Efficient Ways to Randomly Interpolate Between Two Numpy Arrays
Discover how to create an array with random values between two Numpy arrays with minimal operations. Easy to follow steps provided!
---
This video is based on the question https://stackoverflow.com/q/69019367/ asked by the user 'Smer5' ( https://stackoverflow.com/u/1607242/ ) and on the answer https://stackoverflow.com/a/69019444/ provided by the user 'Mad Physicist' ( https://stackoverflow.com/u/2988730/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Random interpolation between 2 numpy arrays
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficient Ways to Randomly Interpolate Between Two Numpy Arrays
Working with Numpy arrays offers a wide array of tools for numerical computation in Python. However, creating random interpolations between two arrays can be somewhat tricky, especially when you want to achieve this with minimal complexity. In this guide, we will explore how to perform random interpolation between two Numpy arrays that contain integer values, making it easier to manipulate and generate new data efficiently.
Understanding the Problem
Let’s start with the problem at hand: you want to interpolate between two Numpy arrays a and b that are of the same dimension. The goal is to generate a new array c where each element is a random integer value that lies between the corresponding elements of arrays a and b. For example, given:
[[See Video to Reveal this Text or Code Snippet]]
The expected output is an array of integers c that contains random values between these two ranges, such as:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To achieve this, Numpy provides simple yet powerful functions that allow for uniform distribution generation. Let's break down the steps to interpolate these arrays effectively.
Setup Numpy
Before we start, ensure that you have the Numpy library installed. You can install it using pip if you haven't done so:
[[See Video to Reveal this Text or Code Snippet]]
Generating Random Integers
Numpy has a straightforward function for generating random integers between two bounds, which can help us solve this problem efficiently.
Use Numpy's random module: To interpolate between two arrays, we will leverage the integers function from Numpy's random module.
Define the arrays: Let's define our example arrays.
[[See Video to Reveal this Text or Code Snippet]]
Random Integer Generation:
Here’s the code you’ll want to use:
[[See Video to Reveal this Text or Code Snippet]]
The integers function generates random integers from a to b, where endpoint=True allows for inclusion of values at the maximum boundary.
Complete Example Code
[[See Video to Reveal this Text or Code Snippet]]
Result Interpretation
The result c is a Numpy array filled with integers that are randomly selected from the ranges specified by a and b. Each time you run the function, the output will be different due to the randomness factor, providing a variety of potential outputs.
Conclusion
In summary, generating random integers between two Numpy arrays can be accomplished with minimal effort using Numpy’s built-in functions. This method is efficient and simplifies your codebase while allowing for the flexibility of random data generation. So next time you need to create a child array with random values drawn from two parent arrays, remember to utilize the powerful capabilities of Numpy for seamless results!
Embrace the magical functionality of Numpy and enhance your data manipulation skills today!
Видео Efficient Ways to Randomly Interpolate Between Two Numpy Arrays канала vlogize
Random interpolation between 2 numpy arrays, python, numpy
---
This video is based on the question https://stackoverflow.com/q/69019367/ asked by the user 'Smer5' ( https://stackoverflow.com/u/1607242/ ) and on the answer https://stackoverflow.com/a/69019444/ provided by the user 'Mad Physicist' ( https://stackoverflow.com/u/2988730/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Random interpolation between 2 numpy arrays
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficient Ways to Randomly Interpolate Between Two Numpy Arrays
Working with Numpy arrays offers a wide array of tools for numerical computation in Python. However, creating random interpolations between two arrays can be somewhat tricky, especially when you want to achieve this with minimal complexity. In this guide, we will explore how to perform random interpolation between two Numpy arrays that contain integer values, making it easier to manipulate and generate new data efficiently.
Understanding the Problem
Let’s start with the problem at hand: you want to interpolate between two Numpy arrays a and b that are of the same dimension. The goal is to generate a new array c where each element is a random integer value that lies between the corresponding elements of arrays a and b. For example, given:
[[See Video to Reveal this Text or Code Snippet]]
The expected output is an array of integers c that contains random values between these two ranges, such as:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To achieve this, Numpy provides simple yet powerful functions that allow for uniform distribution generation. Let's break down the steps to interpolate these arrays effectively.
Setup Numpy
Before we start, ensure that you have the Numpy library installed. You can install it using pip if you haven't done so:
[[See Video to Reveal this Text or Code Snippet]]
Generating Random Integers
Numpy has a straightforward function for generating random integers between two bounds, which can help us solve this problem efficiently.
Use Numpy's random module: To interpolate between two arrays, we will leverage the integers function from Numpy's random module.
Define the arrays: Let's define our example arrays.
[[See Video to Reveal this Text or Code Snippet]]
Random Integer Generation:
Here’s the code you’ll want to use:
[[See Video to Reveal this Text or Code Snippet]]
The integers function generates random integers from a to b, where endpoint=True allows for inclusion of values at the maximum boundary.
Complete Example Code
[[See Video to Reveal this Text or Code Snippet]]
Result Interpretation
The result c is a Numpy array filled with integers that are randomly selected from the ranges specified by a and b. Each time you run the function, the output will be different due to the randomness factor, providing a variety of potential outputs.
Conclusion
In summary, generating random integers between two Numpy arrays can be accomplished with minimal effort using Numpy’s built-in functions. This method is efficient and simplifies your codebase while allowing for the flexibility of random data generation. So next time you need to create a child array with random values drawn from two parent arrays, remember to utilize the powerful capabilities of Numpy for seamless results!
Embrace the magical functionality of Numpy and enhance your data manipulation skills today!
Видео Efficient Ways to Randomly Interpolate Between Two Numpy Arrays канала vlogize
Random interpolation between 2 numpy arrays, python, numpy
Показать
Комментарии отсутствуют
Информация о видео
15 ч. 3 мин. назад
00:01:48
Другие видео канала


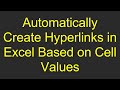
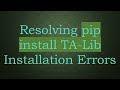
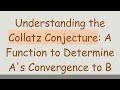


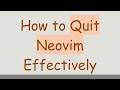

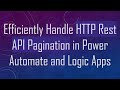
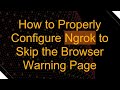


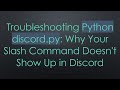

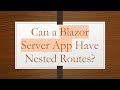
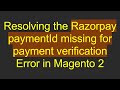
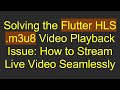
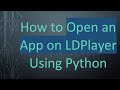

