Python for Network Automation:Create Device Classes and SSH Connection Method for Executing Commands
#pythonautomation #ciscopython #networkautomation
Unlock the Power of Network Automation: Enroll in our Comprehensive Udemy Course Today
https://www.udemy.com/course/python-for-network-engineers/?referralCode=35A75AAE1ACA94A15829
Script
---------------
import time
import paramiko
import re
class CiscoDevice:
def __init__(self, ip, username, password):
self.ip = ip
self.username = username
self.password = password
self.port = 22
def connect(self):
print(f"Connecting to the device {self.ip}")
session = paramiko.client.SSHClient()
session.set_missing_host_key_policy(paramiko.client.AutoAddPolicy())
session.connect(hostname=self.ip,
username=self.username,
password=self.password,
look_for_keys=False, allow_agent=False)
self.device_access = session.invoke_shell()
self.device_access.send('terminal len 0\n')
time.sleep(2)
print("Connected to the device\n")
def get_show_output(self, show_command):
self.device_access.send(f"{show_command}\n")
time.sleep(3)
sh_output = self.device_access.recv(65000).decode()
return sh_output
def version(self):
sh_ver_output = self.get_show_output('show version')
version_pattern = re.compile(r'Cisco .+ Software, Version (\S+)')
version_match = version_pattern.search(sh_ver_output)
return 'IOS Version'.ljust(18) + ': ' + version_match.group(1)
def hostname(self):
sh_run_output = self.get_show_output('show run')
hostname_pattern = re.compile(r'hostname (\S+)')
host_match = hostname_pattern.search(sh_run_output)
return 'Hostname'.ljust(18) + ': ' + str(host_match.group(1))
def disconnect(self):
self.device_access.close()
print("\nClosed SSH Connection")
𝗣𝗹𝗮𝘆𝗹𝗶𝘀𝘁: 𝗣𝘆𝘁𝗵𝗼𝗻 𝗟𝗲𝗮𝗿𝗻𝗶𝗻𝗴 𝗳𝗼𝗿 𝗡𝗲𝘁𝘄𝗼𝗿𝗸 𝗘𝗻𝗴𝗶𝗻𝗲𝗲𝗿𝘀(𝟭𝟮𝟬+ 𝗩𝗶𝗱𝗲𝗼𝘀)
https://www.youtube.com/watch?v=5zN6c-kuda0&list=PLOocymQm7YWakdZkBfCRIC06fv7xQE85N
☸𝗣𝗹𝗲𝗮𝘀𝗲 𝗳𝗼𝗹𝗹𝗼𝘄 𝗯𝗲𝗹𝗼𝘄 𝗚𝗶𝘁𝗛𝘂𝗯 𝗣𝗮𝗴𝗲 𝗳𝗼𝗿 𝘁𝗵𝗲 𝗹𝗮𝘁𝗲𝘀𝘁 𝗰𝗼𝗱𝗲𝘀:
https://github.com/network-evolution
𝗣𝗹𝗮𝘆𝗹𝗶𝘀𝘁: 𝗖𝗶𝘀𝗰𝗼 𝗡𝗫𝗔𝗣𝗜-𝗖𝗟𝗜 𝗣𝘆𝘁𝗵𝗼𝗻 𝗔𝘂𝘁𝗼𝗺𝗮𝘁𝗶𝗼𝗻: 𝗡𝗲𝘅𝘂𝘀 𝟵𝗸 :𝗣𝗮𝗿𝘀𝗲 𝗷𝘀𝗼𝗻 𝗫𝗠𝗟 𝗖𝗼𝗻𝗳𝗶𝗴𝘂𝗿𝗮𝘁𝗶𝗼𝗻 𝗗𝗮𝘁𝗮
https://www.youtube.com/watch?v=Bhx0rW-t8jI&list=PLOocymQm7YWYYq3LVmNHbGbIhlD_GAK4a
𝗣𝗹𝗮𝘆𝗹𝗶𝘀𝘁: 𝗟𝗲𝗮𝗿𝗻 𝘁𝗼 𝗣𝗮𝗿𝘀𝗲 𝗖𝗶𝘀𝗰𝗼 𝗖𝗼𝗻𝗳𝗶𝗴𝘂𝗿𝗮𝘁𝗶𝗼𝗻 𝘂𝘀𝗶𝗻𝗴 𝗣𝘆𝘁𝗵𝗼𝗻 𝗥𝗲𝗴𝗘𝘅:𝗿𝗲 𝗧𝘂𝘁𝗼𝗿𝗶𝗮𝗹(𝟭𝟱+ 𝗩𝗶𝗱𝗲𝗼𝘀)
https://www.youtube.com/watch?v=PbP9tyV0Zao&list=PLOocymQm7YWY8Eksax8mjRSWbUijb7W93
In this tutorial we will see how to create methods inside class
how to set attributes in python class definition
how to create multiple instances of classes
python object orieneted programming network engineers tutorial
python oop access attributes of individual instances
python methods
how to create methods of class in python
what is methods in python
self argument in python class
basics of classes
what is classes and objects in python begineer tutorial
how to create methods inside calss basic tutorial with realitime networking example
self is the first argument
cisco device ssh connection example for python classes tutorial
self.argument
how to do print from class methods
how to create a python method inside device class for initiating ssh connection
