftUI to take your networking to the next level. Updated for iOS 13 and Swift 5.2.
About URLSession:
(from Apple's Developer Documentation):
https://developer.apple.com/documentation/foundation/urlsession
The URLSession class and related classes provide an API for downloading data from and uploading data to endpoints indicated by URLs. Your app can also use this API to perform background downloads when your app isn’t running or, in iOS, while your app is suspended. You can use the related URLSessionDelegate and URLSessionTaskDelegate to support authentication and receive events like redirection and task completion.
Note: The URLSession API involves many different classes that work together in a fairly complex way which may not be obvious if you read the reference documentation by itself. Before using the API, read the overview in the URL Loading System topic. The articles in the Essentials, Uploading, and Downloading sections offer examples of performing common tasks with URLSession.
Your app creates one or more URLSession instances, each of which coordinates a group of related data-transfer tasks. For example, if you’re creating a web browser, your app might create one session per tab or window, or one session for interactive use and another for background downloads. Within each session, your app adds a series of tasks, each of which represents a request for a specific URL (following HTTP redirects, if necessary).
Types of URL Sessions
The tasks within a given URL session share a common session configuration object, which defines connection behavior, like the maximum number of simultaneous connections to make to a single host, whether connections can use the cellular network, and so on.
URLSession has a singleton shared session (which doesn’t have a configuration object) for basic requests. It’s not as customizable as sessions you create, but it serves as a good starting point if you have very limited requirements. You access this session by calling the shared class method. For other kinds of sessions, you create a URLSession with one of three kinds of configurations:
* A default session behaves much like the shared session, but lets you configure it. You can also assign a delegate to the default session to obtain data incrementally.
* Ephemeral sessions are similar to shared sessions, but don’t write caches, cookies, or credentials to disk.
* Background sessions let you perform uploads and downloads of content in the background while your app isn't running.
See Creating a Session Configuration Object in the URLSessionConfiguration class for details on creating each type of configuration.
Types of URL Session Tasks
Within a session, you create tasks that optionally upload data to a server and then retrieve data from the server either as a file on disk or as one or more NSData objects in memory. The URLSession API provides four types of tasks:
* Data tasks send and receive data using NSData objects. Data tasks are intended for short, often interactive requests to a server.
* Upload tasks are similar to data tasks, but they also send data (often in the form of a file), and support background uploads while the app isn’t running.
* Download tasks retrieve data in the form of a file, and support background downloads and uploads while the app isn’t running.
* WebSocket tasks exchange messages over TCP and TLS, using the WebSocket protocol defined in RFC 6455.
Using a Session Delegate
Tasks in a session also share a common delegate object. You implement this delegate to provide and obtain information when various events occur, including when:
* Authentication fails.
* Data arrives from the server.
* Data becomes available for caching.
If you don’t need the features provided by a delegate, you can use this API without providing one by passing nil when you create a session.
Important: The session object keeps a strong reference to the delegate until your app exits or explicitly invalidates the session. If you don’t invalidate the session, your app leaks memory until the app terminates.
Asynchronicity and URL Sessions
Like most networking APIs, the URLSession API is highly asynchronous. It returns data to your app in one of two ways, depending on the methods you call:
* By calling a completion handler block when a transfer finishes successfully or with an error.
* By calling methods on the session’s delegate as data arrives and when the transfer is complete.
Видео An Introduction to Networking with URLSession and SwiftUI - raywenderlich.com канала Kodeco
Показать
Комментарии отсутствуют
Информация о видео
Другие видео канала





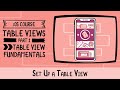

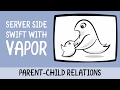

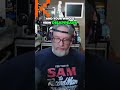


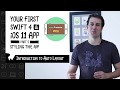

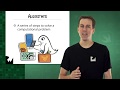
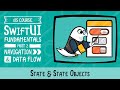


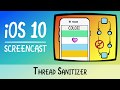

