How to Implement Position Independent Code for Cortex-M0+ Dual Applications
Discover how to implement position independent code for Cortex-M0+, enabling effective dual application management without the need for external flash storage.
---
This video is based on the question https://stackoverflow.com/q/70677559/ asked by the user 'bam' ( https://stackoverflow.com/u/1787919/ ) and on the answer https://stackoverflow.com/a/70683353/ provided by the user 'old_timer' ( https://stackoverflow.com/u/16007/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Cortex-M0+ dual application/image linking
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Implement Position Independent Code for Cortex-M0+ Dual Applications
In embedded systems, particularly in applications utilizing the Cortex-M0+ microcontroller, there arises a common challenge when managing multiple application images. Specifically, when aiming to switch between two applications stored in the device's flash memory, developers encounter a dilemma: how to link and run these applications dynamically without relying upon external flash storage or complicating the bootloading process. This post explores how to utilize position independent code (PIC) for this dual application scenario, simplifying updates and reducing flash requirements.
The Problem Explained
For many microcontroller applications, a bootloader + application architecture is prevalent where different firmware versions are stored externally. However, aiming to streamline this with two applications stored internally can lead to complications:
Dynamic Application Switching: You want one application (let's call it App A) to be able to perform over-the-air (OTA) updates to another application (App B).
Memory Mapping Uncertainty: Upon rebooting, you need to switch between the applications for execution, but the Memory Address of each app is unknown at compile-time. This results in difficulties defining jump addresses or interrupt service tables.
Linking Challenges: With insufficient information, linking tools cannot determine where to set code addresses, making it impossible for both applications to interact efficiently.
The Solution: Position Independent Code (PIC)
Understanding Position Independent Code
Position Independent Code allows the executable to run from any memory address. This is vital when switching between two applications because we cannot always predict where each application resides in memory:
Global Offset Table (GOT): By using GOT, the code can adjust function calls and variable accesses dynamically based on the execution address.
Entry Point Adjustments: Before launching the main application code, the initial setup must adjust the GOT to point to the correct memory locations.
Implementation Steps
Here’s how you can implement PIC using the GNU toolchain with a Cortex-M0+:
Step 1: Bootloader Design
The bootloader must operate from a fixed location in flash, which decides which application path to use when the device starts:
Include code to mark which application is more recent or should be prioritized for booting.
Design the bootloader to execute from a consistent entry point for both applications.
Step 2: Code Compilation
Use the following flags while compiling your application to make it position independent:
[[See Video to Reveal this Text or Code Snippet]]
The -fPIC flag creates position independent code.
Add necessary linker scripts to define memory regions.
Step 3: Linker Script Configuration
Use a linker script that defines both .text and .data sections, ensuring that your GOT remains fixed relative to the executable sections.
Example linker script:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Interaction Between Applications
Both applications should be able to read/write to the correct memory regions dynamically. This might involve:
Adjusting the GOT before executing any essential code.
Providing streamlined functions in both applications to facilitate updates or cross-communication.
Conclusion
Employing position independent code is a robust and efficient solution for managing dual applications on the Cortex-M0+. By adopting this approach, you benefit from:
Reduced Memory Requirements: Eliminating the need for external storage simplifies hardware design.
Smooth Transitioning: Updating applications over-the-air becomes feasible without r
Видео How to Implement Position Independent Code for Cortex-M0+ Dual Applications канала vlogize
---
This video is based on the question https://stackoverflow.com/q/70677559/ asked by the user 'bam' ( https://stackoverflow.com/u/1787919/ ) and on the answer https://stackoverflow.com/a/70683353/ provided by the user 'old_timer' ( https://stackoverflow.com/u/16007/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Cortex-M0+ dual application/image linking
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Implement Position Independent Code for Cortex-M0+ Dual Applications
In embedded systems, particularly in applications utilizing the Cortex-M0+ microcontroller, there arises a common challenge when managing multiple application images. Specifically, when aiming to switch between two applications stored in the device's flash memory, developers encounter a dilemma: how to link and run these applications dynamically without relying upon external flash storage or complicating the bootloading process. This post explores how to utilize position independent code (PIC) for this dual application scenario, simplifying updates and reducing flash requirements.
The Problem Explained
For many microcontroller applications, a bootloader + application architecture is prevalent where different firmware versions are stored externally. However, aiming to streamline this with two applications stored internally can lead to complications:
Dynamic Application Switching: You want one application (let's call it App A) to be able to perform over-the-air (OTA) updates to another application (App B).
Memory Mapping Uncertainty: Upon rebooting, you need to switch between the applications for execution, but the Memory Address of each app is unknown at compile-time. This results in difficulties defining jump addresses or interrupt service tables.
Linking Challenges: With insufficient information, linking tools cannot determine where to set code addresses, making it impossible for both applications to interact efficiently.
The Solution: Position Independent Code (PIC)
Understanding Position Independent Code
Position Independent Code allows the executable to run from any memory address. This is vital when switching between two applications because we cannot always predict where each application resides in memory:
Global Offset Table (GOT): By using GOT, the code can adjust function calls and variable accesses dynamically based on the execution address.
Entry Point Adjustments: Before launching the main application code, the initial setup must adjust the GOT to point to the correct memory locations.
Implementation Steps
Here’s how you can implement PIC using the GNU toolchain with a Cortex-M0+:
Step 1: Bootloader Design
The bootloader must operate from a fixed location in flash, which decides which application path to use when the device starts:
Include code to mark which application is more recent or should be prioritized for booting.
Design the bootloader to execute from a consistent entry point for both applications.
Step 2: Code Compilation
Use the following flags while compiling your application to make it position independent:
[[See Video to Reveal this Text or Code Snippet]]
The -fPIC flag creates position independent code.
Add necessary linker scripts to define memory regions.
Step 3: Linker Script Configuration
Use a linker script that defines both .text and .data sections, ensuring that your GOT remains fixed relative to the executable sections.
Example linker script:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Interaction Between Applications
Both applications should be able to read/write to the correct memory regions dynamically. This might involve:
Adjusting the GOT before executing any essential code.
Providing streamlined functions in both applications to facilitate updates or cross-communication.
Conclusion
Employing position independent code is a robust and efficient solution for managing dual applications on the Cortex-M0+. By adopting this approach, you benefit from:
Reduced Memory Requirements: Eliminating the need for external storage simplifies hardware design.
Smooth Transitioning: Updating applications over-the-air becomes feasible without r
Видео How to Implement Position Independent Code for Cortex-M0+ Dual Applications канала vlogize
Комментарии отсутствуют
Информация о видео
30 марта 2025 г. 19:57:28
00:02:04
Другие видео канала
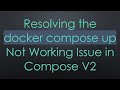


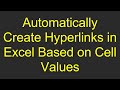
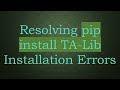
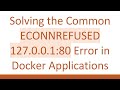


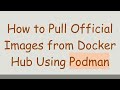

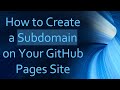

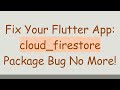
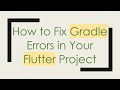
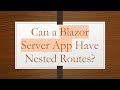
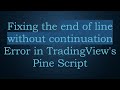
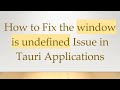
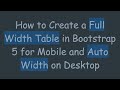
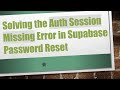