create network device ssh atrributes ip, username, password
python classes tutorial
what is classes and objects in python
beginner training on python objects
basics of object oriented programming in python
what is classes, how to create and initialize and class, what is attributes and how to create class methods
A class will help us to logically group attributes and functions
how to create a blueprint of an object using python classes
classes and objects network automation usecase example
network engineers tutorial on classes and objects
advantages of classes over python functions
what is classes and objects in python beginner tutorial
classes and objects example tutorial
learn python classes and objects as a beginner
attributes are unique proterties which defines an object
methods are actions performed on object
methods are nothing but functions associated to class
how to create a class in python
capitalized words camelcase
init method in python class
builtin method in python
self is a variable name, python convention in __init__(self)
set attributes on a class object example
create an instance of class objects
ip as the attribute
how to pass arguments to class
give named arguments or positional arguments in python class
Видео Python for Network Automation:Create Device Classes and SSH Connection Method for Executing Commands канала NetworkEvolution
Unlock the Power of Network Automation: Enroll in our Comprehensive Udemy Course Today
https://www.udemy.com/course/python-for-network-engineers/?referralCode=35A75AAE1ACA94A15829
Script
---------------
import time
import paramiko
import re
class CiscoDevice:
def __init__(self, ip, username, password):
self.ip = ip
self.username = username
self.password = password
self.port = 22
def connect(self):
print(f"Connecting to the device {self.ip}")
session = paramiko.client.SSHClient()
session.set_missing_host_key_policy(paramiko.client.AutoAddPolicy())
session.connect(hostname=self.ip,
username=self.username,
password=self.password,
look_for_keys=False, allow_agent=False)
self.device_access = session.invoke_shell()
self.device_access.send('terminal len 0\n')
time.sleep(2)
print("Connected to the device\n")
def get_show_output(self, show_command):
self.device_access.send(f"{show_command}\n")
time.sleep(3)
sh_output = self.device_access.recv(65000).decode()
return sh_output
def version(self):
sh_ver_output = self.get_show_output('show version')
version_pattern = re.compile(r'Cisco .+ Software, Version (\S+)')
version_match = version_pattern.search(sh_ver_output)
return 'IOS Version'.ljust(18) + ': ' + version_match.group(1)
def hostname(self):
sh_run_output = self.get_show_output('show run')
hostname_pattern = re.compile(r'hostname (\S+)')
host_match = hostname_pattern.search(sh_run_output)
return 'Hostname'.ljust(18) + ': ' + str(host_match.group(1))
def disconnect(self):
self.device_access.close()
print("\nClosed SSH Connection")
𝗣𝗹𝗮𝘆𝗹𝗶𝘀𝘁: 𝗣𝘆𝘁𝗵𝗼𝗻 𝗟𝗲𝗮𝗿𝗻𝗶𝗻𝗴 𝗳𝗼𝗿 𝗡𝗲𝘁𝘄𝗼𝗿𝗸 𝗘𝗻𝗴𝗶𝗻𝗲𝗲𝗿𝘀(𝟭𝟮𝟬+ 𝗩𝗶𝗱𝗲𝗼𝘀)
https://www.youtube.com/watch?v=5zN6c-kuda0&list=PLOocymQm7YWakdZkBfCRIC06fv7xQE85N
