Why useSelector() with Conditions Triggers ESLint Errors in React
Learn why using `useSelector()` with conditional calls violates React Hook rules and discover simple solutions to resolve ESLint errors.
---
This video is based on the question https://stackoverflow.com/q/74399935/ asked by the user 'ddaudiosolutions' ( https://stackoverflow.com/u/12289678/ ) and on the answer https://stackoverflow.com/a/74401894/ provided by the user 'phry' ( https://stackoverflow.com/u/2075944/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: why useSelector() with conditions EslintError
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding ESLint Errors with useSelector(): The Problem
When working with React and Redux, you might stumble upon a common issue involving the useSelector() hook—a valuable tool for accessing Redux state directly in your functional components. It’s easy to assume that you can control how and when you pull data from your store using conditions. However, doing so can lead to violations of the rules of hooks, prompting ESLint errors and potentially causing serious issues in your application.
The Root of the Problem
Consider this code snippet that you might be accustomed to:
[[See Video to Reveal this Text or Code Snippet]]
At first glance, this seems logical—if useSelector() has valid data, use it; otherwise, fall back on local storage. However, the problem arises from how many times useSelector() is called. In the code above, depending on the condition, useSelector may run once or twice.
Why This Is a Problem
React enforces strict rules for using hooks. The crux is that hooks must be called in the same order on every render. When the number of calls to useSelector() can vary, it may lead to inconsistencies that cause React to crash your application. As a result, ESLint flags this as an error.
Solutions Explained
Fortunately, addressing this issue is relatively simple! Below are two approaches to rework your code while maintaining a single invocation of useSelector() and staying within the rules.
1. Use a Variable to Store Selector Value
Instead of calling useSelector() conditionally, you can store the result in a variable and then check the variable. Here’s how:
[[See Video to Reveal this Text or Code Snippet]]
This method ensures that useSelector() is called only once regardless of the condition.
2. Incorporate Logic Within the useSelector()
Alternatively, you can utilize a single call to useSelector() with embedded logic directly in the selector function. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
This also meets the requirements of React’s hooks rules and avoids multiple calls.
Conclusion
In summary, invoking useSelector() conditionally can lead to ESLint errors and unpredictable behavior in your React applications. By either using a variable to store the selector's return value or embedding the logic within the useSelector() call itself, you can maintain compliance with React’s hooks rules and keep your codebase clean and efficient.
Remember, always call hooks at the top level and never inside conditions or loops. Adopting the aforementioned strategies will help you avoid issues with React while creating robust applications.
Видео Why useSelector() with Conditions Triggers ESLint Errors in React канала vlogize
---
This video is based on the question https://stackoverflow.com/q/74399935/ asked by the user 'ddaudiosolutions' ( https://stackoverflow.com/u/12289678/ ) and on the answer https://stackoverflow.com/a/74401894/ provided by the user 'phry' ( https://stackoverflow.com/u/2075944/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: why useSelector() with conditions EslintError
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding ESLint Errors with useSelector(): The Problem
When working with React and Redux, you might stumble upon a common issue involving the useSelector() hook—a valuable tool for accessing Redux state directly in your functional components. It’s easy to assume that you can control how and when you pull data from your store using conditions. However, doing so can lead to violations of the rules of hooks, prompting ESLint errors and potentially causing serious issues in your application.
The Root of the Problem
Consider this code snippet that you might be accustomed to:
[[See Video to Reveal this Text or Code Snippet]]
At first glance, this seems logical—if useSelector() has valid data, use it; otherwise, fall back on local storage. However, the problem arises from how many times useSelector() is called. In the code above, depending on the condition, useSelector may run once or twice.
Why This Is a Problem
React enforces strict rules for using hooks. The crux is that hooks must be called in the same order on every render. When the number of calls to useSelector() can vary, it may lead to inconsistencies that cause React to crash your application. As a result, ESLint flags this as an error.
Solutions Explained
Fortunately, addressing this issue is relatively simple! Below are two approaches to rework your code while maintaining a single invocation of useSelector() and staying within the rules.
1. Use a Variable to Store Selector Value
Instead of calling useSelector() conditionally, you can store the result in a variable and then check the variable. Here’s how:
[[See Video to Reveal this Text or Code Snippet]]
This method ensures that useSelector() is called only once regardless of the condition.
2. Incorporate Logic Within the useSelector()
Alternatively, you can utilize a single call to useSelector() with embedded logic directly in the selector function. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
This also meets the requirements of React’s hooks rules and avoids multiple calls.
Conclusion
In summary, invoking useSelector() conditionally can lead to ESLint errors and unpredictable behavior in your React applications. By either using a variable to store the selector's return value or embedding the logic within the useSelector() call itself, you can maintain compliance with React’s hooks rules and keep your codebase clean and efficient.
Remember, always call hooks at the top level and never inside conditions or loops. Adopting the aforementioned strategies will help you avoid issues with React while creating robust applications.
Видео Why useSelector() with Conditions Triggers ESLint Errors in React канала vlogize
Комментарии отсутствуют
Информация о видео
25 марта 2025 г. 0:34:24
00:01:35
Другие видео канала



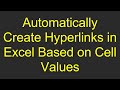


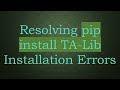
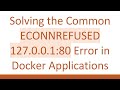

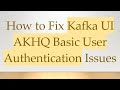
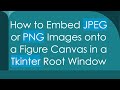
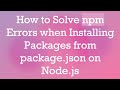
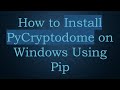
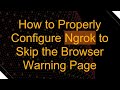
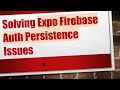
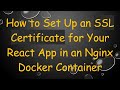
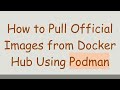

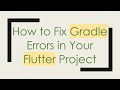
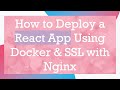
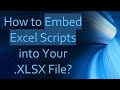