☸𝗣𝗹𝗲𝗮𝘀𝗲 𝗳𝗼𝗹𝗹𝗼𝘄 𝗯𝗲𝗹𝗼𝘄 𝗚𝗶𝘁𝗛𝘂𝗯 𝗣𝗮𝗴𝗲 𝗳𝗼𝗿 𝘁𝗵𝗲 𝗹𝗮𝘁𝗲𝘀𝘁 𝗰𝗼𝗱𝗲𝘀:
https://github.com/network-evolution
𝗣𝗹𝗮𝘆𝗹𝗶𝘀𝘁: 𝗖𝗶𝘀𝗰𝗼 𝗡𝗫𝗔𝗣𝗜-𝗖𝗟𝗜 𝗣𝘆𝘁𝗵𝗼𝗻 𝗔𝘂𝘁𝗼𝗺𝗮𝘁𝗶𝗼𝗻: 𝗡𝗲𝘅𝘂𝘀 𝟵𝗸 :𝗣𝗮𝗿𝘀𝗲 𝗷𝘀𝗼𝗻 𝗫𝗠𝗟 𝗖𝗼𝗻𝗳𝗶𝗴𝘂𝗿𝗮𝘁𝗶𝗼𝗻 𝗗𝗮𝘁𝗮
https://www.youtube.com/watch?v=Bhx0rW-t8jI&list=PLOocymQm7YWYYq3LVmNHbGbIhlD_GAK4a
𝗣𝗹𝗮𝘆𝗹𝗶𝘀𝘁: 𝗟𝗲𝗮𝗿𝗻 𝘁𝗼 𝗣𝗮𝗿𝘀𝗲 𝗖𝗶𝘀𝗰𝗼 𝗖𝗼𝗻𝗳𝗶𝗴𝘂𝗿𝗮𝘁𝗶𝗼𝗻 𝘂𝘀𝗶𝗻𝗴 𝗣𝘆𝘁𝗵𝗼𝗻 𝗥𝗲𝗴𝗘𝘅:𝗿𝗲 𝗧𝘂𝘁𝗼𝗿𝗶𝗮𝗹(𝟭𝟱+ 𝗩𝗶𝗱𝗲𝗼𝘀)
https://www.youtube.com/watch?v=PbP9tyV0Zao&list=PLOocymQm7YWY8Eksax8mjRSWbUijb7W93
In this tutorial we will see how to create methods inside class
how to set attributes in python class definition
how to create multiple instances of classes
python object orieneted programming network engineers tutorial
python oop access attributes of individual instances
python methods
how to create methods of class in python
what is methods in python
self argument in python class
basics of classes
what is classes and objects in python begineer tutorial
how to create methods inside calss basic tutorial with realitime networking example
self is the first argument
cisco device ssh connection example for python classes tutorial
self.argument
how to do print from class methods
how to create a python method inside device class for initiating ssh connection
create network device ssh atrributes ip, username, password
python classes tutorial
what is classes and objects in python
beginner training on python objects
basics of object oriented programming in python
what is classes, how to create and initialize and class, what is attributes and how to create class methods
A class will help us to logically group attributes and functions
how to create a blueprint of an object using python classes
classes and objects network automation usecase example
network engineers tutorial on classes and objects
advantages of classes over python functions
what is classes and objects in python beginner tutorial
classes and objects example tutorial
learn python classes and objects as a beginner
attributes are unique proterties which defines an object
methods are actions performed on object
methods are nothing but functions associated to class
how to create a class in python
capitalized words camelcase
init method in python class
builtin method in python
self is a variable name, python convention in __init__(self)
set attributes on a class object example
create an instance of class objects
ip as the attribute
how to pass arguments to class
give named arguments or positional arguments in python class
Видео Python for Network Automation:Create Device Classes and SSH Connection Method for Executing Commands канала NetworkEvolution
Показать
Комментарии отсутствуют
Информация о видео
Другие видео канала


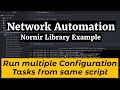
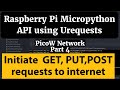


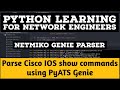

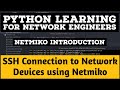
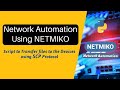
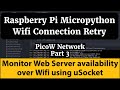

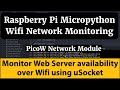
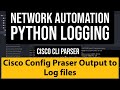

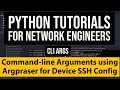

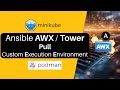

